Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the student's GPA (double). Assume student's name has a maximum length of 20 characters. Implement the Student struct and related function declarations in Student.h, and implement the related function definitions in Student.c as listed below: • Student InitStudent() - initializes name to "Louie" and gpa to 1.0 • Student SetName(char *name, Student s) - sets the student's name • Student SetGPA(double gpa, Student s) - sets the student's GPA • void GetName(char* studentName, Student s) - return the student's name in studentName • double GetGPA(Student s) - returns the students GPA Ex. If a new Student object is created, the default output is: Louie/1.0 Ex. If the student's name is set to "Felix" and the GPA is set to 3.7, the output becomes: Felix/3.7 File is marked as read only 1 #include 2 #include 3 4 #include "Student.h" 6 int main() { 7 8 9 10 11 12 13 14 15 16 17 18 19 } Current file: main.c Student student = InitStudent (); char name[20]; GetName (name, student); printf("%s/%.11f\n", name, GetGPA (student)); student SetName("Felix", student); student = Set GPA (3.7, student); GetName (name, student); printf("%s/%.11f\n", name, GetGPA (student)); return 0;
Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the student's GPA (double). Assume student's name has a maximum length of 20 characters. Implement the Student struct and related function declarations in Student.h, and implement the related function definitions in Student.c as listed below: • Student InitStudent() - initializes name to "Louie" and gpa to 1.0 • Student SetName(char *name, Student s) - sets the student's name • Student SetGPA(double gpa, Student s) - sets the student's GPA • void GetName(char* studentName, Student s) - return the student's name in studentName • double GetGPA(Student s) - returns the students GPA Ex. If a new Student object is created, the default output is: Louie/1.0 Ex. If the student's name is set to "Felix" and the GPA is set to 3.7, the output becomes: Felix/3.7 File is marked as read only 1 #include 2 #include 3 4 #include "Student.h" 6 int main() { 7 8 9 10 11 12 13 14 15 16 17 18 19 } Current file: main.c Student student = InitStudent (); char name[20]; GetName (name, student); printf("%s/%.11f\n", name, GetGPA (student)); student SetName("Felix", student); student = Set GPA (3.7, student); GetName (name, student); printf("%s/%.11f\n", name, GetGPA (student)); return 0;
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter6: User-defined Functions
Section: Chapter Questions
Problem 17PE
Related questions
Question
Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the student's GPA (double). Assume student's name has a maximum length of 20 characters.
![Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the
student's GPA (double). Assume student's name has a maximum length of 20 characters.
Implement the Student struct and related function declarations in Student.h, and implement the related function definitions in Student.c as
listed below:
• Student InitStudent() - initializes name to "Louie" and gpa to 1.0
• Student SetName(char *name, Student s) - sets the student's name
• Student SetGPA(double gpa, Student s) - sets the student's GPA
• void GetName(char* studentName, Student s) - return the student's name in studentName
• double GetGPA(Student s) - returns the students GPA
Ex. If a new Student object is created, the default output is:
Louie/1.0
Ex. If the student's name is set to "Felix" and the GPA is set to 3.7, the output becomes:
Felix/3.7
File is marked as read only
1 #include <stdio.h>
2 #include <string.h>
4 #include "Student.h"
5
6 int main() {
8
9
10
11
12
13
14
15
16
47895
17
18
19 }
Current file: main.c _
Student student = InitStudent ();
char name [20];
GetName(name, student);
printf("%s/%.11f\n", name, GetGPA (student));
student = SetName("Felix", student);
student SetGPA (3.7, student);
GetName(name, student);
printf("%s/%.11f\n", name, GetGPA(student));
return 0;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5779ee7a-d811-43a6-ba75-7d41285b789d%2F72eeba0d-942f-4d35-8bf1-53386d927729%2Flma64t_processed.png&w=3840&q=75)
Transcribed Image Text:Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the
student's GPA (double). Assume student's name has a maximum length of 20 characters.
Implement the Student struct and related function declarations in Student.h, and implement the related function definitions in Student.c as
listed below:
• Student InitStudent() - initializes name to "Louie" and gpa to 1.0
• Student SetName(char *name, Student s) - sets the student's name
• Student SetGPA(double gpa, Student s) - sets the student's GPA
• void GetName(char* studentName, Student s) - return the student's name in studentName
• double GetGPA(Student s) - returns the students GPA
Ex. If a new Student object is created, the default output is:
Louie/1.0
Ex. If the student's name is set to "Felix" and the GPA is set to 3.7, the output becomes:
Felix/3.7
File is marked as read only
1 #include <stdio.h>
2 #include <string.h>
4 #include "Student.h"
5
6 int main() {
8
9
10
11
12
13
14
15
16
47895
17
18
19 }
Current file: main.c _
Student student = InitStudent ();
char name [20];
GetName(name, student);
printf("%s/%.11f\n", name, GetGPA (student));
student = SetName("Felix", student);
student SetGPA (3.7, student);
GetName(name, student);
printf("%s/%.11f\n", name, GetGPA(student));
return 0;
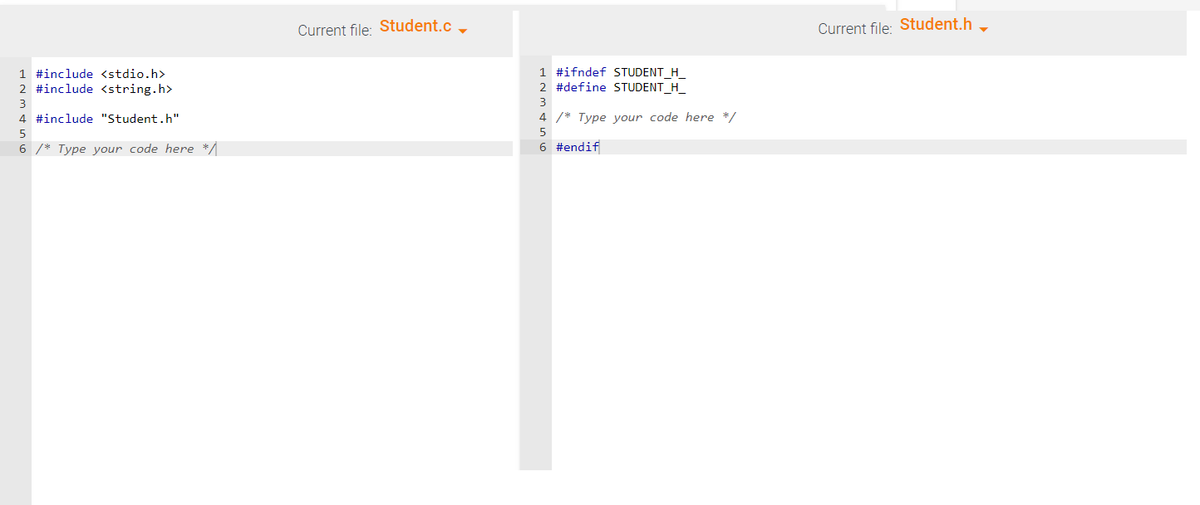
Transcribed Image Text:1 #include <stdio.h>
2 #include <string.h>
3
4 #include "Student.h"
5
6 /* Type your code here */
Current file: Student.c
1 #ifndef STUDENT H
2 #define STUDENT_H_
3
4 /* Type your code here */
5
6 #endif
Current file: Student.h
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
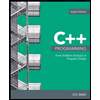
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
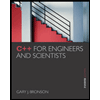
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
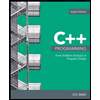
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
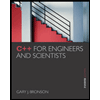
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr