Here is the code I've tried. Although I used the full path to the file and the file is located in the same directory as my program executable, when I run it is doesn't read the text file #include #include #include #include using namespace std; void loadArrays(string product[], int quantity[], float cost[], int& counter) { ifstream file; //ifstream function string inLine, prod, qty, cst; //variables to hold read line temporarily //open input file file.open("C:\\Users\\Honeypie\\source\\repos\\Project4\\inventory.txt", ios::in); while (getline(file, inLine)) { //read first line istringstream ss(inLine); //split the line using a delimeter //you may change this delimeter according to your input file getline(ss, prod, '|'); getline(ss, qty, '|'); getline(ss, cst, '|'); if (prod != "Product Code") { //if statement that will ignore the column headers product[counter] = prod; //insert product code to product array quantity[counter] = stoi(qty); //insert quantity to quantity array. stoi is for string to int conversion. cost[counter] = stof(cst); //insert cost to cost array. stof is for string to float conversion. counter++; //add count value. } } file.close(); } void calculateValues(int quantity[], float cost[], float value[], int& counter) { /*calculate for the total value of each product then insert it in value array.*/ for (int i = 0; i < counter; i++) { value[i] = quantity[i] * cost[i]; } } string GetProd(string product[], float value[], int& counter) { string tempProd = product[0]; //declare a temporary product with the highest value. float tempValue = value[0]; //declare a temporary highest value. for (int i = 0; i < counter; i++) { if (value[i] > tempValue) { //if the current value is greater than temporary highest value. tempValue = value[i]; //change the tempValue with the new highest value. tempProd = product[i]; //change the tempProd with the product of the new highest value. } } //return the product with the highest value. return tempProd; } float GetAverage(int quantity[], float value[], int& counter) { float totalVal = 0, aveVal = 0; int totalQty = 0; for (int i = 0; i < counter; i++) { //addition of all quantities and values from the array. totalQty = totalQty + quantity[i]; totalVal = totalVal + value[i]; } //get average value by dividing total value by the total quantity on hand. aveVal = totalVal / totalQty; return aveVal; //return the average value. } void displayTable(string product[], int quantity[], float cost[], float value[], int& counter) { /* This function displays the table with the respective values Displays the product with the highest value Displays the average value of an inventory item */ cout << "Product Code\tQty on Hand\tCost Each\tTotal Value" << endl; for (int i = 0; i < counter; i++) { cout << product[i]; cout << right << setw(22) << quantity[i]; cout << right << setw(14) << cost[i]; cout << right << setw(18) << value[i]; if (value[i] > 9000) { cout << " !!!"; } cout << endl; } cout << endl; //call function GetProd to get the product with the highest value. cout << "The product with the highest inventory value is " << GetProd(product, value, counter) << "." << endl; //call GetAverage function to get the average value. //setprecision() makes float display 2 decimal places. cout << fixed << setprecision(2) << "The average cost of an inventory item is $" << GetAverage(quantity, value, counter) << "." << endl; } int main() { const int SIZE = 20; //declaring the array size. /* Declaration of array for product, quantity, cost, and value. Declaration of variable counter. */ string product[SIZE]; int quantity[SIZE], counter = 0; float cost[SIZE], value[SIZE]; //calls the loadArrays function to read data from file then insert it to the arrays. loadArrays(product, quantity, cost, counter); //calls calculateValues function to compute for the total value of each product. calculateValues(quantity, cost, value, counter); //calls displayTable function to display all the gathered and computed data. displayTable(product, quantity, cost, value, counter); return 0; }
Here is the code I've tried. Although I used the full path to the file and the file is located in the same directory as my program executable, when I run it is doesn't read the text file
#include <iostream>
#include <fstream>
#include <sstream>
#include <iomanip>
using namespace std;
void loadArrays(string product[], int quantity[], float cost[], int& counter) {
ifstream file; //ifstream function
string inLine, prod, qty, cst; //variables to hold read line temporarily
//open input file
file.open("C:\\Users\\Honeypie\\source\\repos\\Project4\\inventory.txt", ios::in);
while (getline(file, inLine)) { //read first line
istringstream ss(inLine);
//split the line using a delimeter
//you may change this delimeter according to your input file
getline(ss, prod, '|');
getline(ss, qty, '|');
getline(ss, cst, '|');
if (prod != "Product Code") { //if statement that will ignore the column headers
product[counter] = prod; //insert product code to product array
quantity[counter] = stoi(qty); //insert quantity to quantity array. stoi is for string to int conversion.
cost[counter] = stof(cst); //insert cost to cost array. stof is for string to float conversion.
counter++; //add count value.
}
}
file.close();
}
void calculateValues(int quantity[], float cost[], float value[], int& counter) {
/*calculate for the total value of each product
then insert it in value array.*/
for (int i = 0; i < counter; i++) {
value[i] = quantity[i] * cost[i];
}
}
string GetProd(string product[], float value[], int& counter) {
string tempProd = product[0]; //declare a temporary product with the highest value.
float tempValue = value[0]; //declare a temporary highest value.
for (int i = 0; i < counter; i++) {
if (value[i] > tempValue) { //if the current value is greater than temporary highest value.
tempValue = value[i]; //change the tempValue with the new highest value.
tempProd = product[i]; //change the tempProd with the product of the new highest value.
}
}
//return the product with the highest value.
return tempProd;
}
float GetAverage(int quantity[], float value[], int& counter) {
float totalVal = 0, aveVal = 0;
int totalQty = 0;
for (int i = 0; i < counter; i++) {
//addition of all quantities and values from the array.
totalQty = totalQty + quantity[i];
totalVal = totalVal + value[i];
}
//get average value by dividing total value by the total quantity on hand.
aveVal = totalVal / totalQty;
return aveVal; //return the average value.
}
void displayTable(string product[], int quantity[], float cost[], float value[], int& counter) {
/*
This function displays the table with the respective values
Displays the product with the highest value
Displays the average value of an inventory item
*/
cout << "Product Code\tQty on Hand\tCost Each\tTotal Value" << endl;
for (int i = 0; i < counter; i++) {
cout << product[i];
cout << right << setw(22) << quantity[i];
cout << right << setw(14) << cost[i];
cout << right << setw(18) << value[i];
if (value[i] > 9000) {
cout << " !!!";
}
cout << endl;
}
cout << endl;
//call function GetProd to get the product with the highest value.
cout << "The product with the highest inventory value is " << GetProd(product, value, counter) << "." << endl;
//call GetAverage function to get the average value.
//setprecision() makes float display 2 decimal places.
cout << fixed << setprecision(2) << "The average cost of an inventory item is $" << GetAverage(quantity, value, counter) << "." << endl;
}
int main()
{
const int SIZE = 20; //declaring the array size.
/*
Declaration of array for product,
quantity, cost, and value.
Declaration of variable counter.
*/
string product[SIZE];
int quantity[SIZE], counter = 0;
float cost[SIZE], value[SIZE];
//calls the loadArrays function to read data from file then insert it to the arrays.
loadArrays(product, quantity, cost, counter);
//calls calculateValues function to compute for the total value of each product.
calculateValues(quantity, cost, value, counter);
//calls displayTable function to display all the gathered and computed data.
displayTable(product, quantity, cost, value, counter);
return 0;
}
![Read the input file into the arrays. Allow for a file with an unknown number of input lines (up to SIZE), so
use an EOF loop with a counter to keep track of how many items were read in. Return this count using
the reference parameter.
1. void calculateValues(int[], float[], float[], int);
Fill the second float array with the value of each inventory item by multiplying the quantity by the cost.
1. void displayTable(string[], int[], float[], float[], int);
Display a neatly formatted data table with headings and one output line per product, showing the
product code, the quantity on hand, the cost each, and the total value.
Display an indicator if the total value for a product is over $9,000.
Display which product has the highest total value.
Display the average value of an inventory item. (Divide the total value by the total quantity on hand).
Display all dollar amounts to two decimal places.
1. Functions must pass parameters and return values as needed, using only local variables. Global
variables are not allowed.
2. Create any other functions or variables as you see fit.
3. Use comments to document your program as you see fit. Include comments at the beginning of your
program with your name, the class (with section), and the date.
Sample Output](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd5d0662c-e3a2-4a4e-b96d-9b625dcca7d4%2F6eb0b6e7-60f2-421e-872f-8855955db0f5%2Fp3piccn_processed.png&w=3840&q=75)
![I have to write a program to calculate the value of the inventory in a warehouse.
Product Code Quantity on Hand Cost Each Total Value
123-A
467
1.99
412-S
998-W
005-Z
672-K
439-B
388
14115
8
44
24122
24.44
3.37
3. float cost[SIZE]
4. float value[SIZE]
447.12
7.28
0.09
The information from the first three columns exists a file called inventory.txt. Your program will calculate
the fourth column, then display the data on the screen.
Requirements
1. Read the input file inventory.txt.
2. Store the input data in parallel arrays, defined with a maximum capacity determined by the named
constant called SIZE, initialized with a value of 20.
1. string product[SIZE]
2. int quantity[SIZE]
3. Create these functions according to the prototypes.
1. void loadArrays(string[], int[], float[], int &);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd5d0662c-e3a2-4a4e-b96d-9b625dcca7d4%2F6eb0b6e7-60f2-421e-872f-8855955db0f5%2Fi0lm1ta_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 1 images

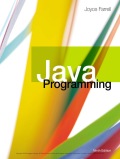
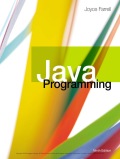