For this challenge, you will write a program that prompts a user to answer whether or not they are feeling stressed, and if so, recommend them a quick activity to try. Then, the program will continue to prompt the user to answer whether or not they are still feeling stressed, giving them the options "Y" or "N", and recommend a new activity each time until the user responds no, or "N". We have given you the following code to get started: • CommWell Health Blog recommends 12 natural ways to reduce stress, which we have copied into a list of strings called wellness activities. • Completed the print statement for you which uses the random.choice () method from the random module to choose a random string from the list wellness activities (Note: Your first subtask is to import the random python module, else this line of code will not work) Complete the program as follows: 1. Import the random python module at the top of your code (TODO #1). 2. Define a function called wellness_recommender that takes no input parameters (TODO #2). 3. Prompt the user with the following question: "Are you feeling stressed? (Y/N)", and store their answer in the variable response (TODO #3). 4. Write an infinite while loop (TODO # 4) and within the while loop: 1. Complete the if condition to check if the user entered "Y" (TODO #5) 2. Complete the elif condition to check if the user entered "N" (TODO #6) 1. If the user entered "N", print a take care message and break out of the while loop (TODO #7) 3. If the user enters any other option (e.g., "n", "y", "no", "yes"), then print the following message: "Invalid option. The only valid options are Y or N." (TODO #8) 4. Prompt the user again with the question: "Are you still feeling stressed? (Y/N)" (already done for you!) 5. Call the wellness_recommender function with the appropriate arguments (if any). ***There are no test cases for this exercise-test this program on your own by running it in the terminal and making sure it works as expected. Once you are satisfied, in order to move on to the next task you must have a tutor check off that it works as expected. Sample Runs: # Sample Run #1: Are you feeling stressed (Y/N)? Y Try to stretch Are you still feeling stressed (Y/N)? Y Try to have a laugh Are you still feeling stressed (Y/N)? N Glad that you are not feeling stressed! Take care! 1 TODO #1: Import the random python module 2 3 # Note: You can split a long line using backslashes as shown below! 4 wellness activities = ["get outside", "turn on some tunes", "take a walk", \ "stretch", "eat a smart snack", "have a laugh", \ 5 6 "wish someone well", "connect with someone", 7 "do a good deed", "clear your mind", \ 8 "check a chore off your list", "give yourself a pep ta 9 10 def # TODO #2: Complete the function header 11 response = # TODO #3: Prompt the user for "Y" or "N" 12 13 while # TODO #4: Complete the while condition to make it an infinite loop 14 if # TODO #5: Complete the if condition 15 print("Try to " + random.choice (wellness activities)) elif # TODO #6: Complete the elif condition 16 17 17 print("Glad that you are not feeling stressed! Take care!") 18 # TODO #7: Write a statement to break out of the loop 19 else: 20 20 # TODO #8: print an error message for invalid options response input ("Are you still feeling stressed (Y/N)? ") 21 22 23 # TODO #9: Call the wellness recommender function 24 /home/lab4_3.py Spaces: 4 (Auto) Console Terminal
