For example, the input: S 1 100.00 C 2 200.00 S 5 500.00 C 4 20.00 q 1 D 50.00 2 W 250.00 2 W 50.00 5 W 450.00 5 W 200.00 2 D 50.00 2 D 100.00 4 W 10.00 -1 must generate the following output: Error: withdrawal from account No.2 not allowed Error: withdrawal from account No.5 not allowed Error: deposit into account No.2 not allowed Savings Account Number: 1 Balance = 150.0 Credit Account Number: 2 Balance = 0.0 limit = 200.0 Savings Account Number: 5 Balance = 50.0v Credit Account Number: 4 Balance = 10.0 limit = 20.0v import java.util.ArrayList; import java.util.Scanner; public class LabProgram { public static int find(ArrayList accounts, int acctNo) { for (int i = 0; i < accounts.size(); i++) if (accounts.get(i).getAcctNo() == acctNo) return (i); return (-1); } public static void display(ArrayList accounts) { /* Displays account information for eaach account in accounts */ /* Type your code here */ } public static void transactions(ArrayList accounts, Scanner kb) { int acctNo = kb.nextInt(); double amount; String type; int i; while (acctNo >= 0) { type = kb.next(); amount = kb.nextDouble(); i = find(accounts, acctNo); if (i >= 0) { /* target account is found at position i of accounts */ /* transaction types: D for deposit and W for Withdraw */ /* Assume tranasaction codes are correct */ /* Type your code here */ } else System.out.println("Eror: account not found"); acctNo = kb.nextInt(); } } public static void main(String[] args) { ArrayList accounts = new ArrayList(); Scanner kb = new Scanner(System.in); double amount; int acctNo; String acctType = kb.next(); while (!acctType.equals("q")) { acctNo = kb.nextInt(); amount = kb.nextDouble(); if (acctType.equals("S")) accounts.add(new SavingsAccount(acctNo, amount)); else accounts.add(new CreditAccount(acctNo, amount)); acctType = kb.next(); } transactions(accounts, kb); display(accounts); } } public interface Account { public boolean deposit(double amount); public boolean withdraw(double amount); public void display(); public double getBalance(); public int getAcctNo(); } public class CreditAccount implements Account { private double creditLimit; private double balance; private int acctNo; public CreditAccount(int acctNo, double amount){ /* CreditAccount Balance. new caredit accounts */ /* start with a balance of 0" */ /* Type your code here */ } @Override public boolean withdraw(double amount){ /* Withdraws amount from the credit accout (i.e. balance=balance+amount) */ /* A withdraw that is more than credit limit is not accepted*/ /* returns true if withdrawal is accepted; otherwise retuen false */ /* Type your code here */ } @Override public boolean deposit(double amount){ /* Deposits amount into the credit account (i.e. balance=balance-amount) */ /* A deposit that is more than balance is not accepted */ /* returns true if deposit is successful; otherwise retuen false */ /* Type your code here */ } @Override public double getBalance() { /* returns account balance */ /* Type your code here */ } @Override public int getAcctNo(){ /* returns account number */ /* Type your code here */ } @Override public void display(){ System.out.println("Credit Account Number: " + acctNo + " Balance = " + balance + " limit = " + creditLimit); } } public class SavingsAccount implements Account { private int acctNo; private double balance; public SavingsAccount(int acctNo, double amount) { /* SavingsAccount Balance. */ /* Type your code here */ } @Override public boolean withdraw(double amount) { /* Withdraws amount from the savings accout (i.e. balance=balance-amount) */ /* A withdraw that is more than balance is not accepted*/ /* returns true if withdrawal is accepted; otherwise retuen false */ } @Override public boolean deposit(double amount) { /* Deposits amount into the savings account (i.e. balance=balance+amount) */ /* returns true if deposit is successful; otherwise retuen false */ /* Type your code here */ } @Override public double getBalance() { /* returns account balance */ /* Type your code here */ } @Override public int getAcctNo() { /* returns account number */ /* Type your code here */ } @Override public void display() { System.out.println("Savings Account Number: " + acctNo + " Balance = " + balance); } }
For example, the input:
S 1 100.00
C 2 200.00
S 5 500.00
C 4 20.00
q
1 D 50.00
2 W 250.00
2 W 50.00
5 W 450.00
5 W 200.00
2 D 50.00
2 D 100.00
4 W 10.00
-1
must generate the following output:
Error: withdrawal from account No.2 not allowed
