Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts public class ItemNode { private String item; private ItemNode nextNodeRef; // Reference to the next node public ItemNode() { item = ""; nextNodeRef = null; } // Constructor public ItemNode(String itemInit) { this.item = itemInit; this.nextNodeRef = null; } // Constructor public ItemNode(String itemInit, ItemNode nextLoc) { this.item = itemInit; this.nextNodeRef = nextLoc; } // Insert node after this node. public void insertAfter(ItemNode nodeLoc) { ItemNode tmpNext; tmpNext = this.nextNodeRef; this.nextNodeRef = nodeLoc; nodeLoc.nextNodeRef = tmpNext; } // TODO: Define insertAtEnd() method that inserts a node // to the end of the linked list // Get location pointed by nextNodeRef public ItemNode getNext() { return this.nextNodeRef; } public void printNodeData() { System.out.println(this.item); } }
Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node.
Ex. if the input is:
4 Kale Lettuce Carrots Peanuts
where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list.
The output is:
Kale Lettuce Carrots Peanuts
public class ItemNode {
private String item;
private ItemNode nextNodeRef; // Reference to the next node
public ItemNode() {
item = "";
nextNodeRef = null;
}
// Constructor
public ItemNode(String itemInit) {
this.item = itemInit;
this.nextNodeRef = null;
}
// Constructor
public ItemNode(String itemInit, ItemNode nextLoc) {
this.item = itemInit;
this.nextNodeRef = nextLoc;
}
// Insert node after this node.
public void insertAfter(ItemNode nodeLoc) {
ItemNode tmpNext;
tmpNext = this.nextNodeRef;
this.nextNodeRef = nodeLoc;
nodeLoc.nextNodeRef = tmpNext;
}
// TODO: Define insertAtEnd() method that inserts a node
// to the end of the linked list
// Get location pointed by nextNodeRef
public ItemNode getNext() {
return this.nextNodeRef;
}
public void printNodeData() {
System.out.println(this.item);
}
}
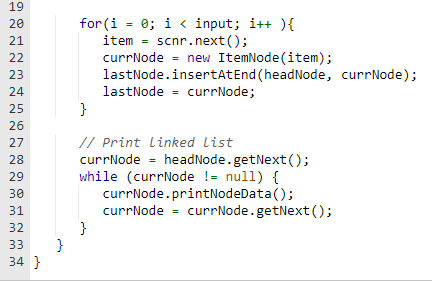
![File is marked as read only
1 import java.util.Scanner;
3 public class ShoppingList {
IN35∞ H
2
4
6
7
8
9
10 |
11
12
13
14
15
16
17
18
public static void main (String[] args) {
Scanner scnr = new Scanner (System.in);
ItemNode headNode; // Create intNode objects
ItemNode currNode;
ItemNode lastNode;
String item;
int i;
Current file: ShoppingList.java ❤
// Front of nodes List
headNode = new ItemNode();
lastNode headNode;
int input = scnr.nextInt():](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2F6f47c2c5-1197-4700-a0aa-894b2e4e68e5%2Fm10mb4p_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

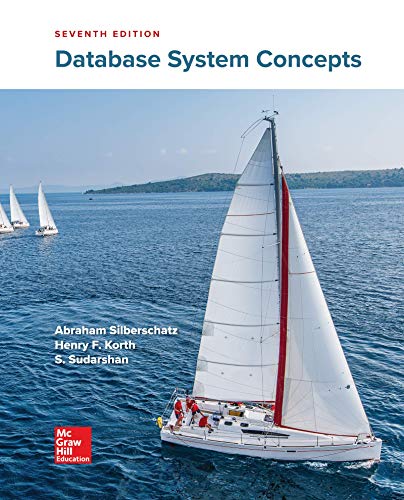
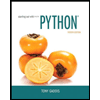
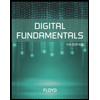
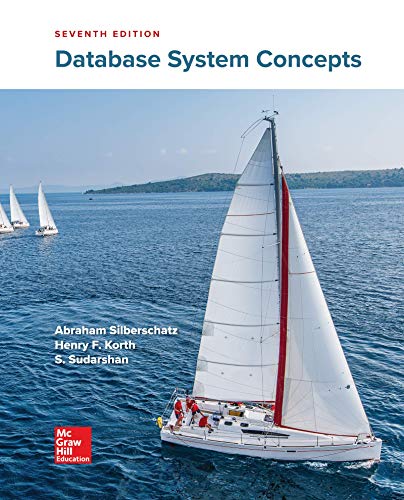
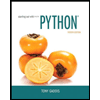
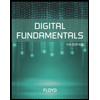
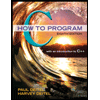
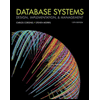
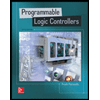