Implement the following: 1. Add the missing functionality in “controller.java” to finish the “sellLIFO” and “sellFIFO” methods. 2. Provide error checking so users can’t sell a stock they don’t own or try to sell more stock than they own. Eliminate that runtime exception. 3. We think its sort of limited to only work with just two stocks. You need to change the user input, so the user enters the stock name they wish to buy or sell instead of just choosing between Google and Amazon. Keep the command line interface since we are only in the testing phase. 4. We like the layout and design of the application so don’t change any of the other methods or objects. Only edit the Controller.java file!
Given the following code in Java and attached images( containing related code):
“sellLIFO” and “sellFIFO” methods.
2. Provide error checking so users can’t sell a stock they don’t own or
try to sell more stock than they own. Eliminate that runtime exception.
3. We think its sort of limited to only work with just two stocks. You
need to change the user input, so the user enters the stock name they
wish to buy or sell instead of just choosing between Google and
Amazon. Keep the command line interface since we are only in the
testing phase.
4. We like the layout and design of the application so don’t change any
of the other methods or objects. Only edit the Controller.java file!
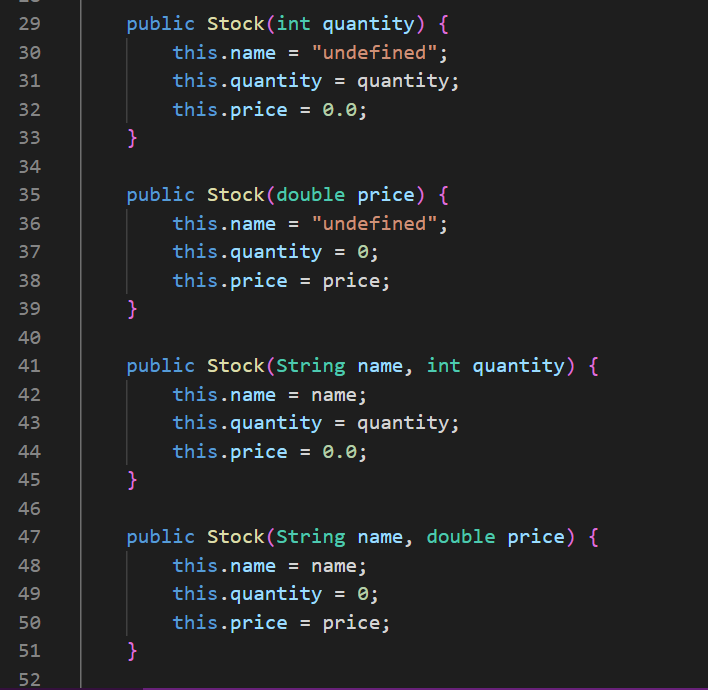
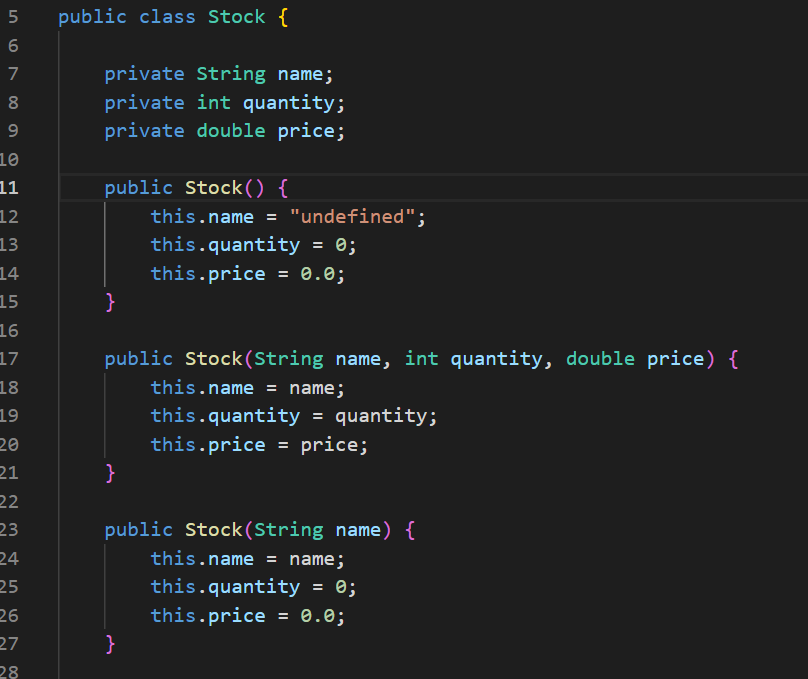

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 6 images

Given the code:
import java.util.HashMap;
import java.util.LinkedList;
import java.util.Scanner;
public class Controller {
privateHashMap<String,LinkedList<Stock>>stockMap;
publicController(){
stockMap=newHashMap<>();
Scannerinput=newScanner(System.in);
do{
// Prompt for stock name or option to quit
System.out.print("Enter stock name or 3 to quit: ");
StringstockName=input.next();
if(stockName.equals("3")){
break;// Exit if user inputs '3'
}
// Get or create a list for the specified stock
LinkedList<Stock>stockList=stockMap.computeIfAbsent(stockName,k->newLinkedList<>());
// Prompt to buy or sell
System.out.print("Input 1 to buy, 2 to sell: ");
intcontrolNum=input.nextInt();
System.out.print("How many stocks: ");
intquantity=input.nextInt();
if(controlNum==1){
// Buying stocks
System.out.print("At what price: ");
doubleprice=input.nextDouble();
buyStock(stockList,stockName,quantity,price);
}else{
// Selling stocks
System.out.print("Press 1 for LIFO accounting, 2 for FIFO accounting: ");
controlNum=input.nextInt();
if(controlNum==1){
// Sell using LIFO method
sellLIFO(stockList,quantity);
}else{
// Sell using FIFO method