For this challenge, you will write a program that prompts a user to answer whether or not they are feeling stressed, and if so, recommend them a quick activity to try. Then, the program will continue to prompt the user to answer whether or not they are still feeling stressed, giving them the options "Y" or "N", and recommend a new activity each time until the user responds no, or "N". We have given you the following code to get started: • CommWell Health Blog recommends 12 natural ways to reduce stress, which we have copied into a list of strings called wellness activities. • Completed the print statement for you which uses the random.choice () method from the random module to choose a random string from the list wellness activities (Note: Your first subtask is to import the random python module, else this line of code will not work) Complete the program as follows: 1. Import the random python module at the top of your code (TODO #1). 2. Define a function called wellness_recommender that takes no input parameters (TODO #2). 3. Prompt the user with the following question: "Are you feeling stressed? (Y/N)", and store their answer in the variable response (TODO #3). 4. Write an infinite while loop (TODO # 4) and within the while loop: 1. Complete the if condition to check if the user entered "Y" (TODO #5) 2. Complete the elif condition to check if the user entered "N" (TODO #6) 1. If the user entered "N", print a take care message and break out of the while loop (TODO #7) 3. If the user enters any other option (e.g., "n", "y", "no", "yes"), then print the following message: "Invalid option. The only valid options are Y or N." (TODO #8) 4. Prompt the user again with the question: "Are you still feeling stressed? (Y/N)" (already done for you!) 5. Call the wellness_recommender function with the appropriate arguments (if any). ***There are no test cases for this exercise-test this program on your own by running it in the terminal and making sure it works as expected. Once you are satisfied, in order to move on to the next task you must have a tutor check off that it works as expected. Sample Runs: # Sample Run #1: Are you feeling stressed (Y/N)? Y Try to stretch Are you still feeling stressed (Y/N)? Y Try to have a laugh Are you still feeling stressed (Y/N)? N Glad that you are not feeling stressed! Take care! 1 TODO #1: Import the random python module 2 3 # Note: You can split a long line using backslashes as shown below! 4 wellness activities = ["get outside", "turn on some tunes", "take a walk", \ "stretch", "eat a smart snack", "have a laugh", \ 5 6 "wish someone well", "connect with someone", 7 "do a good deed", "clear your mind", \ 8 "check a chore off your list", "give yourself a pep ta 9 10 def # TODO #2: Complete the function header 11 response = # TODO #3: Prompt the user for "Y" or "N" 12 13 while # TODO #4: Complete the while condition to make it an infinite loop 14 if # TODO #5: Complete the if condition 15 print("Try to " + random.choice (wellness activities)) elif # TODO #6: Complete the elif condition 16 17 17 print("Glad that you are not feeling stressed! Take care!") 18 # TODO #7: Write a statement to break out of the loop 19 else: 20 20 # TODO #8: print an error message for invalid options response input ("Are you still feeling stressed (Y/N)? ") 21 22 23 # TODO #9: Call the wellness recommender function 24 /home/lab4_3.py Spaces: 4 (Auto) Console Terminal
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter5: Control Structures Ii (repetition)
Section: Chapter Questions
Problem 19PE
Related questions
Question
Help with code
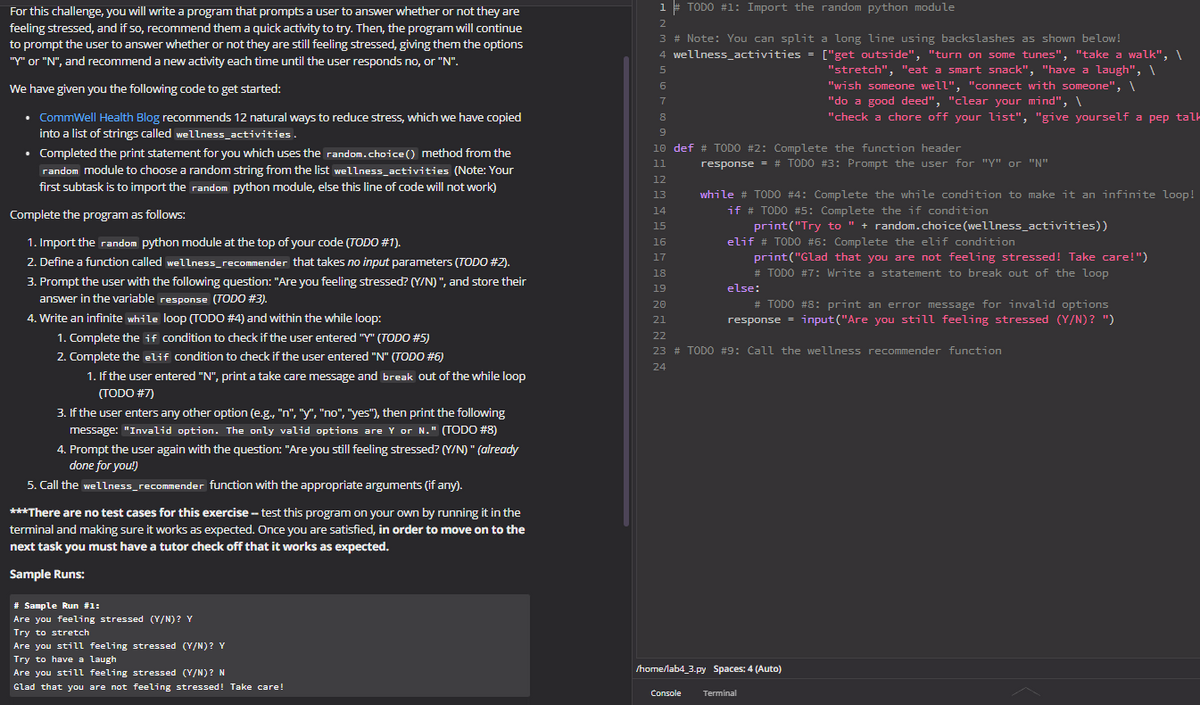
Transcribed Image Text:For this challenge, you will write a program that prompts a user to answer whether or not they are
feeling stressed, and if so, recommend them a quick activity to try. Then, the program will continue
to prompt the user to answer whether or not they are still feeling stressed, giving them the options
"Y" or "N", and recommend a new activity each time until the user responds no, or "N".
