FA2.3.2 Geometric Mean The geometric mean of a set of positive numbers x₁ through x is defined as the nth root of the product of the numbers: (eq) geometric mean =... Write a MATLAB program that will accept arbitrary number of positive input values and calculate the arithmetic mean (i.e., the avarage) and the geometric mean of the numbers. Use a while loop to get the input values, and terminate the inputs when a user enters a negative number. Test your program by calculating the average and geometric mean of the four numbers 10, 5, 2, and 5. Script 1 % Define variables: 2% X Input values Geometric mean 4 % nvals -- Number of input values 5 % prod Product of input values 6% Initialize product and nvals 7 1 = 1; 8 prod=1; -- -- 9 Sum=0 10 nvals=0; 11 % Simulate User Input 12 % Read the first number 13 sampleData = [10 5 2 5 -1]; 14 x = sampleData(1,1); 15 disp(strcat('Enter first number: ',int2str(x))); 16 % Read the remaining numbers 17 while sampleData(1,1)>0 18 prod=prod*x; %calculate product 19 20 21 22 23 i=i+1; %incrementing the count 24 25 x=sampleData(1,1); %assigning to x sum=sum+x; %calculate sum % Simulate User Input starting 2nd element of the sampleData 26 27 disp(strcat('Enter number:', int2str(x))); %displaing the values 28 end 29 % Calculate geometric mean 30 g-power (prod, 1/(1-1)); 31 fprintf('The geometric mean is %.4f \n',g) ; %displaying geometric mean |32|am-sum/(1-1); %calculating arithmetic mean 33 fprintf('The geometric mean is %.4f \n', am) ; %displaying arithmetic mean 34 % Tell user 35 fprintf('The geometric mean is %.4f\n',g); 36 My Solutions > Save C Reset MATLAB Documentation Run Script
FA2.3.2 Geometric Mean The geometric mean of a set of positive numbers x₁ through x is defined as the nth root of the product of the numbers: (eq) geometric mean =... Write a MATLAB program that will accept arbitrary number of positive input values and calculate the arithmetic mean (i.e., the avarage) and the geometric mean of the numbers. Use a while loop to get the input values, and terminate the inputs when a user enters a negative number. Test your program by calculating the average and geometric mean of the four numbers 10, 5, 2, and 5. Script 1 % Define variables: 2% X Input values Geometric mean 4 % nvals -- Number of input values 5 % prod Product of input values 6% Initialize product and nvals 7 1 = 1; 8 prod=1; -- -- 9 Sum=0 10 nvals=0; 11 % Simulate User Input 12 % Read the first number 13 sampleData = [10 5 2 5 -1]; 14 x = sampleData(1,1); 15 disp(strcat('Enter first number: ',int2str(x))); 16 % Read the remaining numbers 17 while sampleData(1,1)>0 18 prod=prod*x; %calculate product 19 20 21 22 23 i=i+1; %incrementing the count 24 25 x=sampleData(1,1); %assigning to x sum=sum+x; %calculate sum % Simulate User Input starting 2nd element of the sampleData 26 27 disp(strcat('Enter number:', int2str(x))); %displaing the values 28 end 29 % Calculate geometric mean 30 g-power (prod, 1/(1-1)); 31 fprintf('The geometric mean is %.4f \n',g) ; %displaying geometric mean |32|am-sum/(1-1); %calculating arithmetic mean 33 fprintf('The geometric mean is %.4f \n', am) ; %displaying arithmetic mean 34 % Tell user 35 fprintf('The geometric mean is %.4f\n',g); 36 My Solutions > Save C Reset MATLAB Documentation Run Script
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![FA2.3.2 Geometric Mean
The geometric mean of a set of positive numbers x₁ through x is defined as the nth root of the product of the numbers:
(eq) geometric mean =...
Write a MATLAB program that will accept arbitrary number of positive input values and calculate the arithmetic mean (i.e., the avarage) and the geometric mean of the numbers.
Use a while loop to get the input values, and terminate the inputs when a user enters a negative number. Test your program by calculating the average and geometric mean of the
four numbers 10, 5, 2, and 5.
Script
1 % Define variables:
2% X
Input values
Geometric mean
4 % nvals -- Number of input values
5 % prod Product of input values
6% Initialize product and nvals
7 1 = 1;
8 prod=1;
--
--
9 Sum=0
10 nvals=0;
11 % Simulate User Input
12 % Read the first number
13 sampleData = [10 5 2 5 -1];
14 x = sampleData(1,1);
15 disp(strcat('Enter first number: ',int2str(x)));
16 % Read the remaining numbers
17 while sampleData(1,1)>0
18
prod=prod*x; %calculate product
19
20
21
22
23 i=i+1; %incrementing the count
24
25 x=sampleData(1,1); %assigning to x
sum=sum+x; %calculate sum
% Simulate User Input starting 2nd element of the sampleData
26
27 disp(strcat('Enter number:', int2str(x))); %displaing the values
28 end
29 % Calculate geometric mean
30 g-power (prod, 1/(1-1));
31 fprintf('The geometric mean is %.4f \n',g) ; %displaying geometric mean
|32|am-sum/(1-1);
%calculating arithmetic mean
33 fprintf('The geometric mean is %.4f \n', am) ; %displaying arithmetic mean
34 % Tell user
35 fprintf('The geometric mean is %.4f\n',g);
36
My Solutions >
Save C Reset
MATLAB Documentation
Run Script](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb8058d91-61cb-4e04-a092-9a6fff19c84b%2Fe03a454b-72e4-4f23-8f74-e2a97bc4c00c%2F4mmq6l4_processed.png&w=3840&q=75)
Transcribed Image Text:FA2.3.2 Geometric Mean
The geometric mean of a set of positive numbers x₁ through x is defined as the nth root of the product of the numbers:
(eq) geometric mean =...