sellFIFO(stockList,quantity);
}
}
}while(true);
input.close();// Close the scanner
}
publicstaticvoidbuyStock(LinkedList<Stock>list,Stringname,intquantity,doubleprice){
// Create a new stock object and add it to the list
Stocktemp=newStock(name,quantity,price);
list.push(temp);
// Display purchase details
System.out.printf("You bought %d shares of %s stock at $%.2f per share %n",quantity,name,price);
}
publicstaticvoidsellLIFO(LinkedList<Stock>list,intnumToSell){
// Check if there are enough stocks to sell
inttotalQuantity=list.stream().mapToInt(Stock::getQuantity).sum();
if(totalQuantity<numToSell){
System.out.println("Not enough stocks to sell.");
return;
}
doubletotal=0;
doubleprofit=0;
intremainingToSell=numToSell;
while(remainingToSell>0){
// Retrieve and sell stocks using LIFO
StocklastStock=list.peek();
if(lastStock.getQuantity()<=remainingToSell){
remainingToSell-=lastStock.getQuantity();
total+=lastStock.getPrice()*lastStock.getQuantity();
profit+=lastStock.getPrice()*lastStock.getQuantity();
list.pop();// Remove stock from the list
}else{
lastStock.setQuantity(lastStock.getQuantity()-remainingToSell);
total+=lastStock.getPrice()*remainingToSell;
profit+=lastStock.getPrice()*remainingToSell;
remainingToSell=0;
}
}
// Display sale details
System.out.printf("You sold %d shares of %s stock at %.2f per share %n",numToSell,list.peek().getName(),total/numToSell);
System.out.printf("You made $%.2f on the sale %n",profit);
}
publicstaticvoidsellFIFO(LinkedList<Stock>list,intnumToSell){
// Check if there are enough stocks to sell
inttotalQuantity=list.stream().mapToInt(Stock::getQuantity).sum();
if(totalQuantity<numToSell){
System.out.println("Not enough stocks to sell.");
return;
}
doubletotal=0;
doubleprofit=0;
intremainingToSell=numToSell;
while(remainingToSell>0){
// Retrieve and sell stocks using FIFO
StockfirstStock=list.peekLast();// Get the first element (FIFO)
if(firstStock.getQuantity()<=remainingToSell){
remainingToSell-=firstStock.getQuantity();
total+=firstStock.getPrice()*firstStock.getQuantity();
profit+=firstStock.getPrice()*firstStock.getQuantity();
list.removeLast();// Remove stock from the list (FIFO)
}else{
firstStock.setQuantity(firstStock.getQuantity()-remainingToSell);
total+=firstStock.getPrice()*remainingToSell;
profit+=firstStock.getPrice()*remainingToSell;
remainingToSell=0;
}
}
// Display sale details
System.out.printf("You sold %d shares of %s stock at %.2f per share %n",numToSell,list.peekLast().getName(),total/numToSell);
System.out.printf("You made $%.2f on the sale %n",profit);
}
}
Rewrite without using Hashmap, but just stick with the LinkedList instead! The output should display the profit made from sales!
