For this assignment you will be building on the Fraction class you began last week. All the requirements from that class are still in force. You'll be making five major changes to the class. Delete your set() function. Add two constructors, a default constructor (a constructor that takes no parameters) and a parameterized constructor (a constructor that takes parameters). The default constructor assigns the value 0 to the Fraction. In the parameterized constructor, the first parameter will represent the initial numerator of the Fraction, and the second parameter will represent the initial denominator of the Fraction. Since Fractions cannot have denominators of 0, the default constructor should assign 0 to the numerator and 1 to the denominator. Also, the parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement "assert(denominatorParameter != 0);". To use the assert() function you'll also need to #include . (Note, I don't expect the variable to be named "denominatorParameter," that's just my placeholder for the example.) assert() is not the best way to handle this, but it will have to do until we study exception handling. Add the const keyword to your class wherever appropriate (and also make sure to pass objects by reference -- see lesson 15.9). Your class may still work correctly even if you don't do this correctly, so this will require extra care!! Add a private "simplify()" function to your class and call it from the appropriate member functions. The best way to do this is to make the function a void function with no parameters that reduces the calling object. I am providing a client program for you below. You should copy and paste this and use it as your client program. The output that should be produced when the provided client program is run with your class is also given below, so that you can check your results. Since you are not writing the client program, you are not required to include comments in it. Here is the client program. #include #include "Fraction.h" using namespace std; int main() { Fraction f1(9,8); Fraction f2(2,3); Fraction result; cout << "The result starts off at "; result.print(); cout << endl; cout << "The product of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.multipliedBy(f2); result.print(); cout << endl; cout << "The quotient of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.dividedBy(f2); result.print(); cout << endl; cout << "The sum of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.addedTo(f2); result.print(); cout << endl; cout << "The difference of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.subtract(f2); result.print(); cout << endl; if (f1.isEqualTo(f2)){ cout << "The two Fractions are equal." << endl; } else { cout << "The two Fractions are not equal." << endl; } const Fraction f3(12, 8); const Fraction f4(202, 303); result = f3.multipliedBy(f4); cout << "The product of "; f3.print(); cout << " and "; f4.print(); cout << " is "; result.print(); cout << endl; }
For this assignment you will be building on the Fraction class you began last week. All the requirements from that class are still in force. You'll be making five major changes to the class.
-
Delete your set() function. Add two constructors, a default constructor (a constructor that takes no parameters) and a parameterized constructor (a constructor that takes parameters). The default constructor assigns the value 0 to the Fraction. In the parameterized constructor, the first parameter will represent the initial numerator of the Fraction, and the second parameter will represent the initial denominator of the Fraction.
Since Fractions cannot have denominators of 0, the default constructor should assign 0 to the numerator and 1 to the denominator. Also, the parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement "assert(denominatorParameter != 0);". To use the assert() function you'll also need to #include <cassert>. (Note, I don't expect the variable to be named "denominatorParameter," that's just my placeholder for the example.)
assert() is not the best way to handle this, but it will have to do until we study exception handling.
-
Add the const keyword to your class wherever appropriate (and also make sure to pass objects by reference -- see lesson 15.9). Your class may still work correctly even if you don't do this correctly, so this will require extra care!!
-
Add a private "simplify()" function to your class and call it from the appropriate member functions. The best way to do this is to make the function a void function with no parameters that reduces the calling object.
I am providing a client
program for you below. You should copy and paste this and use it as your client program. The output that should be produced when the provided client program is run with your class is also given below, so that you can check your results. Since you are not writing the client program, you are not required to include comments in it.Here is the client program.
#include <iostream> #include "Fraction.h" using namespace std; int main() { Fraction f1(9,8); Fraction f2(2,3); Fraction result; cout << "The result starts off at "; result.print(); cout << endl; cout << "The product of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.multipliedBy(f2); result.print(); cout << endl; cout << "The quotient of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.dividedBy(f2); result.print(); cout << endl; cout << "The sum of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.addedTo(f2); result.print(); cout << endl; cout << "The difference of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.subtract(f2); result.print(); cout << endl; if (f1.isEqualTo(f2)){ cout << "The two Fractions are equal." << endl; } else { cout << "The two Fractions are not equal." << endl; } const Fraction f3(12, 8); const Fraction f4(202, 303); result = f3.multipliedBy(f4); cout << "The product of "; f3.print(); cout << " and "; f4.print(); cout << " is "; result.print(); cout << endl; }
This client should produce the output shown here:
The result starts off at 0/1 The product of 9/8 and 2/3 is 3/4 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/24 The difference of 9/8 and 2/3 is 11/24 The two Fractions are not equal. The product of 3/2 and 2/3 is 1/1

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

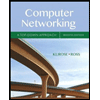
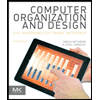
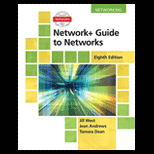
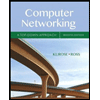
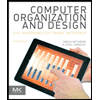
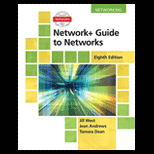
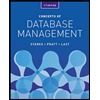
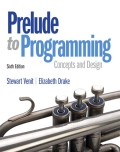
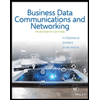