Design an interface named Encryptor that has a single (abstract) method named encrypt with one String argument named message. The method should return a String (which will be the encrypted message). Design a class named EncryptorOne that implements the Encryptor interface, and with one int instance variable named shift. The constructor should have one parameter to initialize the shift. Implement the method encrypt so that each letter of the message sent as the argument (with lowercase letters) is shifted by the value in shift. For example, if shift is 3, a will be replaced by d, b will be replaced by e, c will be replaced by f, and so on with a wraparound (i.e. with shift 3, x will be replaced by a, y will be replaced by b, and z will be replaced by c). The method should return the modified version of the message. (HINT: The value of a character literal is the integer value of the character in the Unicode character set. So, adding an integer (say, shift) to a character and type- casting the resulting number back to a character will give a chararcter that is at a distance shift from the original character. One another way is to use an array of alphabets.) Design a class named EncryptorTwo that implements the Encryptor interface. Implement the method encrypt so that the message sent as the argument is modified as follows: the first half of the letters that make the message should be swapped with the second half of the message. For example, if the message is boston, the modified message should be tonbos. If the message is houston, the modified message should be tonhous. The method should return the modified version of the message. Write a test program that creates an array of type Encryptor of size 3, and populates the the array with two EncryptorOne objects (with shift values input by the user) and one EncryptorTwo object. Then, using a loop, invoke the encrypt method of the objects (in the array) by passing a message input by the user and print the modified message returned by the method
java
Design an interface named Encryptor that has a single (abstract) method named encrypt with one
String argument named message. The method should return a String (which will be the encrypted
message).
Design a class named EncryptorOne that implements the Encryptor interface, and with one int
instance variable named shift. The constructor should have one parameter to initialize the shift.
Implement the method encrypt so that each letter of the message sent as the argument (with
lowercase letters) is shifted by the value in shift. For example, if shift is 3, a will be replaced by d, b
will be replaced by e, c will be replaced by f, and so on with a wraparound (i.e. with shift 3, x will be
replaced by a, y will be replaced by b, and z will be replaced by c). The method should return the
modified version of the message. (HINT: The value of a character literal is the integer value of the
character in the Unicode character set. So, adding an integer (say, shift) to a character and type-
casting the resulting number back to a character will give a chararcter that is at a distance shift from
the original character. One another way is to use an array of alphabets.)
Design a class named EncryptorTwo that implements the Encryptor interface. Implement the
method encrypt so that the message sent as the argument is modified as follows: the first half of the
letters that make the message should be swapped with the second half of the message. For
example, if the message is boston, the modified message should be tonbos. If the message is
houston, the modified message should be tonhous. The method should return the modified version
of the message.
Write a test program that creates an array of type Encryptor of size 3, and populates the the array
with two EncryptorOne objects (with shift values input by the user) and one EncryptorTwo object.
Then, using a loop, invoke the encrypt method of the objects (in the array) by passing a message
input by the user and print the modified message returned by the method.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Hey, so would i have to make three seperate java files labeled in order
EncryptorOne
EncryptorTwo
EncryptorMain
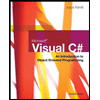
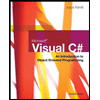