Find all the errors in the C++ code below: class Point { private: int x, y; public: Point(int x = 0, int y = 0); int getX() const; // Getters int getY() const; void setX(int x); // Setters void setY(int y); }; Point::Point(int x, int y) : x(x), y(y) { } // Using initializer // Getters int Point::getX() const { return x; } int Point::getY() const { return y; } // Setters void Point::setX(int x) { this->x = x; } void Point::setY(int y) { this->y = y; } // Public Functions void print() const { cout <« "(" « x « "," « y <« ")" « endl; } // Member function overloading '+' operator void Point::operator+(Point rhs) const { return Point(x + rhs.x, y + rhs.y); } int main() { Point p1(1, 2), p2(4, 5); // Use overloaded operator + Point p3 = p1 + p2; p1.print(); // (1,2) p2.print (); // (4,5) p3.print(); // (5,7) Point p5 = p1 + p2 + p3 + p4; p5.print (); // (15,21) cout<
Find all the errors in the C++ code below: class Point { private: int x, y; public: Point(int x = 0, int y = 0); int getX() const; // Getters int getY() const; void setX(int x); // Setters void setY(int y); }; Point::Point(int x, int y) : x(x), y(y) { } // Using initializer // Getters int Point::getX() const { return x; } int Point::getY() const { return y; } // Setters void Point::setX(int x) { this->x = x; } void Point::setY(int y) { this->y = y; } // Public Functions void print() const { cout <« "(" « x « "," « y <« ")" « endl; } // Member function overloading '+' operator void Point::operator+(Point rhs) const { return Point(x + rhs.x, y + rhs.y); } int main() { Point p1(1, 2), p2(4, 5); // Use overloaded operator + Point p3 = p1 + p2; p1.print(); // (1,2) p2.print (); // (4,5) p3.print(); // (5,7) Point p5 = p1 + p2 + p3 + p4; p5.print (); // (15,21) cout<
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Note solve within 30 minutes.
