Give a brief description of the problem (Specification and assumptions) of the program below. import java.lang.*; import java.util.*; class BankAccount { double initprincipal; double annualRate; double principal; int quarter=0; double amount; BankAccount(double r, double p) { annualRate=r; principal=p; initprincipal=p; } public void deposit() { Scanner keyboard = new Scanner(System.in); System.out.println("Enter the amount you want to deposit"); amount=Double.parseDouble(keyboard.nextLine()); principal = principal + amount; } public void withdrawal() { Scanner keyboard = new Scanner(System.in); System.out.println("Enter the amount you want to withdraw: "); amount=Double.parseDouble(keyboard.nextLine()); if(principal >= amount) { principal = principal - amount; System.out.println("Balance after withdraw: " + principal); } else { System.out.println("Your balance is less than" + amount + "\tTransaction failed....!!"); } } public void showData(){ while(true){ quarter++; if(quarter==1){ System.out.print("\nYou started with:"+initprincipal); amount=initprincipal+(initprincipal*annualRate/400); System.out.print("\nYour balance after quarter1"+principal); System.out.print("\nYour withdrawals in quarter1"+(amount-principal)); } else if(quarter>=2 && quarter<=4){ System.out.print("\nYou started with:"+principal); amount=principal+(principal*annualRate/400); System.out.print("\nYour balance after quarter"+quarter+":"+principal); System.out.print("Your withdrawals in quarter"+quarter+":"+(amount-principal)); } else return; } } public void quit(){ System.exit(0); } } public class MoneyInBank { public static void main(String[] args) { int choice=0; double rate, principal; while(true) { Scanner keyboard = new Scanner(System.in); System.out.print("Enter the rate: "); rate = Double.parseDouble(keyboard.nextLine()); System.out.print("Enter the initial principal: "); principal = Double.parseDouble(keyboard.nextLine()); BankAccount b=new BankAccount(rate, principal); System.out.println("1. Make a Deposit to the account"); System.out.println("2. Withdraw from the account"); System.out.println("3. Show quarter wise data"); System.out.println("4. Quit"); System.out.println("Enter your choice: "); choice = Integer.parseInt(keyboard.nextLine()); if(choice==1) b.deposit(); else if(choice==2) b.withdrawal(); else if(choice==3) b.showData(); else if(choice==4) b.quit(); else System.out.print("No such option available"); } } }
PLEASE HELP ME :(
Thank you in advance.
PROBLEM:
a. Give a brief description of the problem (Specification and assumptions) of the program below.
import java.lang.*;
import java.util.*;
class BankAccount
{
double initprincipal;
double annualRate;
double principal;
int quarter=0;
double amount;
BankAccount(double r, double p)
{
annualRate=r;
principal=p;
initprincipal=p;
}
public void deposit()
{
Scanner keyboard = new Scanner(System.in);
System.out.println("Enter the amount you want to deposit");
amount=Double.parseDouble(keyboard.nextLine());
principal = principal + amount;
}
public void withdrawal()
{
Scanner keyboard = new Scanner(System.in);
System.out.println("Enter the amount you want to withdraw: ");
amount=Double.parseDouble(keyboard.nextLine());
if(principal >= amount)
{
principal = principal - amount;
System.out.println("Balance after withdraw: " + principal);
}
else
{
System.out.println("Your balance is less than" + amount + "\tTransaction failed....!!");
}
}
public void showData(){
while(true){
quarter++;
if(quarter==1){
System.out.print("\nYou started with:"+initprincipal);
amount=initprincipal+(initprincipal*annualRate/400);
System.out.print("\nYour balance after quarter1"+principal);
System.out.print("\nYour withdrawals in quarter1"+(amount-principal));
}
else if(quarter>=2 && quarter<=4){
System.out.print("\nYou started with:"+principal);
amount=principal+(principal*annualRate/400);
System.out.print("\nYour balance after quarter"+quarter+":"+principal);
System.out.print("Your withdrawals in quarter"+quarter+":"+(amount-principal));
}
else
return;
}
}
public void quit(){
System.exit(0);
}
}
public class MoneyInBank
{
public static void main(String[] args) {
int choice=0;
double rate, principal;
while(true)
{
Scanner keyboard = new Scanner(System.in);
System.out.print("Enter the rate: ");
rate = Double.parseDouble(keyboard.nextLine());
System.out.print("Enter the initial principal: ");
principal = Double.parseDouble(keyboard.nextLine());
BankAccount b=new BankAccount(rate, principal);
System.out.println("1. Make a Deposit to the account");
System.out.println("2. Withdraw from the account");
System.out.println("3. Show quarter wise data");
System.out.println("4. Quit");
System.out.println("Enter your choice: ");
choice = Integer.parseInt(keyboard.nextLine());
if(choice==1)
b.deposit();
else if(choice==2)
b.withdrawal();
else if(choice==3)
b.showData();
else if(choice==4)
b.quit();
else
System.out.print("No such option available");
}
}
}

Step by step
Solved in 3 steps

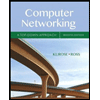
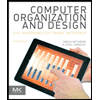
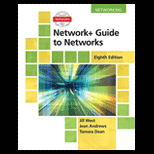
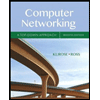
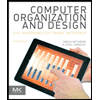
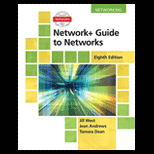
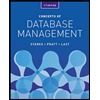
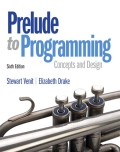
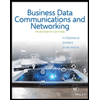