Hi there dear expert, Here I have pasted the errors (max 2 allowed, but suffices hopefully), the assignment and my code. It seems the problem is in the function def grade() (and the weights).. it seems to work for all students though but one (error carried along across all functions). Hopefully you can help fix it? Thank you so much in advance. Kind regards Assignment: Make a class student (in student.py) that stores the following information for a student: Name (name) Student number (student_nr) Points per assignment (points_per_assignment) Exam grade (exam_grade) a) Behind each point of information is the name of the parameter to the initializer method. Store this information from the parameters in the object's attributes with the same name. b) Add a method course_points() which returns the number of course points the student has gotten. Example: mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9) print(mary.course_points()) > 121 The calculation of the course points is explained in the course overview, course setup slides (Links to an external site.) and the first lectureLinks to an external site.. c) Add a method grade() which returns a the final grade of the student, rounded to nearest half (upwards, 6.75 -> 7). As per regulations, a 5.5 becomes a 6. If the student did not pass both the assignments (>= 95 course points) and the exam (exam grade >= 5.5), then the grade is "NVD" (niet voldaan = not fulfilled). For example: mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9) print(mary.grade()) > 9.5 joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5) print(joe.grade()) > "NVD" d) Add a method result() which gives the final result of the student for the VU administration. The grade should always be displayed with one decimal number. For example: mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9) print(mary.result()) > Mary (15789613) has scored 9.5 on X_401096 joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5) print(joe.result()) > Joe (15789688) has scored NVD on X_401096 e) Add a method passes() that returns if a student passes the course. mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9) print(mary.passes()) > True joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5) print(joe.passes()) > False f) Implement the method a.scores_higher_than(b) that determines if student a scores higher than student b. A student scores higher than another student if their grade is higher (any grade is higher than "NVD"). If students a and b have the same grade, then neither does a score higher than b, nor the other way around. mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9) joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5) print(joe.scores_higher_than(mary)) > False g) Make a class Class (as in a class of students, not a class as in a class of objects) that stores several Student objects. Store a list of students in an attribute called students. The initializer method should initialize the class as an empty class (no students). Implement a method add_student() that takes an object of class Student and adds it to class. Implement method pass_rate() that determines the fraction of students that pass the class (have a grade of >= 6). My Code: class Student: def __init__(self, name, student_nr, points_per_assignment, exam_grade): self.name = name self.student_nr = student_nr self.points = points_per_assignment self.exam_grade = exam_grade def course_points(self): weight, total = [1, 2, 2, 2, 3, 3], 0 for i in range(len(weight)): total += weight[i] * self.points[i] return total def grade(self): #add divisor variable that counts total number of points num_points = len(self.points) average = sum(self.points) * 100 / num_points if average >= 95 and self.exam_grade >= 5.5: return (self.exam_grade + 0.5) else: return 'NVD' def result(self): return f'{self.name} ({self.student_nr}) has scored {self.grade()} on X_401096' def passes(self): #for self.name in Student: if self.grade() == 'NVD': return False return self.grade() >= 6 def scores_higher_than(self, b): if self.grade() != 'NVD' and b.grade() != 'NVD': return self.grade() > b.grade() return False class Class: def __init__(self): self.students = [] def add_student(self, student): self.students.append(student) def pass_rate(self): passed = 0 for student in self.students: student.result() if student.passes(): passed += 1 return passed / len(self.students) if __name__ == "__main__": # put test code here mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9) alice = Student("Alice", 1235233, [10, 9, 9, 9, 8, 7], 7) bob = Student("Bob", 1235232, [10, 9, 7, 9, 8, 9], 5) charlie = Student("Charlie", 13655232, [10, 7, 6, 7, 9, 6], 5.5) anne = Student("Anne", 12655232, [10, 9, 5, 10, 5, 5], 8) classroom = Class() classroom.add_student(mary) classroom.add_student(alice) classroom.add_student(bob) classroom.add_student(charlie) classroom.add_student(anne) #testing myself # print(anne.grade()) # print(mary.grade()) # print(alice.grade()) #print(bob.grade()) #print(charlie.grade())
Hi there dear expert,
Here I have pasted the errors (max 2 allowed, but suffices hopefully), the assignment and my code. It seems the problem is in the function def grade() (and the weights).. it seems to work for all students though but one (error carried along across all functions). Hopefully you can help fix it? Thank you so much in advance. Kind regards
Assignment:
Make a class student (in student.py) that stores the following information for a student:
- Name (name)
- Student number (student_nr)
- Points per assignment (points_per_assignment)
- Exam grade (exam_grade)
a) Behind each point of information is the name of the parameter to the initializer method. Store this information from the parameters in the object's attributes with the same name.
b) Add a method course_points() which returns the number of course points the student has gotten. Example:
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
print(mary.course_points())
> 121
The calculation of the course points is explained in the course overview, course setup slides (Links to an external site.) and the first lectureLinks to an external site..
c) Add a method grade() which returns a the final grade of the student, rounded to nearest half (upwards, 6.75 -> 7). As per regulations, a 5.5 becomes a 6. If the student did not pass both the assignments (>= 95 course points) and the exam (exam grade >= 5.5), then the grade is "NVD" (niet voldaan = not fulfilled). For example:
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
print(mary.grade())
> 9.5
joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)
print(joe.grade())
> "NVD"
d) Add a method result() which gives the final result of the student for the VU administration. The grade should always be displayed with one decimal number. For example:
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
print(mary.result())
> Mary (15789613) has scored 9.5 on X_401096
joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)
print(joe.result())
> Joe (15789688) has scored NVD on X_401096
e) Add a method passes() that returns if a student passes the course.
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
print(mary.passes())
> True
joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)
print(joe.passes())
> False
f) Implement the method a.scores_higher_than(b) that determines if student a scores higher than student b. A student scores higher than another student if their grade is higher (any grade is higher than "NVD"). If students a and b have the same grade, then neither does a score higher than b, nor the other way around.
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
joe = Student("Joe", 15789688, [10, 9, 8, 10, 5, 10], 5)
print(joe.scores_higher_than(mary))
> False
g) Make a class Class (as in a class of students, not a class as in a class of objects) that stores several Student objects. Store a list of students in an attribute called students. The initializer method should initialize the class as an empty class (no students). Implement a method add_student() that takes an object of class Student and adds it to class. Implement method pass_rate() that determines the fraction of students that pass the class (have a grade of >= 6).
My Code:
class Student:
def __init__(self, name, student_nr, points_per_assignment, exam_grade):
self.name = name
self.student_nr = student_nr
self.points = points_per_assignment
self.exam_grade = exam_grade
def course_points(self):
weight, total = [1, 2, 2, 2, 3, 3], 0
for i in range(len(weight)):
total += weight[i] * self.points[i]
return total
def grade(self):
#add divisor variable that counts total number of points
num_points = len(self.points)
average = sum(self.points) * 100 / num_points
if average >= 95 and self.exam_grade >= 5.5:
return (self.exam_grade + 0.5)
else:
return 'NVD'
def result(self):
return f'{self.name} ({self.student_nr}) has scored {self.grade()} on X_401096'
def passes(self):
#for self.name in Student:
if self.grade() == 'NVD':
return False
return self.grade() >= 6
def scores_higher_than(self, b):
if self.grade() != 'NVD' and b.grade() != 'NVD':
return self.grade() > b.grade()
return False
class Class:
def __init__(self):
self.students = []
def add_student(self, student):
self.students.append(student)
def pass_rate(self):
passed = 0
for student in self.students:
student.result()
if student.passes():
passed += 1
return passed / len(self.students)
if __name__ == "__main__":
# put test code here
mary = Student("Mary", 15789613, [10, 9, 8, 10, 9, 10], 9)
alice = Student("Alice", 1235233, [10, 9, 9, 9, 8, 7], 7)
bob = Student("Bob", 1235232, [10, 9, 7, 9, 8, 9], 5)
charlie = Student("Charlie", 13655232, [10, 7, 6, 7, 9, 6], 5.5)
anne = Student("Anne", 12655232, [10, 9, 5, 10, 5, 5], 8)
classroom = Class()
classroom.add_student(mary)
classroom.add_student(alice)
classroom.add_student(bob)
classroom.add_student(charlie)
classroom.add_student(anne)
#testing myself
# print(anne.grade())
# print(mary.grade())
# print(alice.grade())
#print(bob.grade())
#print(charlie.grade())

![tests_student. TestsStudent test class
tests_student.TestsStudent test_course_points
AssertionError: 0.8 != 0.6
1. self = <tests student.Testsstudent testMethod=test class>
tests_student. TestsStudent test_initializer
2.
AttributeError: 'Student' object has no attribute 'points_per_assignment'
3.
def test_class (self):
4.
students = [self.mary, self.alice, self.bob, self.charlie, self.anne]
1. self = <tests student.TestsStudent testMethod=test initializer>
5.
c - Class (O
2.
def test_initializer (self):
self.assertEqual (self.mary.name, "Mary")
self.assertEqual (self.mary.student_nr, 15789613)
self.assertEqual (self.mary.points_per_assignment, [10, 9, 8, 10, 9, 10])
6.
3.
for student in students :
c.add_student (student)
self.assertEqual (c.pass_rate(), 3/5)
7.
4.
8. >
5.
9. E
AssertionError: 0.8 != 0.6
6.
>
10.
7.
E
AttributeError: 'Student' object has no attribute 'points_per_assignment'
8.
11.
tests student.pv:84: AssertionError](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fea9f22ce-d122-4180-a6e5-3c667e608f3c%2Fdc1043de-a0a0-4755-b05b-42947d00b6d0%2Fkg1mdbf_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 2 images

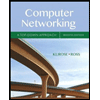
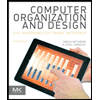
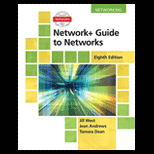
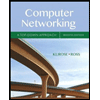
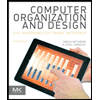
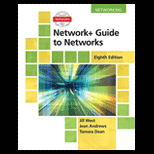
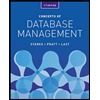
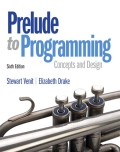
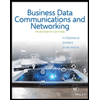