For this assignment you will be building on the Fraction class you began last week. All the requirements from that class are still in force. You'll be making five major changes to the class. Delete your set() function. Add two constructors, a default constructor (a constructor that takes no parameters) and a parameterized constructor (a constructor that takes parameters). The default constructor assigns the value 0 to the Fraction. In the parameterized constructor, the first parameter will represent the initial numerator of the Fraction, and the second parameter will represent the initial denominator of the Fraction. Since Fractions cannot have denominators of 0, the default constructor should assign 0 to the numerator and 1 to the denominator. Also, the parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement "assert(denominatorParameter != 0);". To use the assert() function you'll also need to #include . assert() is not the best way to handle this, but it will have to do until we study exception handling. Add the const keyword to your class wherever appropriate (and also make sure to pass objects by reference -- see lesson 15.9). Your class may still work correctly even if you don't do this correctly, so this will require extra care!! Add a private "simplify()" function to your class and call it from the appropriate member functions. The best way to do this is to make the function a void function with no parameters that reduces the calling object. As you can see from the sample output given below, you are still not required to change improper Fractions into mixed numbers for printing. Just print it as an improper Fraction. Make sure that your class will reduce ANY non-negative Fraction, not just the Fractions that are tested in the provided client program. Fractions should not be simply reduced upon output, they should be stored in reduced form at all times. In other words, you should ensure that all Fraction objects are reduced before the end of any member function. You are also not required to deal with negative numbers, either in the numerator or the denominator. You must create your own algorithm for reducing Fractions. Don't look up an already existing algorithm for reducing Fractions or finding GCF. The point here is to have you practice solving the problem on your own. In particular, don't use Euclid's algorithm. Don't worry about being efficient. It's fine to have your function check every possible factor, even if it would be more efficient to just check prime numbers. Just create something of your own that works correctly on ANY Fraction. Note: this part of the assignment is worth 5 points. If you are having trouble keeping up with the class, I suggest you skip this part and take the 5 point deduction. Put the client program in a separate file from the class, and divide the class into specification file (fraction.h) and implementation file (fraction.cpp), so your code will be in 3 separate files. Your class should still have exactly two data members.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
For this assignment you will be building on the Fraction class you began last week. All the requirements from that class are still in force. You'll be making five major changes to the class.
- Delete your set() function. Add two constructors, a default constructor (a constructor that takes no parameters) and a parameterized constructor (a constructor that takes parameters). The default constructor assigns the value 0 to the Fraction. In the parameterized constructor, the first parameter will represent the initial numerator of the Fraction, and the second parameter will represent the initial denominator of the Fraction.
Since Fractions cannot have denominators of 0, the default constructor should assign 0 to the numerator and 1 to the denominator. Also, the parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement "assert(denominatorParameter != 0);". To use the assert() function you'll also need to #include <cassert>.
assert() is not the best way to handle this, but it will have to do until we study exception handling. - Add the const keyword to your class wherever appropriate (and also make sure to pass objects by reference -- see lesson 15.9). Your class may still work correctly even if you don't do this correctly, so this will require extra care!!
- Add a private "simplify()" function to your class and call it from the appropriate member functions. The best way to do this is to make the function a void function with no parameters that reduces the calling object.
As you can see from the sample output given below, you are still not required to change improper Fractions into mixed numbers for printing. Just print it as an improper Fraction. Make sure that your class will reduce ANY non-negative Fraction, not just the Fractions that are tested in the provided client program. Fractions should not be simply reduced upon output, they should be stored in reduced form at all times. In other words, you should ensure that all Fraction objects are reduced before the end of any member function. You are also not required to deal with negative numbers, either in the numerator or the denominator.
You must create your ownalgorithm for reducing Fractions. Don't look up an already existing algorithm for reducing Fractions or finding GCF. The point here is to have you practice solving the problem on your own. In particular, don't use Euclid's algorithm. Don't worry about being efficient. It's fine to have your function check every possible factor, even if it would be more efficient to just check prime numbers. Just create something of your own that works correctly on ANY Fraction.
Note: this part of the assignment is worth 5 points. If you are having trouble keeping up with the class, I suggest you skip this part and take the 5 point deduction. - Put the client program in a separate file from the class, and divide the class into specification file (fraction.h) and implementation file (fraction.cpp), so your code will be in 3 separate files.
Your class should still have exactly two data members.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

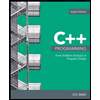
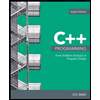