Error: withdrawal from account No.5 not allowed
Error: deposit into account No.2 not allowed
Savings Account Number: 1 Balance = 150.0
Credit Account Number: 2 Balance = 0.0 limit = 200.0
Savings Account Number: 5 Balance = 50.0v Credit Account Number: 4 Balance = 10.0 limit = 20.0v
import java.util.ArrayList;
import java.util.Scanner;
public class LabProgram {
public static int find(ArrayList<Account> accounts, int acctNo) {
for (int i = 0; i < accounts.size(); i++)
if (accounts.get(i).getAcctNo() == acctNo)
return (i);
return (-1);
}
public static void display(ArrayList<Account> accounts) {
/* Displays account information for eaach account in accounts */
/* Type your code here */
}
public static void transactions(ArrayList<Account> accounts, Scanner kb) {
int acctNo = kb.nextInt();
double amount;
String type;
int i;
while (acctNo >= 0) {
type = kb.next();
amount = kb.nextDouble();
i = find(accounts, acctNo);
if (i >= 0) {
/* target account is found at position i of accounts */
/* transaction types: D for deposit and W for Withdraw */
/* Assume tranasaction codes are correct */
/* Type your code here */
}
else
System.out.println("Eror: account not found");
acctNo = kb.nextInt();
}
}
public static void main(String[] args) {
ArrayList<Account> accounts = new ArrayList<Account>();
Scanner kb = new Scanner(System.in);
double amount;
int acctNo;
String acctType = kb.next();
while (!acctType.equals("q")) {
acctNo = kb.nextInt();
amount = kb.nextDouble();
if (acctType.equals("S"))
accounts.add(new SavingsAccount(acctNo, amount));
else
accounts.add(new CreditAccount(acctNo, amount));
acctType = kb.next();
}
transactions(accounts, kb);
display(accounts);
}
}
public interface Account {
public boolean deposit(double amount);
public boolean withdraw(double amount);
public void display();
public double getBalance();
public int getAcctNo();
}
public class CreditAccount implements Account {
private double creditLimit;
private double balance;
private int acctNo;
public CreditAccount(int acctNo, double amount){
/* CreditAccount Balance. new caredit accounts */
/* start with a balance of 0" */
/* Type your code here */
}
@Override
public boolean withdraw(double amount){
/* Withdraws amount from the credit accout (i.e. balance=balance+amount) */
/* A withdraw that is more than credit limit is not accepted*/
/* returns true if withdrawal is accepted; otherwise retuen false */
/* Type your code here */
}
@Override
public boolean deposit(double amount){
/* Deposits amount into the credit account (i.e. balance=balance-amount) */
/* A deposit that is more than balance is not accepted */
/* returns true if deposit is successful; otherwise retuen false */
/* Type your code here */
}
@Override
public double getBalance() {
/* returns account balance */
/* Type your code here */
}
@Override
public int getAcctNo(){
/* returns account number */
/* Type your code here */
}
@Override
public void display(){
System.out.println("Credit Account Number: " + acctNo + " Balance = "
+ balance + " limit = " + creditLimit);
}
}
public class SavingsAccount implements Account {
private int acctNo;
private double balance;
public SavingsAccount(int acctNo, double amount) {
/* SavingsAccount Balance. */
/* Type your code here */
}
@Override
public boolean withdraw(double amount) {
/* Withdraws amount from the savings accout (i.e. balance=balance-amount) */
/* A withdraw that is more than balance is not accepted*/
/* returns true if withdrawal is accepted; otherwise retuen false */
}
@Override
public boolean deposit(double amount) {
/* Deposits amount into the savings account (i.e. balance=balance+amount) */
/* returns true if deposit is successful; otherwise retuen false */
/* Type your code here */
}
@Override
public double getBalance() {
/* returns account balance */
/* Type your code here */
}
@Override
public int getAcctNo() {
/* returns account number */
/* Type your code here */
}
@Override
public void display() {
System.out.println("Savings Account Number: " + acctNo
+ " Balance = " + balance);
}
}



Step by step
Solved in 6 steps with 5 images

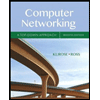
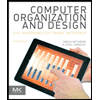
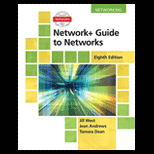
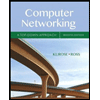
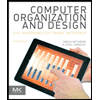
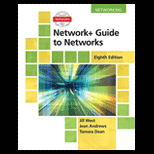
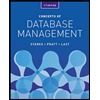
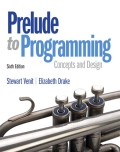
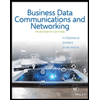