If I bought 30 stocks of Google for 120 then I decide to sell 5 Google stocks at 140, then the output should display the gained profit!
It didn't solve my question, the console should ask how many stocks I want to sell and at what price. Then it should print out the amount made from selling the shares. When it gives me the option to select 1 and 2 for sellLIFO and sellFIFO it gives "Not enough stocks to sell" which should not be the case if I have 20 stocks bought and I want to sell 3!
Please fix!
The code using only the LinkedList doesn't produce the right output now, it gives this again. Refer to image
The output should produce the amount made from the shares using only the LinkedList instead of the Hashmap.
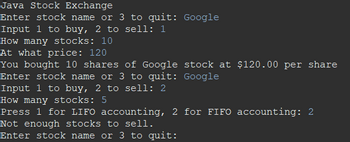
Thank you, it has the correct output now!
Last thing, can this be done without using a Hashmap, but instead with the original method of using just the LinkedList in the original question? If so, please provide another solution without using hashmap to see the difference in structure of the code!
The code is not working the way it should be. If I buy 20 stocks, I should be able to sell 3. The output should be the profit loss/gain not "Not enough stocks to sell".
Refer to the image to see the problem
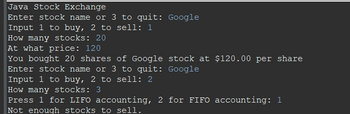
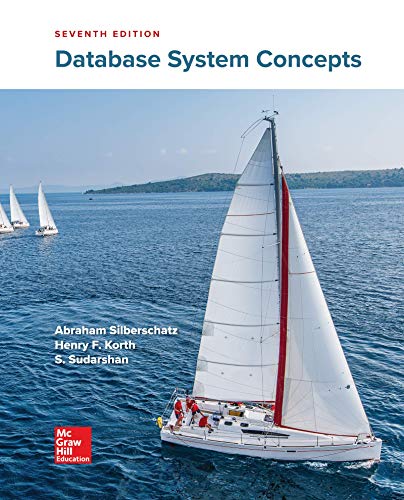
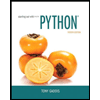
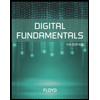
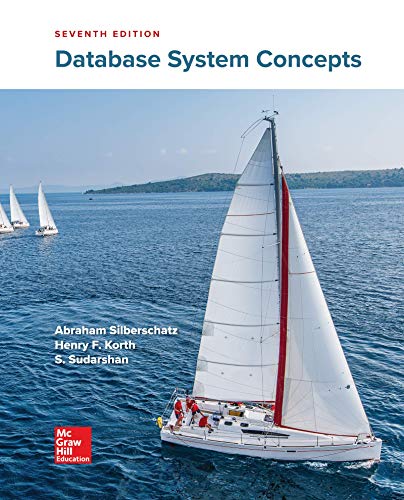
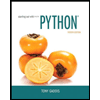
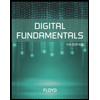
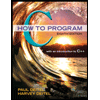
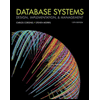
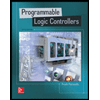