General Description For this assignment, you will read and process historical stock data for the Tesla company. Project Setup and Structure Create a new java project in
General Description For this assignment, you will read and process historical stock data for the Tesla company. Project Setup and Structure Create a new java project in
Chapter3: Using Gui Objects And The Visual Studio Ide
Section: Chapter Questions
Problem 1DE: Each of the following projects in the Chapter.03 folder of your downloadable student files has...
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
-
-
-
General Description
For this assignment, you will read and process historical stock data for the Tesla company.
Project Setup and Structure
- Create a new java project in Eclipse and call it homework01.
- Inside the src folder, create a new java package called hw01.
- All your source code files will need to be placed in the hw01 file.
- Inside the src folder, create a new folder called files.
- Your .csv files should go in files.
- NOTE: When working with the .csv file, your program must use a relative path to the .csv file, NOT an absolute path.
TeslaStock Class
- Create a class called TeslaStock. This class will be used to represent each stock's data in your project. This class should not contain any methods to read/write the data. Only implement the following in the class.
- This class should contain data fields for the date, opening price, low price, high price, and closing price of a stock. All data fields shall have the PRIVATE access modifier..
- Create getters and setters for each data field.
- Create appropriate constructors for this class.
- Implement the toString() method to display a TeslaStock object in the following format:
- Date: 2010-06-29
Opening Price: 3.8
High Price: 5.0
Low Price: 3.508
Closing Price: 4.778 - No other methods are allowed.
StockList Class
- This class will contain the methods necessary to display various information about the stock data.
- This class shall be a subclass of ArrayList<TeslaStock>.
- This class shall not contain any data fields.
- This class shall have a default constructor with no parameters.
- No other constructors are allowed.
- This class shall have the following public, non-static, methods:
- printAllStocks:
- This method shall take no parameters and return nothing.
- This method should print each stock in the StockList to an output file.
- NOTE: This method shall NOT print the data to the console.
- The output file shall be called all_stock_data.txt.
- The data should be printed in a nice and easy to read format.
- displayFirstTenStocks:
- This method shall take no parameters and return nothing.
- This method should display only the first 10 stocks in the StockList in a nice and easy to read format.
- This method should display the data in the console.
- displayLastTenStocks:
- This method shall take no parameters and return nothing.
- This method should display only the last 10 stocks in the StockList in a nice and easy to read format.
- This method should display the data in the console.
- computeAverageOpeningPrice:
- This method shall take no parameters and return a double value.
- This method should compute and return the average opening price of all stock data in the StockList.
- computeAverageClosingPrice:
- This method shall take no parameters and return a double value.
- This method should compute and return the average opening price of all stock data in the StockList.
- findMaxHighPrice:
- This method shall take no parameters and return a single TeslaStock object.
- This method should compute and return the maximum high price of all the stock data.
- findMinLowPrice:
- This method shall take no parameters and return a single TeslaStock object.
- This method should compute and return the minimum low price of all the stock data.
- No other methods are allowed or necessary.
StockReader Class
- This class will contain the method necessary to read and parse the .csv file of stock information.
- This class shall contain a default constructor with no parameters. The constructor should be made private.
- This is to prevent the class from being instantiated since the class will only contain static methods.
- This class shall contain no data fields.
- This class shall have a method called readStockData:
- This method shall be a public, static, method.
- This method shall return a StockList object once all data has been processed.
- This method will take a File object as a parameter. This File object should link to the stock market data in your files folder created previously.
- This method must validate that the given File object is a .csv file. If it is not, then this method shall throw an IllegalArgumentException.
- This method shall read the File object and process all of the stock data into TeslaStock objects and store each object in a StockList.
- NOTE: The data must be parsed so each piece of data is correctly assigned to its respective data field in the TeslaStock class.
- NOTE: You will want to ignore the first line of data as this line contains the header labels for each column of data.
- No other methods are allowed.
Main Class
- This class shall be called Main and will contain your main() method.
- This class shall do the following:
- Create a File object linked to your .csv file.
- NOTE: You do not need to ask the user for the name of the file, you can just hardcode the relative path name into your source code.
- NOTE: Use Relative Path
- Read all the stock data from the file and obtain a StockList object.
- Execute each method from the StockList class and show the results of each method.
- NOTE: For the printAllStocks method, you can show that the file was correctly created and open it during your video presentation.
-
-
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
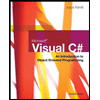
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
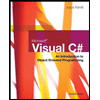
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage