from typing import List def smash_or_sad (the_list: List[int], hulk_power: int) -> int: smash_count=0 for i in range (len (the_list)): if the list[i] <= hulk_power: print("Hulk SMASH! >:(") smash_count += 1 else: print("Hulk Sad :(") return smash_count def main() -> None: my_list = [10, 14, 16] hulk power 15
from typing import List def smash_or_sad (the_list: List[int], hulk_power: int) -> int: smash_count=0 for i in range (len (the_list)): if the list[i] <= hulk_power: print("Hulk SMASH! >:(") smash_count += 1 else: print("Hulk Sad :(") return smash_count def main() -> None: my_list = [10, 14, 16] hulk power 15
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Need help faithfully translating the below Python code (ignoring type hints) into a properly commented MIPS program. Please ensure that while passing arguments to the function, you should pass 'the_list' as the first argument and 'hulk_power' as the second argument. Included is an example run of the expected output of the program as well as a MIPS sheet of allowed instructions that can be used. Thank you in advance for your help :)
![from typing import List
def smash_or_sad (the_list: List[int], hulk_power: int) -> int:
smash_count=0
for i in range (len (the_list)):
if the list[i] <= hulk_power:
print("Hulk SMASH! >:(")
smash_count += 1
else:
print("Hulk Sad :(")
return smash_count
def main() -> None:
my_list = [10, 14, 16]
hulk power
15
print (f"Hulk smashed {smash_or_sad (my_list, hulk_power)} people")
main ()
You can test your program by clicking "Run", or by running the following command in the Terminal:
An example of a run is:
Hulk SMASH! >:(
Hulk SMASH! >:(
Hulk Sad (
Hulk smashed 2 people.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3cb672f7-47ed-4ee3-be4e-71db737c6150%2F33041037-0980-4052-aeae-647c5dc581dd%2F7khen8_processed.png&w=3840&q=75)
Transcribed Image Text:from typing import List
def smash_or_sad (the_list: List[int], hulk_power: int) -> int:
smash_count=0
for i in range (len (the_list)):
if the list[i] <= hulk_power:
print("Hulk SMASH! >:(")
smash_count += 1
else:
print("Hulk Sad :(")
return smash_count
def main() -> None:
my_list = [10, 14, 16]
hulk power
15
print (f"Hulk smashed {smash_or_sad (my_list, hulk_power)} people")
main ()
You can test your program by clicking "Run", or by running the following command in the Terminal:
An example of a run is:
Hulk SMASH! >:(
Hulk SMASH! >:(
Hulk Sad (
Hulk smashed 2 people.
![Call code Service
($v0)
1
4
5
=
8
9
10
Print integer
Print string
Input integer
Input string
On function call:
Instruction format
add Rdest, Rarel, Hare2
sub Rdest, Rarel, Rare2
mult Rsrel, Rare2
div Rarel, Rsrc2
j label
jal label
and Rdest, Rsrel, Rsre2
or Rdest, Rsrel, Rare2
xor Rdest, Rarel, Rare2
nor Rdest, Rarel, Rsre2
sllv Rdest, Rarel, Rare2
srlv Rdest, Rarel, Rare2
srav Rdest, Rarel, Rarc2
jr Rare
jair Rare
syscall
Arguments
Allocate memory $a0= number of bytes
Exit
mfhi Rdest
mflo Rdest
w Rdest, Addr
sw Rsre, Addr
la Rdest, Addr(or label)**
beq Rarel, Rare2, label
bne Rarel, Rare2, label
slt Rdest, Rarel. Rsre2
$a0 value to print
$a0-address of string to print
$20 address at which the
string will be stored
$a1 = maximum number of
characters in the string
R29
R30
R31
Number
ROO
R01
R02, R03
Table 1: System calls.
R24, R25
R28
Purpose
provides constant zero
reserved for assembler
system call code, return value
R04-R07 $20--$23
system call and function arguments
R08-R15 $t0--$17 temporary storage (caller-saved)
R16-R23 $20-$s7
Sat
$v0, $v1
Table 2: General-purpose registers
Name
$zero
$18, $19
$KP
$sp
$fp
$ra
Caller:
sets $v0 to return value
clears local variables off stack
On function return: restores saved $fp off stack
restores saved $ra off stack
returns to caller with jr $ra
Subtract
Multiply
Divide
Table 3: Assembler directives
data
assemble into data segment
text
assemble into text (code) segment
word wl, w2, ...] allocate word(s) with initial value(s)
space n
ascii "string"
asciiz "string"
Returns
$v0 = entered integer
Caller:
saves temporary registers on stack
passes arguments on stack
calls function using jal falabel
$v0 address of first byte
temporary storage (callee-saved)
temporary storage (caller-saved)
pointer to global area.
Table 5: A partial instruction set is provided below. The following conventions apply.
Instruction Format column
Rare, Rarel, Rsre2: register source operand(s) - must be the name of a register
Rdest: register destination must be the name of a register
Addr: address in the form offset (Rarc), that is, absolute address Rarc offset
label: label of an instruction.
**: pseudoinstruction
stack pointer
frame pointer
return address
allocate n bytes of uninitialized, unaligned space.
allocate ASCII string, do not terminate
allocate ASCII string, terminate with "\0*
Table 4: Function calling convention
Callee:
saves value of $ra on stack
saves value of $fp on stack
Immediate Form column
Associated instruction where Rsre2 is an immediate. Symbol - appears if there is no immediate form.
Unsigned or overflow column
Associated unsigned (or overflow) instruction for the values of Rsrel and Rsre2. Symbol - if no such form.
Bitwise AND
Bitwise OR
Bitwise XOR
Bitwise NOR
Shift Left Logical
Shift Right Logical
Shift Right Arithmet
Move from Hi
Move from Lo
Load word
Store word
Load Address (for
printing strings)
Branch if equal
Branch if not equal
Set if less than
Jump
Jump and link
copies $sp to $fp
allocates local variables on stack
Table 6: Allowed MIPS instruction (and pseudoinstruction) set
Meaning
Operation
Add
Caller:
clears arguments off stack
restores temporary registers off stack
uses return value in $v0
Rdest = Rsrel + Rsrc2
Rdest=Rsrel- Rare2
Hi:Lo Rarel Rare2
Lo Rarel/Rsrc2;
Hi Rsrel % Rsrc2
Rdest Rsrel & Rsrc2
Rdest Rarel | Rsrc2
Rdest Rarel A Rare2
Rdest(Rarel | Rare2)
Rdest = Rsrel << Rsre2
Rdest = Rsrel >> Rsrc2
(MSB-0)
Rdest Rarel >> Rare2
(MSB preserved)
Rdest = Hi
Rdest Lo
Rdest meen32 Addr
mem32[Addr] Rare
Rdest=Addr
Rdest=label)
if (Rarel Rsrc2)
PC = label
if (Rarel ! Rare2)
PC label
(or
if (Rarel< Rare2)
Rdest 1
else Rdest 0
PC label
Sra PC + 4;
PC label
Jump register
PC- Rare
Jump and link register Sra = PC + 4
PC Rare
system call
depends on the value of
$VO
Immediate
addi
-
andi
ori
Notes
value is signed
string must be termi-
nated with 0
value is signed
returns if $a1-1 char
acters or Enter typed,
the string is termi-
nated with 10
ends simulation
xori
sll
srl
sra
-
slti
Unsigned or Overflow
addu (no overflow trap)
subu (no overflow trap)
divu
.
situ, sitiu](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3cb672f7-47ed-4ee3-be4e-71db737c6150%2F33041037-0980-4052-aeae-647c5dc581dd%2F7mb0ggw_processed.png&w=3840&q=75)
Transcribed Image Text:Call code Service
($v0)
1
4
5
=
8
9
10
Print integer
Print string
Input integer
Input string
On function call:
Instruction format
add Rdest, Rarel, Hare2
sub Rdest, Rarel, Rare2
mult Rsrel, Rare2
div Rarel, Rsrc2
j label
jal label
and Rdest, Rsrel, Rsre2
or Rdest, Rsrel, Rare2
xor Rdest, Rarel, Rare2
nor Rdest, Rarel, Rsre2
sllv Rdest, Rarel, Rare2
srlv Rdest, Rarel, Rare2
srav Rdest, Rarel, Rarc2
jr Rare
jair Rare
syscall
Arguments
Allocate memory $a0= number of bytes
Exit
mfhi Rdest
mflo Rdest
w Rdest, Addr
sw Rsre, Addr
la Rdest, Addr(or label)**
beq Rarel, Rare2, label
bne Rarel, Rare2, label
slt Rdest, Rarel. Rsre2
$a0 value to print
$a0-address of string to print
$20 address at which the
string will be stored
$a1 = maximum number of
characters in the string
R29
R30
R31
Number
ROO
R01
R02, R03
Table 1: System calls.
R24, R25
R28
Purpose
provides constant zero
reserved for assembler
system call code, return value
R04-R07 $20--$23
system call and function arguments
R08-R15 $t0--$17 temporary storage (caller-saved)
R16-R23 $20-$s7
Sat
$v0, $v1
Table 2: General-purpose registers
Name
$zero
$18, $19
$KP
$sp
$fp
$ra
Caller:
sets $v0 to return value
clears local variables off stack
On function return: restores saved $fp off stack
restores saved $ra off stack
returns to caller with jr $ra
Subtract
Multiply
Divide
Table 3: Assembler directives
data
assemble into data segment
text
assemble into text (code) segment
word wl, w2, ...] allocate word(s) with initial value(s)
space n
ascii "string"
asciiz "string"
Returns
$v0 = entered integer
Caller:
saves temporary registers on stack
passes arguments on stack
calls function using jal falabel
$v0 address of first byte
temporary storage (callee-saved)
temporary storage (caller-saved)
pointer to global area.
Table 5: A partial instruction set is provided below. The following conventions apply.
Instruction Format column
Rare, Rarel, Rsre2: register source operand(s) - must be the name of a register
Rdest: register destination must be the name of a register
Addr: address in the form offset (Rarc), that is, absolute address Rarc offset
label: label of an instruction.
**: pseudoinstruction
stack pointer
frame pointer
return address
allocate n bytes of uninitialized, unaligned space.
allocate ASCII string, do not terminate
allocate ASCII string, terminate with "\0*
Table 4: Function calling convention
Callee:
saves value of $ra on stack
saves value of $fp on stack
Immediate Form column
Associated instruction where Rsre2 is an immediate. Symbol - appears if there is no immediate form.
Unsigned or overflow column
Associated unsigned (or overflow) instruction for the values of Rsrel and Rsre2. Symbol - if no such form.
Bitwise AND
Bitwise OR
Bitwise XOR
Bitwise NOR
Shift Left Logical
Shift Right Logical
Shift Right Arithmet
Move from Hi
Move from Lo
Load word
Store word
Load Address (for
printing strings)
Branch if equal
Branch if not equal
Set if less than
Jump
Jump and link
copies $sp to $fp
allocates local variables on stack
Table 6: Allowed MIPS instruction (and pseudoinstruction) set
Meaning
Operation
Add
Caller:
clears arguments off stack
restores temporary registers off stack
uses return value in $v0
Rdest = Rsrel + Rsrc2
Rdest=Rsrel- Rare2
Hi:Lo Rarel Rare2
Lo Rarel/Rsrc2;
Hi Rsrel % Rsrc2
Rdest Rsrel & Rsrc2
Rdest Rarel | Rsrc2
Rdest Rarel A Rare2
Rdest(Rarel | Rare2)
Rdest = Rsrel << Rsre2
Rdest = Rsrel >> Rsrc2
(MSB-0)
Rdest Rarel >> Rare2
(MSB preserved)
Rdest = Hi
Rdest Lo
Rdest meen32 Addr
mem32[Addr] Rare
Rdest=Addr
Rdest=label)
if (Rarel Rsrc2)
PC = label
if (Rarel ! Rare2)
PC label
(or
if (Rarel< Rare2)
Rdest 1
else Rdest 0
PC label
Sra PC + 4;
PC label
Jump register
PC- Rare
Jump and link register Sra = PC + 4
PC Rare
system call
depends on the value of
$VO
Immediate
addi
-
andi
ori
Notes
value is signed
string must be termi-
nated with 0
value is signed
returns if $a1-1 char
acters or Enter typed,
the string is termi-
nated with 10
ends simulation
xori
sll
srl
sra
-
slti
Unsigned or Overflow
addu (no overflow trap)
subu (no overflow trap)
divu
.
situ, sitiu
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
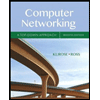
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
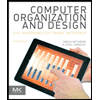
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
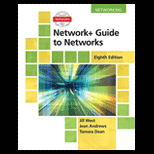
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
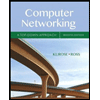
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
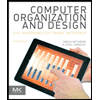
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
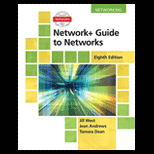
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
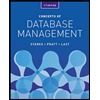
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
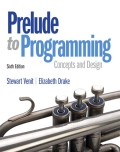
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
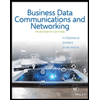
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY