have written this rock, scissors, and paper game code and it runs fine. but I need a two dimensional array that have to keep and print a record of the games played, which would say how many times the user won, how many times the computer won and how many times the games were tied, could you help me with this please? This is the code that need the two dimensional array mentioned above, don't forget the indents: import random print("Let's play the Rock Paper Scissors game!") def playerchoice(): ## Error Trap for choice while True: print("Rock = 1\nPaper = 2\nScissors = 3") try: player = int(input("Choose your move: ")) if player <= 0 or player >= 4: raise ValueError("Wrong move! Please choose 1, 2, or 3") else: return player except ValueError as wrongmsg: print(wrongmsg) ## Comparing choice with a function def result(player,computer): if player == computer: print("Computer chose",player,"\nTie Game") return 0 if player == 1 and computer == 3: print("Computer chose 3\nPlayer won") return 1 if player == 1 and computer == 2: print("Computer chose 2\nComputer won") return 2 if player == 2 and computer == 1: print("Computer chose 1\nPlayer won") return 1 if player == 2 and computer == 3: print("Computer chose 3\nComputer won") return 2 if player == 3 and computer == 2: print("Computer chose 2\nPlayer won") return 1 if player == 3 and computer == 1: print("Computer chose 1\nComputer won") return 2 PlayAgainNpt = "y" player_score = 0 computer_score = 0 total_games = [] game_count = 0 tie_games = 0 while True: player_choice = playerchoice() ## Computer will randomly choose a move computer_choice = random.randint(1,3) res = result(player_choice,computer_choice) toAppend = (1,0) if res == 1: player_score +=1 if res == 2: computer_score +=1 if res == 0: tie_games +=1 game_count +=1 total_games.append([game_count, toAppend]) PlayAgainNpt = input("Do you want to play again?(y/n): ") if PlayAgainNpt == "n": print("\nTotal games played:",game_count) print("Scores") print("Player won:",player_score) print("Computer won:",computer_score) print() break
I have written this rock, scissors, and paper game code and it runs fine. but I need a two dimensional array that have to keep and print a record of the games played, which would say how many times the user won, how many times the computer won and how many times the games were tied, could you help me with this please?
This is the code that need the two dimensional array mentioned above, don't forget the indents:
import random
print("Let's play the Rock Paper Scissors game!")
def playerchoice():
## Error Trap for choice
while True:
print("Rock = 1\nPaper = 2\nScissors = 3")
try:
player = int(input("Choose your move: "))
if player <= 0 or player >= 4:
raise ValueError("Wrong move! Please choose 1, 2, or 3")
else:
return player
except ValueError as wrongmsg:
print(wrongmsg)
## Comparing choice with a function
def result(player,computer):
if player == computer:
print("Computer chose",player,"\nTie Game")
return 0
if player == 1 and computer == 3:
print("Computer chose 3\nPlayer won")
return 1
if player == 1 and computer == 2:
print("Computer chose 2\nComputer won")
return 2
if player == 2 and computer == 1:
print("Computer chose 1\nPlayer won")
return 1
if player == 2 and computer == 3:
print("Computer chose 3\nComputer won")
return 2
if player == 3 and computer == 2:
print("Computer chose 2\nPlayer won")
return 1
if player == 3 and computer == 1:
print("Computer chose 1\nComputer won")
return 2
PlayAgainNpt = "y"
player_score = 0
computer_score = 0
total_games = []
game_count = 0
tie_games = 0
while True:
player_choice = playerchoice()
## Computer will randomly choose a move
computer_choice = random.randint(1,3)
res = result(player_choice,computer_choice)
toAppend = (1,0)
if res == 1:
player_score +=1
if res == 2:
computer_score +=1
if res == 0:
tie_games +=1
game_count +=1
total_games.append([game_count, toAppend])
PlayAgainNpt = input("Do you want to play again?(y/n): ")
if PlayAgainNpt == "n":
print("\nTotal games played:",game_count)
print("Scores")
print("Player won:",player_score)
print("Computer won:",computer_score)
print()
break

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

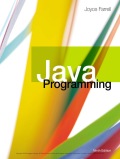
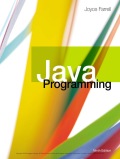