Write a MATLAB program that will accept arbitrary number of positive input values and calculate the arithmetic mean (i.e., the avarage) and the geometric mean of the numbers.
Use a while loop to get the input values, and terminate the inputs when a user enters a negative number. Test your program by calculating the average and geometric mean of the
four numbers 10, 5, 2, and 5.
Script
1 % Define variables:
2% X
Input values
Geometric mean
4 % nvals -- Number of input values
5 % prod Product of input values
6% Initialize product and nvals
7 1 = 1;
8 prod=1;
--
--
9 Sum=0
10 nvals=0;
11 % Simulate User Input
12 % Read the first number
13 sampleData = [10 5 2 5 -1];
14 x = sampleData(1,1);
15 disp(strcat('Enter first number: ',int2str(x)));
16 % Read the remaining numbers
17 while sampleData(1,1)>0
18
prod=prod*x; %calculate product
19
20
21
22
23 i=i+1; %incrementing the count
24
25 x=sampleData(1,1); %assigning to x
sum=sum+x; %calculate sum
% Simulate User Input starting 2nd element of the sampleData
26
27 disp(strcat('Enter number:', int2str(x))); %displaing the values
28 end
29 % Calculate geometric mean
30 g-power (prod, 1/(1-1));
31 fprintf('The geometric mean is %.4f \n',g) ; %displaying geometric mean
|32|am-sum/(1-1);
%calculating arithmetic mean
33 fprintf('The geometric mean is %.4f \n', am) ; %displaying arithmetic mean
34 % Tell user
35 fprintf('The geometric mean is %.4f\n',g);
36
My Solutions >
Save C Reset
MATLAB Documentation
Run Script
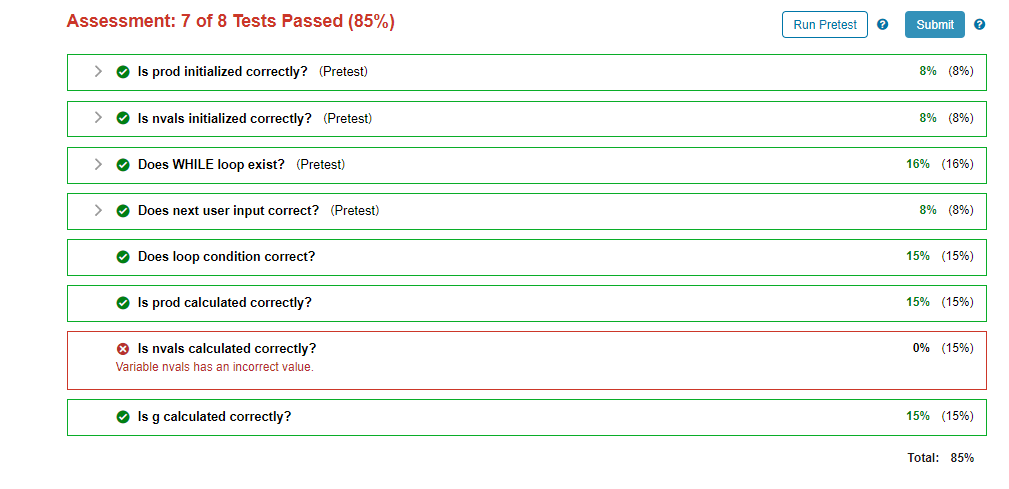
Transcribed Image Text:Assessment: 7 of 8 Tests Passed (85%)
> > Is prod initialized correctly? (Pretest)
> ✓ Is nvals initialized correctly? (Pretest)
> ✓ Does WHILE loop exist? (Pretest)
✓ Does next user input correct? (Pretest)
✔ Does loop condition correct?
Is prod calculated correctly?
Is nvals calculated correctly?
Variable nvals has an incorrect value.
✓ Is g calculated correctly?
Run Pretest
?
Submit ?
8% (8%)
8% (8%)
16% (16%)
8% (8%)
15% (15%)
15% (15%)
0% (15%)
15% (15%)
Total: 85%
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 4 images

Recommended textbooks for you
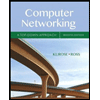
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
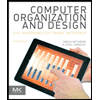
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
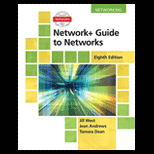
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
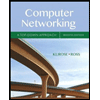
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
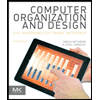
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
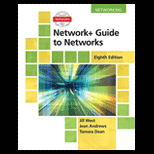
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
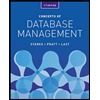
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
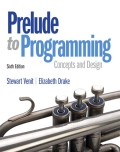
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
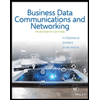
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY