Hey, this is my code below and I cannot seem to get the import.java.io.*; to create and save information on a txt file. Any help would be great. There is a person class with get and set for firstname, lastname, etc. However I could not fit it in due to space. Code in bold is what I tried for the txt.
Hey, this is my code below and I cannot seem to get the import.java.io.*; to create and save information on a txt file. Any help would be great. There is a person class with get and set for firstname, lastname, etc. However I could not fit it in due to space. Code in bold is what I tried for the txt.
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Scanner;
import mainInterface.Interface;
public class StaffManager {
protected static ArrayList<Staff> Staffs = new ArrayList<Staff>();
public static void main(String[] args) throws FileNotFoundException{
staffManMenu();
//Call writeToFile
writeToFile();
}
// method to write the staff data to a file titled StaffFile.txt
public static void writeToFile() throws FileNotFoundException{
//create object
PrintWriter write = new PrintWriter(new File("StaffData.txt"));
for(Staff s : Staffs) {
write.println("First Name: " + s.getFName());
write.println("Last Name: " + s.getLName());
write.println("Date of Birth: " + s.getDOB());
write.println("University ID: " + s.getUnivID());
write.println("Department: " + s.getDept());
write.println("\n");
}
write.close();
System.out.println("Staff data write to file, StaffData.txt");
}
public static void staffManMenu() {
Scanner key = new Scanner(System.in);
while (true) {
System.out.println("Staff Menu:");
System.out.println("1 - Add\n" + "2 - Delete\n" + "3 - Edit\n" + "4 - Display\n" + "5 - Return to Main Menu");
int menuOption = key.nextInt();
if (menuOption == 1)
addStaff();
else if (menuOption == 2)
deleteStaff();
else if (menuOption == 3)
editStaff();
else if (menuOption == 4)
displayStaff();
else if (menuOption == 5)
break;
}
Interface.mainMenu();
}
public static void addStaff() {
Scanner key = new Scanner(System.in);
System.out.print("Staff's first name: ");
String fn = key.nextLine();
System.out.print("Staff's last name: ");
String ln = key.nextLine();
System.out.print("Staff's date of birth: ");
String dob = key.nextLine();
System.out.print("Staff's University ID: ");
String id = key.nextLine();
System.out.print("Staff's Department: ");
String dept = key.nextLine();
Staffs.add(new Staff(fn, ln, dob, id, dept));
System.out.println("You added " + fn + " " + ln + " to the Staffs list.");
}
public static void deleteStaff() {
Scanner key = new Scanner(System.in);
System.out.print("Enter the University ID of the Staff you would like to delete: ");
String id = key.nextLine();
int index = searchStaff(id);
if (index > -1) {
Staffs.remove(index);
System.out.println("You successfully deleted the Staff with the id " + id);
} else {
System.out.println("The Staff you are looking for does not exist.");
}
}
public static void editStaff() {
Scanner key = new Scanner(System.in);
System.out.print("Enter the University ID of the Staff you would like to edit: ");
String id = key.nextLine();
int index = searchStaff(id);
if (index > -1) {
Staffs.set(index, null);
System.out.println("Enter new Name : ");
String fn = key.nextLine();
System.out.println("Enter new Name : ");
String ln = key.nextLine();
System.out.println("Enter new Date of Birth : ");
String dob = key.nextLine();
System.out.println("Enter new ID Number : ");
String newID = key.nextLine();
System.out.println("Enter new Department : ");
String d = key.nextLine();
Staffs.set(index, new Staff(fn, ln, dob, newID, d));
}
}
public static void displayStaff() {
Scanner key = new Scanner(System.in);
System.out.println("Display Menu:\n" + "1 - Display all Staffs\n" + "2 - Display a given Staff");
int n = key.nextInt();
key.nextLine();
if (n == 1) {
for (Staff p : Staffs) {
System.out.println("First Name: " + p.getFName());
System.out.println("Last Name: " + p.getLName());
System.out.println("Date of Birth: " + p.getDOB());
System.out.println("University ID: " + p.getUnivID());
System.out.println("Department: " + p.getDept());
System.out.println("\n");
}
} else if (n == 2) {
System.out.print("Enter the University ID of the Staff you want to display: ");
String id = key.nextLine();
int index = searchStaff(id);
if (index > -1) {
Staff p = Staffs.get(index);
System.out.println("First Name: " + p.getFName());
System.out.println("Last Name: " + p.getLName());
System.out.println("Date of Birth: " + p.getDOB());
System.out.println("University ID: " + p.getUnivID());
System.out.println("Department: " + p.getDept());
System.out.println("\n");
}
}
}
public static int searchStaff(String id) {
for (int i = 0; i < Staffs.size(); i++) {
if (Staffs.get(i).getUnivID().equals(id))
return i;
}
return -1;
}
}
package Staff;
import mainInterface.Person;
public class Staff extends Person{
protected String dept;
public Staff(String f, String l, String dob, String id, String d) {
super(f, l, dob, id);
dept = d;
}
public void setDept(String d) {
dept = d;
}
public String getDept() {
return dept;
}
public int compareTo(Staff o) {
return this.univID.compareTo(o.univID);
}
}

Step by step
Solved in 4 steps with 2 images

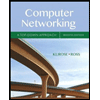
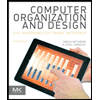
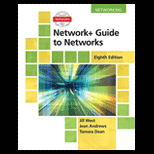
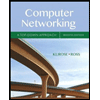
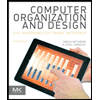
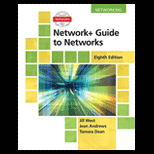
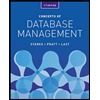
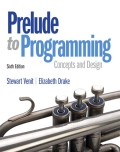
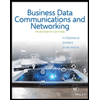