Here's the code. When I tried solving I kept getting an incompatible type error. If a solution could be provided it would be greatly appreciated. import java.util.Scanner; public class WhatOrder { // TODO: Define a generic method called checkOrder() that // takes in four variables of generic type as arguments. // The return type of the method is integer // Check the order of the input: return -1 for ascending, // 0 for neither, 1 for descending public static void main(String[] args) { Scanner scnr = new Scanner(System.in); // Check order of four strings System.out.println("Order: " + checkOrder(scnr.next(), scnr.next(), scnr.next(), scnr.next())); // Check order of four doubles System.out.println("Order: " + checkOrder(scnr.nextDouble(), scnr.nextDouble(), scnr.nextDouble(), scnr.nextDouble())); } }
Here's the code. When I tried solving I kept getting an incompatible type error. If a solution could be provided it would be greatly appreciated.
import java.util.Scanner;
public class WhatOrder {
// TODO: Define a generic method called checkOrder() that
// takes in four variables of generic type as arguments.
// The return type of the method is integer
// Check the order of the input: return -1 for ascending,
// 0 for neither, 1 for descending
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
// Check order of four strings
System.out.println("Order: " + checkOrder(scnr.next(), scnr.next(), scnr.next(), scnr.next()));
// Check order of four doubles
System.out.println("Order: " + checkOrder(scnr.nextDouble(), scnr.nextDouble(), scnr.nextDouble(), scnr.nextDouble()));
}
}
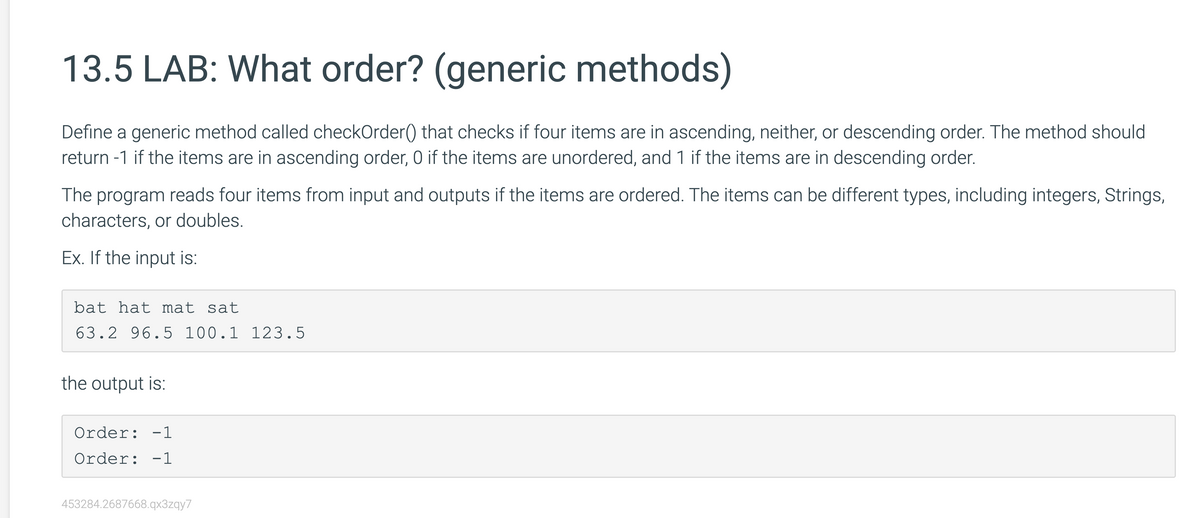

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

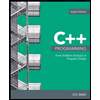
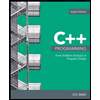