We have given you the following code to get started:
• CommWell Health Blog recommends 12 natural ways to reduce stress, which we have copied
into a list of strings called wellness_activities.
• Completed the print statement for you which uses the random.choice () method from the
random module to choose a random string from the list wellness activities (Note: Your
first subtask is to import the random python module, else this line of code will not work)
Complete the program as follows:
1. Import the random python module at the top of your code (TODO #1).
2. Define a function called wellness_recommender that takes no input parameters (TODO #2).
3. Prompt the user with the following question: "Are you feeling stressed? (Y/N)", and store their
answer in the variable response (TODO #3).
4. Write an infinite while loop (TODO #4) and within the while loop:
1. Complete the if condition to check if the user entered "Y" (TODO #5)
2. Complete the elif condition to check if the user entered "N" (TODO #6)
1. If the user entered "N", print a take care message and break out of the while loop
(TODO #7)
3. If t
user enters any other option (e.g., "n", "y", "no", "yes"), then print the following
message: "Invalid option. The only valid options are Y or N." (TODO #8)
4. Prompt the user again with the question: "Are you still feeling stressed? (Y/N)" (already
done for you!)
5. Call the wellness_recommender function with the appropriate arguments (if any).
***There are no test cases for this exercise - test this program on your own by running it in the
terminal and making sure it works as expected. Once you are satisfied, in order to move on to the
next task you must have a tutor check off that it works as expected.
Sample Runs:
# Sample Run #1:
Are you feeling stressed (Y/N)? Y
Try to stretch
Are you still feeling stressed (Y/N)? Y
Try to have a laugh
Are you still feeling stressed (Y/N)? N
Glad that you are not feeling stressed! Take care!
1 # TODO #1: Import the random python module
2
3 # Note: You can split a long line using backslashes as shown below!
4 wellness activities = ["get outside", "turn on some tunes", "take a walk", \
"stretch", "eat a smart snack", "have a laugh", \
5
6
"wish someone well", "connect with someone", \
7
"do a good deed", "clear your mind", \
8
"check a chore off your list", "give yourself a pep talk
9
10 def # TODO #2: Complete the function header
11
response = # TODO #3: Prompt the user for "Y" or "N"
12
13
while # TODO #4: Complete the while condition to make it an infinite loop!
14
if # TODO #5: Complete the if condition
15
print("Try to " + random.choice (wellness_activities))
16
elif # TODO #6: Complete the elif condition
17
print("Glad that you are not feeling stressed! Take care!")
18
# TODO #7: Write a statement to break out of the loop
19
else:
20
# TODO #8: print an error message for invalid options
response = input ("Are you still feeling stressed (Y/N)? ")
21
22
23 # TODO #9: Call the wellness recommender function
24
/home/lab4_3.py Spaces: 4 (Auto)
Console
Terminal
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
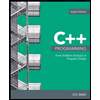
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
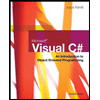
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
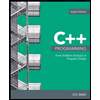
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
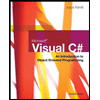
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,