Given an intNode struct, write the operating functions for a linked list by completing the following functions. 1. IntNode* IntNode Create (int initData); - Create a new node on the heap which stores the value initData 2. void IntNode.insertAfter (IntNode" this Node, IntNode* newNode); - Insert newNode in the linked list after this Node 3. void IntNode PrintNodeData(IntNode* thisNode):-Print out the value stored in the node, if valid 4. IntNode* IntNode.GetNext(IntNode* thisNode); - Return the address of the next node of the given this Node 5. int IntNode Length(intNode* firstNode):- Return the length (number of nodes) in the list 6. IntNode IntNode_GetNth (IntNode* firstNode, int n)-Return a pointer to the nth node of the list starting at firstNode. 7. void IntNode_PrintList (IntNode* firstNode)-Call IntNode PrintNodeData() to output values of the list starting at firstNode. Do not add extra space characters in between values. 8 int IntNode SumList (IntNode* firstNode) - Return the sum of the values of all nodes starting at firstNode. Note: A linked list is simply a chain of IntNode structures. Refer to chapter 7 of the zybook for more information on a linked list. To store a linked list object, we simply store a pointer to the first node in the linked list. That first node then points to the next node in the list, and so on, until we reach a node that points to NULL instead of an additional node. A node in the list which points to NULL is the last node in the list. Your main should read 5 integers from a user 1. The number of nodes to be added to a new list 2. The value of the first node of the list 3. An increment between the values of two consecutive nodes 4. A value of a new node 5. The position of a node after which the new node will be added, with 1 indicating the first node With this, you should create a linked list with the specified number of nodes in the list (user value 1). The value of the first node should be user value 2. Each of the remaining nodes should store values, each incremented by the increment value from the previous value (user value 3) Next, print out the list using the print list function you wrote. Then, print out the list starting from the second Node. If there is no second node, print out 'No second element" in its place. Next, print out the sum of the values stored in the list. Finally, add a new node with the 4th specified user value after the node located at the specified location in the list (look at user input value
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
IN C
I want accurate code with output screenshot
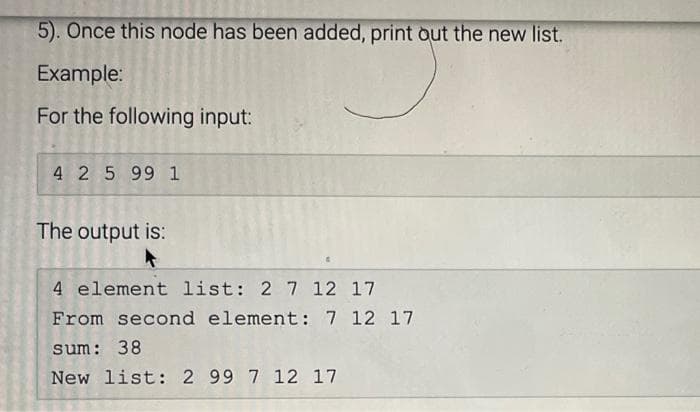
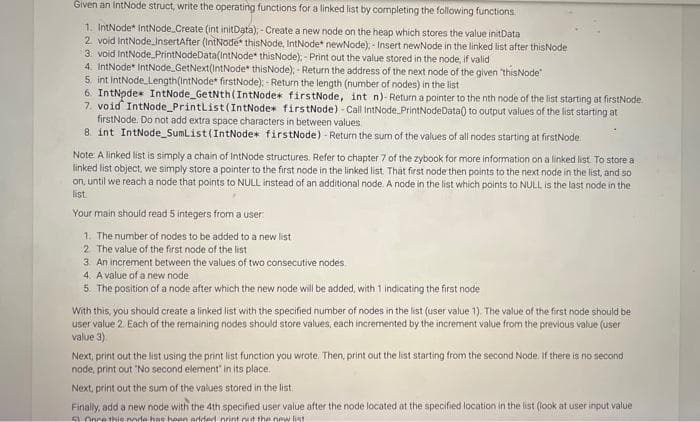

Step by step
Solved in 4 steps with 2 images

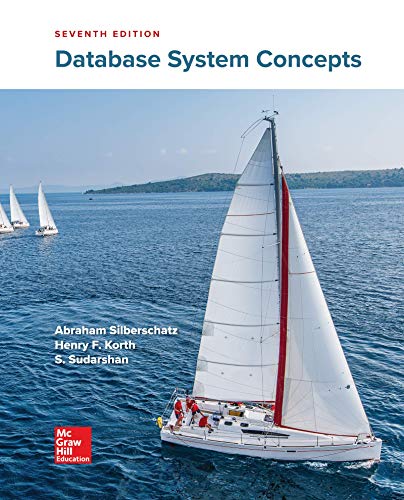
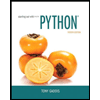
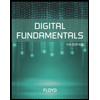
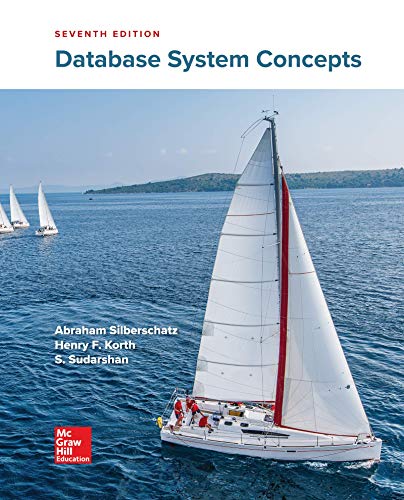
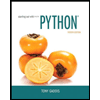
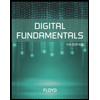
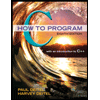
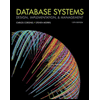
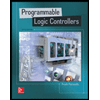