group of statisticians at a local college has asked you to create a set of functions that compute the median and mode of a set of numbers, as defined in the below sample programs: · mode.py · median.py Define these functions in a module named stats.py. Also include a function named mean, which computes the average of a set of numbers. Each function should expect a list of numbers as an argument and return a single number. Each function should return 0 if the list is empty. Include a main function that tests the three statistical functions with a given list.
A group of statisticians at a local college has asked you to create a set of functions that compute the median and mode of a set of numbers, as defined in the below sample programs:
· mode.py
· median.py
Define these functions in a module named stats.py. Also include a function named mean, which computes the average of a set of numbers. Each function should expect a list of numbers as an argument and return a single number. Each function should return 0 if the list is empty. Include a main function that tests the three statistical functions with a given list.
***mode.py code***
"""
File: mode.py
Prints the mode of a set of numbers in a file.
"""
fileName = input("Enter the file name: ")
f = open(fileName, 'r')
# Input the text, convert its to words to uppercase, and
# add the words to a list
words = []
for line in f:
wordsInLine = line.split()
for word in wordsInLine:
words.append(word.upper())
# Obtain the set of unique words and their
# frequencies, saving these associations in
# a dictionary
theDictionary = {}
for word in words:
number = theDictionary.get(word, None)
if number == None:
# word entered for the first time
theDictionary[word] = 1
else:
# word already seen, increment its number
theDictionary[word] = number + 1
# Find the mode by obtaining the maximum value
# in the dictionary and determining its key
theMaximum = max(theDictionary.values())
for key in theDictionary:
if theDictionary[key] == theMaximum:
print("The mode is", key)
break
***median.py code***
"""
File: median.py
Prints the median of a set of numbers in a file.
"""
fileName = input("Enter the file name: ")
f = open(fileName, 'r')
# Input the text, convert it to numbers, and
# add the numbers to a list
numbers = []
for line in f:
words = line.split()
for word in words:
numbers.append(float(word))
# Sort the list and print the number at its midpoint
numbers.sort()
midpoint = len(numbers) // 2
print("The median is", end=" ")
if len(numbers) % 2 == 1:
print(numbers[midpoint])
else:
print((numbers[midpoint] + numbers[midpoint - 1]) / 2)
![median.py
1
2
File: median.py
3
Prints the median of a set of numbers in a file.
4
5
fileName = input("Enter the file name: ")
f = open(fileName, 'r')
%3D
8
9.
# Input the text, convert it to numbers, and
10
# add the numbers to a list
11
numbers =
[]
12
for line in f:
13
words = line.split()
14
for word in words:
15
numbers.append(float(word))
16
# Sort the list and print the number at its midpoint
numbers.sort()
17
18
len(numbers) // 2
midpoint
print("The median is", end=" ")
if len(numbers) % 2 == 1:
print(numbers[midpoint])
19
20
21
22
23
else:
24
print((numbers[midpoint] + numbers[midpoint - 1]) / 2)
67](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb296c980-a286-4075-beee-6899fcd06d3e%2F791cf4bf-16f4-41cc-91db-21b43890bb68%2Fe9k5oqx_processed.png&w=3840&q=75)
![mode.py
1
2
File: mode.py
3
Prints the mode of a set of numbers in a file.
4
5
fileName = input("Enter the file name: ")
f = open(fileName, 'r')
7
%3D
8
9.
# Input the text, convert its to words to uppercase, and
10
# add the words to a list
11
words = []
12
for line in f:
13
wordsInline = line.split()
14
for word in wordsInLine:
15
words.append(word.upper())
16
# Obtain the set of unique words and their
# frequencies, saving these associations in
# a dictionary
17
18
19
20
theDictionary
{}
21
for word in words:
number = theDictionary.get(word, None)
22
23
if number == None:
=D3D
24
# word entered for the first time
25
theDictionary[word] = 1
26
else:
# word already seen, increment its number
theDictionary[word] = number + 1
27
28
29
# Find the mode by obtaining the maximum value
# in the dictionary and determining its key
theMaximum = max(theDictionary.values())
30
31
32
for key in theDictionary:
if theDictionary[key]
33
34
theMaximum:
35
print("The mode is", key)
36
break
37](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb296c980-a286-4075-beee-6899fcd06d3e%2F791cf4bf-16f4-41cc-91db-21b43890bb68%2F3hz42t9_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

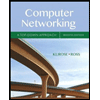
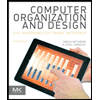
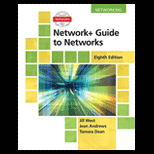
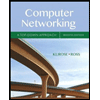
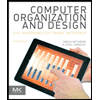
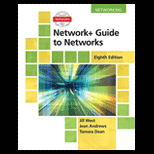
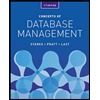
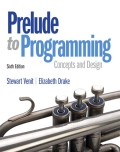
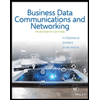