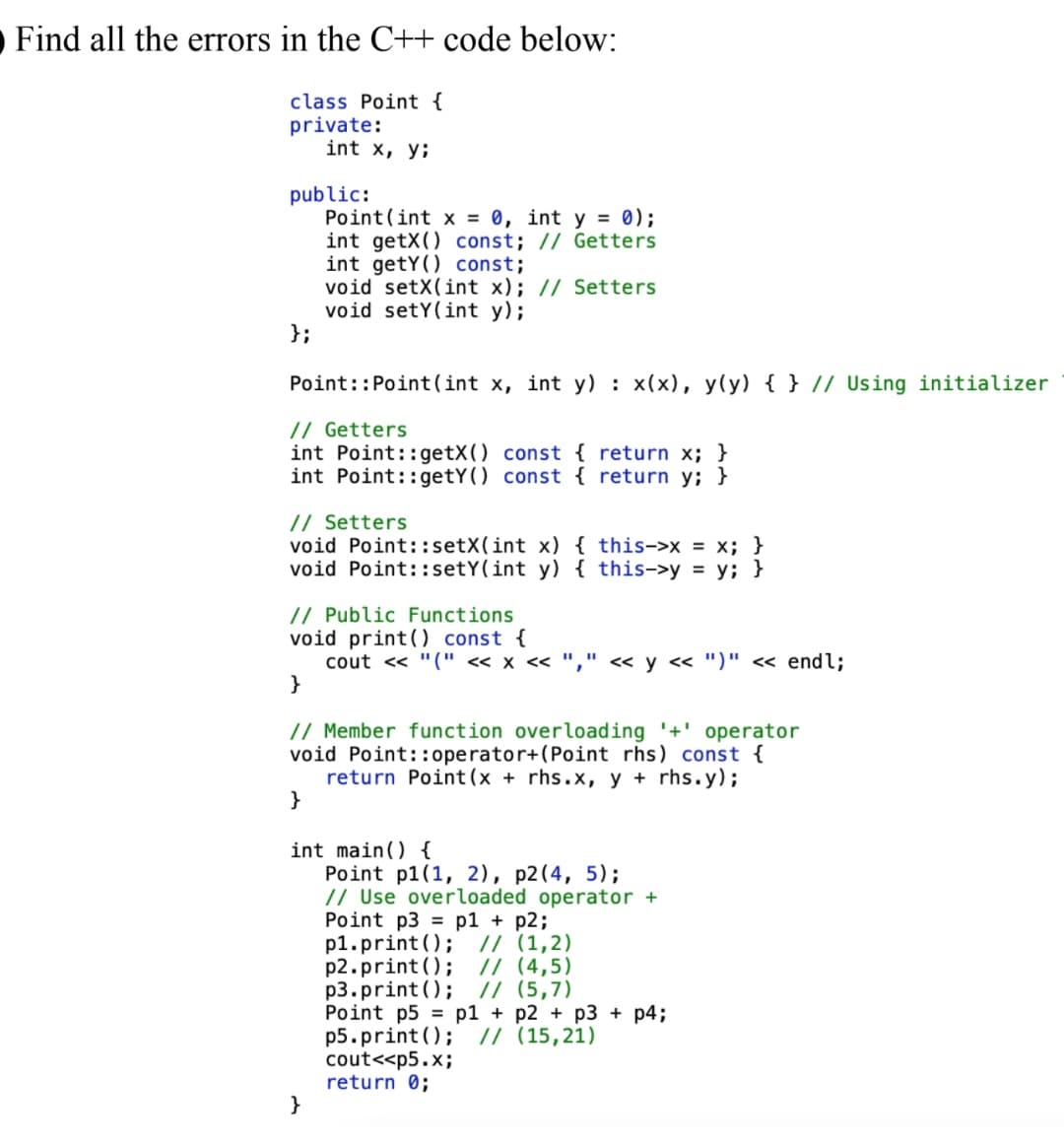
Transcribed Image Text:Find all the errors in the C++ code below:
class Point {
private:
int x, y;
public:
Point(int x = 0, int y = 0);
int getX() const; // Getters
int getY() const;
void setX(int x); // Setters
void setY(int y);
};
Point::Point(int x, int y) : x(x), y(y) { } // Using initializer
// Getters
int Point:: getX() const { return x; }
int Point::getY() const { return y; }
// Setters
void Point::setX(int x) { this->x = x; }
void Point::setY(int y) { this->y = y; }
// Public Functions
void print() const {
cout << "(" « x <« "," « y <« ")" « endl;
}
// Member function overloading '+' operator
void Point::operator+(Point rhs) const {
return Point(x + rhs.x, y + rhs.y);
}
int main() {
Point p1(1, 2), p2(4, 5);
// Use overloaded operator +
Point p3 = p1 + p2;
p1.print(); // (1,2)
p2.print (); // (4,5)
p3.print (); // (5,7)
Point p5 = p1 + p2 + p3 + p4;
p5.print (); // (15,21)
cout<<p5.x;
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
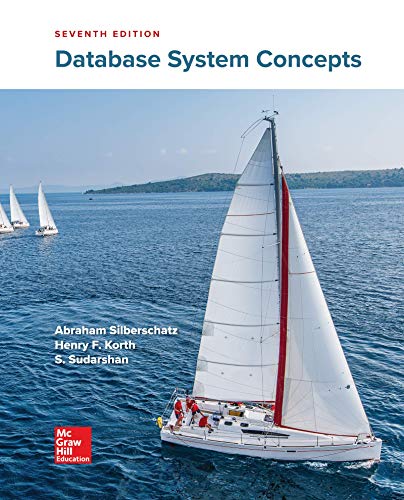
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
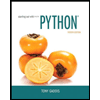
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
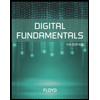
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
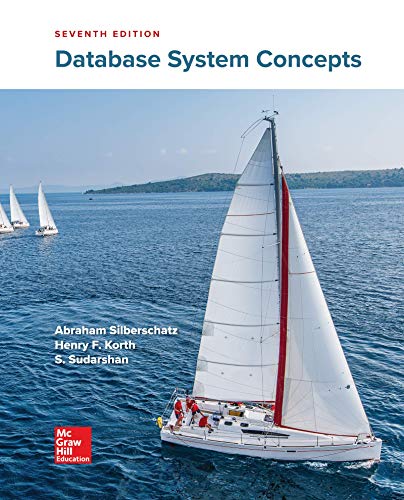
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
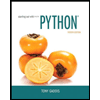
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
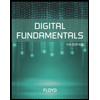
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
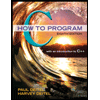
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
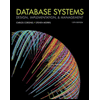
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
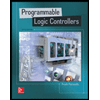
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education