For this assignment, write a program named PizzaPrices that prompts the user to make a choice for a pizza size - S,M, L, or X - and the number of pizza the user wants to order. The price for different size pizzas are $6.99, $8.99, $12.5, and $15.0 respectively. There is also a discount based on the number of pizza ordered: no discount for one pizza, 10% discount for 2 pizzas, 15% discount for 3-5 pizzas, and 20% for more than 5 pizzas. Display a full accounting of the transaction, similar to the sample screenshot. Please make sure you use parallel arrays and range match in your solution.
For this assignment, write a program named PizzaPrices that prompts the user to make a choice for a pizza size - S,M, L, or X - and the number of pizza the user wants to order. The price for different size pizzas are $6.99, $8.99, $12.5, and $15.0 respectively. There is also a discount based on the number of pizza ordered: no discount for one pizza, 10% discount for 2 pizzas, 15% discount for 3-5 pizzas, and 20% for more than 5 pizzas. Display a full accounting of the transaction, similar to the sample screenshot. Please make sure you use parallel arrays and range match in your solution.
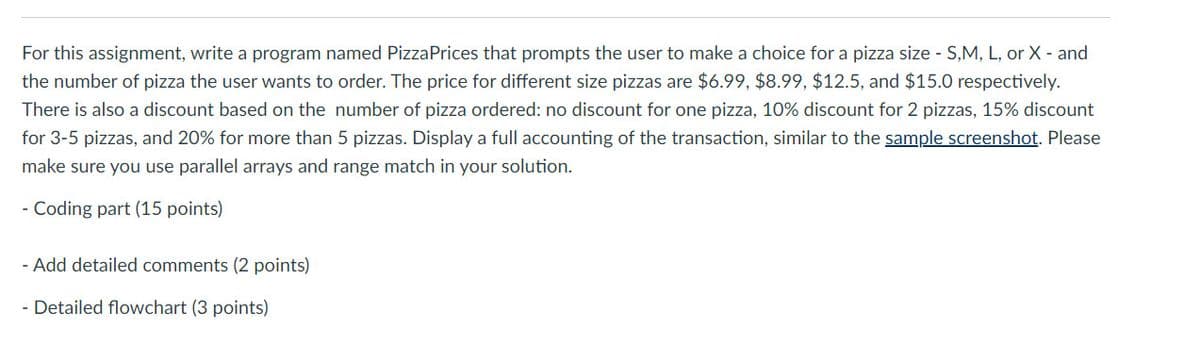
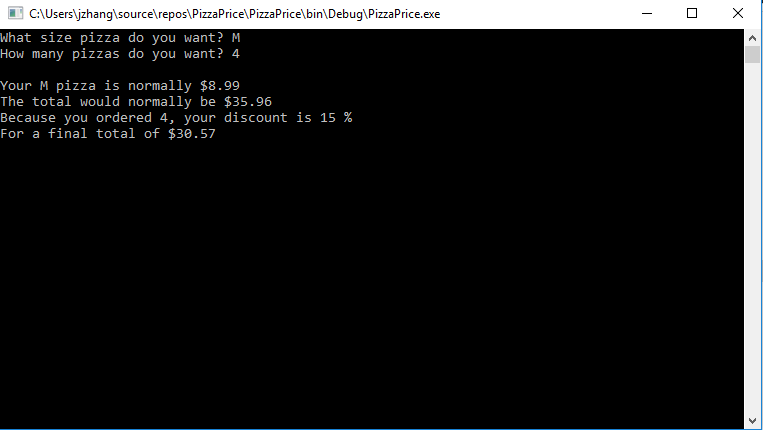

c# program for Pizza Price
using System;
class MainClass {
public static void Main (string[] args) {
int numPizza,discount;
char size;
double totalAmount=0,discountedAmount=0;
double[] rates={6.99,8.99,12.5,15.0};
Console.WriteLine ("Enter Number of Pizza's you wish to order: ");
numPizza=Convert.ToInt32(Console.ReadLine());
Console.WriteLine ("Enter Size (S,M,L,X): ");
size=Console.ReadLine()[0];
if(size=='S' || size=='s'){
totalAmount=numPizza*rates[0];
}
else if(size=='M' || size=='m'){
totalAmount=numPizza*rates[1];
}
else if(size=='L' || size=='l'){
totalAmount=numPizza*rates[2];
}
else if(size=='X' || size=='x'){
totalAmount=numPizza*rates[3];
}
else
Console.WriteLine("Wrong size input");
if(numPizza==1){
discountedAmount=totalAmount;
discount=0;
}
else if(numPizza==2){
discountedAmount=totalAmount-(totalAmount*0.10);
discount=10;
}
else if(numPizza>2 && numPizza<=5){
discountedAmount=totalAmount-(totalAmount*0.15);
discount=15;
}
else{
discountedAmount=totalAmount-(totalAmount*0.20);
discount=20;
}
Console.WriteLine("Total number of Pizza: "+numPizza);
Console.WriteLine("Size: "+size);
Console.WriteLine("Total Amount= $"+totalAmount);
Console.WriteLine("Total discount = "+discount+"%");
Console.WriteLine("Total Amount after discount = $"+discountedAmount);
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

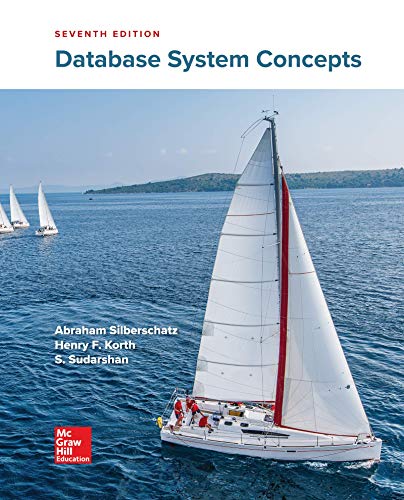
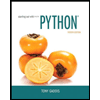
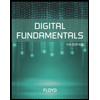
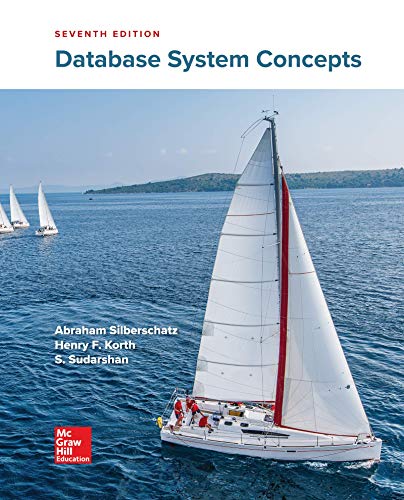
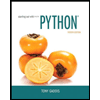
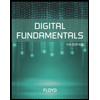
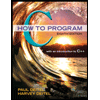
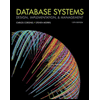
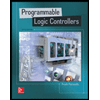