Fix the errors in the Customer class and the Program. DATA FILE ThinkAbout Tech 344 Park Ave, NY, NY 10022 Kemp Technologies 3601 Broadway, NY, NY 10021 Verizon 6 Hudson Street, NY, NY 10001 Merrill Lynch 7 World Financial Center, NY, NY 10001 Philips Laboratories 345 Scarborough Rd, Briarcliff Manor, NY 10510 CODE #include #include #include using namespace std; class Customer { // Constructor void Customer(string name, string address) : cust_name(name), cust_address(address) { acct_number = this.getNextAcctNumber(); } // Accessor to get the account number const int getAcctNumber() { return acct_number; } // Accessor to get the customer name string getCustName(} const { return cust_name; } // Accessor to get the customer address string getCustAddress() const { return cust_address; } // Set a customer name and address static void set(string name, string address); // Set a customer address void setAddress(string cust_address) { cust_address = cust_address; } // Get the next account number for the next customer. static const unsigned long getNextAcctNumber() { ++nextAcctNum; } // input operator friend Customer operator>> (istream& ins, Customer cust); // output operator friend void operator<< (const ostream& outs, const Customer &cust) const; private string cust_name; // customer name unsigned long acct_number; // account number string cust_address; // customer address static const unsigned long nextAcctNum; }; const int MAX_CUSTOMERS=20; // Change this to a smaller number to test. // Declare the class variable nextAcctNum unsigned long Customer::nextAcctNum=10000; // set the customer name and address // @param name: the customer name // @param address: the account address void Customer::set(string name, string address) : cust_name(name), cust_address(address) { } // input operator reads customer as a name and address on two separate lines. // name // address1 friend Customer Customer::operator>>(istream& ins, Customer cust) { getline(cin, cust.cust_name); getline(cin, cust.cust_address); return *this; } // output operator friend void Customer::operator<<(const ostream& out, const Customer& cust) const { out << acct_number << ": " << cust_name << "\n" << cust_address << endl; } int main() { Customer custList[MAX_CUSTOMERS]; ifstream infile; string filename; cout << "Enter the name of the file for input: "; cin >> filename; infile.open(filename); if (infile.fail()) { cout << "Error: failed to open file: " << filename; exit(1); } // Read in customer list. int size = 0; while (infile >> custList[size]) { if (++size == MAX_CUSTOMERS) break; } // Get customer address changes for (int i = 0; i < MAX_CUSTOMERS; i++) { int answer; cout << "Address change for " << custList.acct_number[i] << "? (y or n) : "; cin >> answer; if (answer == 'y') { cin.ignore(); // newline getline(cin, input); custList.setAddress[i] = input; } } // Write out customer list. for (int i = 0; I < MAX_CUSTOMERS; i++) { cout << custList[i]; } return 0; }
Fix the errors in the Customer class and the Program.
DATA FILE
ThinkAbout Tech
344 Park Ave, NY, NY 10022
Kemp Technologies
3601 Broadway, NY, NY 10021
Verizon 6 Hudson Street, NY, NY 10001
Merrill Lynch
7 World Financial Center, NY, NY 10001
Philips Laboratories
345 Scarborough Rd, Briarcliff Manor, NY 10510
CODE
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Customer {
// Constructor
void Customer(string name, string address) : cust_name(name), cust_address(address)
{
acct_number = this.getNextAcctNumber();
}
// Accessor to get the account number
const int getAcctNumber() { return acct_number; }
// Accessor to get the customer name
string getCustName(} const { return cust_name; }
// Accessor to get the customer address
string getCustAddress() const { return cust_address; }
// Set a customer name and address
static void set(string name, string address);
// Set a customer address
void setAddress(string cust_address) { cust_address = cust_address; }
// Get the next account number for the next customer.
static const unsigned long getNextAcctNumber() { ++nextAcctNum; }
// input operator
friend Customer operator>> (istream& ins, Customer cust);
// output operator
friend void operator<< (const ostream& outs, const Customer &cust) const;
private
string cust_name; // customer name
unsigned long acct_number; // account number
string cust_address; // customer address
static const unsigned long nextAcctNum;
};
const int MAX_CUSTOMERS=20; // Change this to a smaller number to test.
// Declare the class variable nextAcctNum
unsigned long Customer::nextAcctNum=10000;
// set the customer name and address
// @param name: the customer name
// @param address: the account address
void Customer::set(string name, string address) : cust_name(name), cust_address(address)
{
}
// input operator reads customer as a name and address on two separate lines.
// name
// address1
friend Customer Customer::operator>>(istream& ins, Customer cust)
{
getline(cin, cust.cust_name);
getline(cin, cust.cust_address);
return *this;
}
// output operator
friend void Customer::operator<<(const ostream& out, const Customer& cust) const
{
out << acct_number << ": " << cust_name << "\n" << cust_address << endl;
}
int main()
{
Customer custList[MAX_CUSTOMERS];
ifstream infile;
string filename;
cout << "Enter the name of the file for input: ";
cin >> filename;
infile.open(filename);
if (infile.fail()) {
cout << "Error: failed to open file: " << filename;
exit(1);
}
// Read in customer list.
int size = 0;
while (infile >> custList[size]) {
if (++size == MAX_CUSTOMERS)
break;
}
// Get customer address changes
for (int i = 0; i < MAX_CUSTOMERS; i++)
{
int answer;
cout << "Address change for " << custList.acct_number[i] << "? (y or n) : ";
cin >> answer;
if (answer == 'y') {
cin.ignore(); // newline
getline(cin, input);
custList.setAddress[i] = input;
}
}
// Write out customer list.
for (int i = 0; I < MAX_CUSTOMERS; i++)
{
cout << custList[i];
}
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

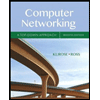
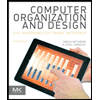
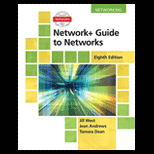
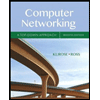
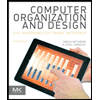
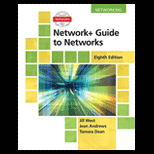
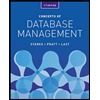
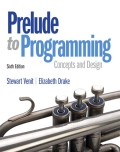
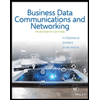