Hi! I've asked this multiple times but the code given by the expert is wrong. Below is the supposedly correct output, and my current one. Please fix, thank you! ### Template import random
Hi! I've asked this multiple times but the code given by the expert is wrong. Below is the supposedly correct output, and my current one. Please fix, thank you! ### Template import random
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hi! I've asked this multiple times but the code given by the expert is wrong. Below is the supposedly correct output, and my current one. Please fix, thank you!
### Template
import random
class Card:
def __init__(self, value, suite):
self.value = value
self.suite = suite
def __str__(self):
return f"{self.value} of {self.suite}"
def __eq__(self, other):
"""Check if two cards are the same"""
# -- YOUR CODE HERE --
return (self.value == other.value and self.suite == other.suite)
class CardSet:
def __init__(self):
self.cards = []
def view(self):
for card in self.cards:
print(card)
def add_cards(self, cards):
"""Add cards to your set"""
# -- YOUR CODE HERE --
for card in cards:
self.cards.append(card)
class Deck(CardSet):
def __init__(self):
"""Initialize the 52-card set. Start from 1-11, then Jack, Queen, King, then by suite: clubs, spades, hearts, diamonds"""
cards = []
# -- YOUR CODE HERE --
for suite in ("clubs", "spades", "hearts", "diamonds"):
for value in ("1", "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King"):
card = Card(value, suite)
cards.append(card)
self.cards = cards
def count_cards(self):
""""Count the number of cards in a deck"""
# -- YOUR CODE HERE --
return len(self.cards)
def shuffle(self, seed=None):
"""Shuffle your deck using a random seed"""
random.seed(seed)
# -- YOUR CODE HERE --
random.shuffle(self.cards)
def peek(self, number=5):
"""Show the top n cards of the stack. This is analogous to getting the last n cards then reversing it."""
# -- YOUR CODE HERE --
cnt = 0
if number > len(self.cards):
cnt = len(self.cards)
else:
cnt = number
for i in range(-5, -5+cnt):
print(self.cards[i])
def draw(self, cardset, number=5):
"""Transfer the top n cards of the stack to your cardset."""
# -- YOUR CODE HERE --
cards = []
cnt = 0
if number > len(self.cards):
cnt = len(self.cards)
else:
cnt = number
for i in range(-5, -5+cnt):
cards.append(self.cards[i])
self.cards.pop()
cards.reverse()
cardset.add_cards(cards)
def add_cards(self):
pass
if __name__ == "__main__":
seed, hand, peek = input().split(",")
myDeck = Deck()
handA = CardSet()
handB = CardSet()
myDeck.shuffle(int(seed))
for x in range(1,3):
print(f"\nRound {x}:")
myDeck.draw(handA, int(hand))
myDeck.draw(handB, int(hand))
print("Hand A: ")
handA.view()
print("Hand B: ")
handB.view()
myDeck.count_cards()
if(x == 1):
print(f"\n{peek} Cards at the top: ")
myDeck.peek(int(peek))
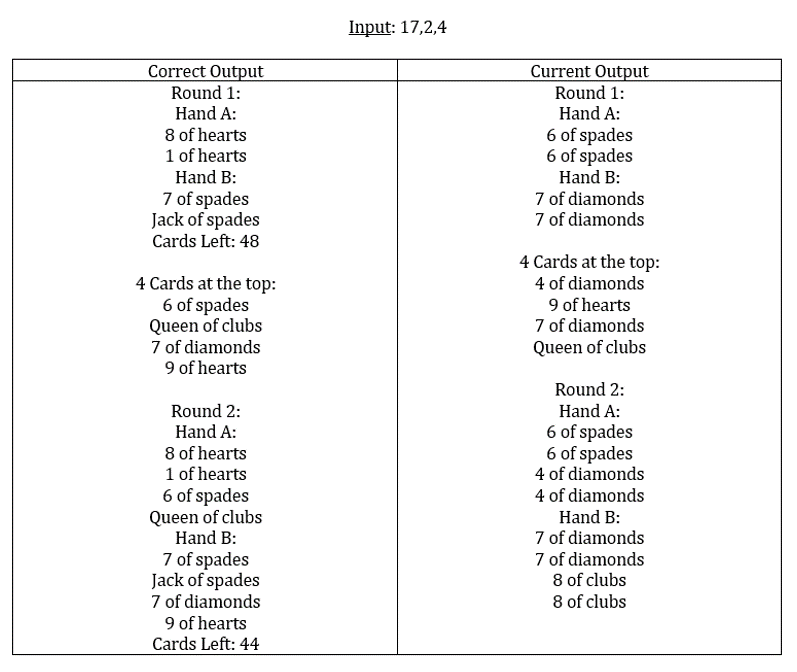
Transcribed Image Text:Input: 17,2,4
Correct Output
Round 1:
Hand A:
Current Output
Round 1:
Hand A:
6 of spades
6 of spades
Hand B:
8 of hearts
1 of hearts
Hand B:
7 of spades
Jack of spades
Cards Left: 48
7 of diamonds
7 of diamonds
4 Cards at the top:
4 of diamonds
4 Cards at the top:
6 of spades
Queen of clubs
7 of diamonds
9 of hearts
9 of hearts
7 of diamonds
Queen of clubs
Round 2:
Round 2:
Hand A:
6 of spades
6 of spades
4 of diamonds
Hand A:
8 of hearts
1 of hearts
6 of spades
Queen of clubs
Hand B:
7 of spades
Jack of spades
7 of diamonds
9 of hearts
Cards Left: 44
4 of diamonds
Hand B:
7 of diamonds
7 of diamonds
8 of clubs
8 of clubs
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
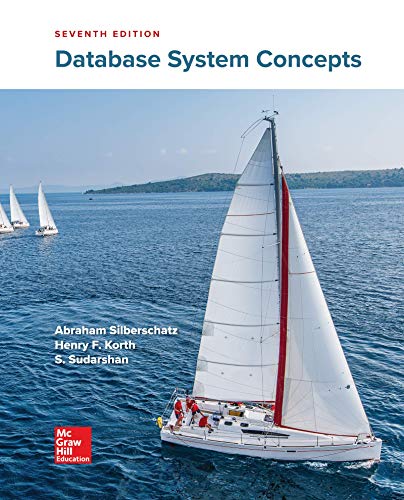
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
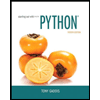
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
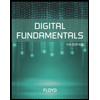
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
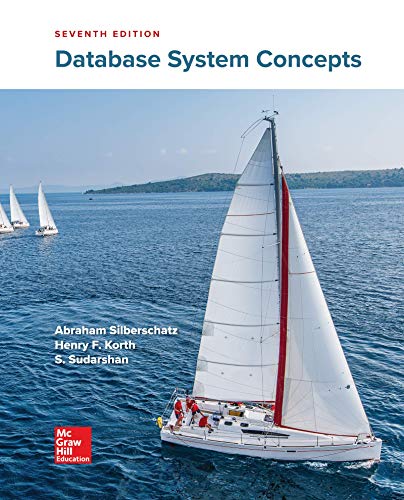
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
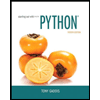
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
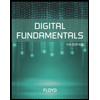
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
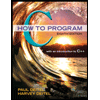
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
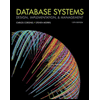
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
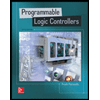
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education