Hi, I am implementing a matrox with several functions. I have pasted the error, the assignment and my code. Hopefully you can see what causes the error and how to fix it. Thank you so much. My transpose function gives an error: IndexError: list assignment index out of range self = def test_transpose(self): self.assertEqual(str(self.a.transpose()), ' 1 4 7\n 2 5 8\n 3 6 9\n') self.assertEqual(str(self.b.transpose()), ' 2 8 14\n 4 10 16\n 6 12 18\n') self.assertEqual(str(self.c.transpose()), ' 1 0 0\n 0 1 0\n 0 0 1\n') self.assertEqual(str(self.a.transpose().transpose()), ' 1 2 3\n 4 5 6\n 7 8 9\n') self.assertEqual(str(self.e.transpose()), ' 0 0 0 1\n 0 0 1 0\n 0 1 0 0\n 1 0 0 0\n') > self.assertEqual(str(self.f.transpose()), ' 1 2 3 1\n 2 3 2 3\n 3 1 1 2\n') tests_matrix.py:34: _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ self = 1 2 3 2 3 1 3 2 1 1 3 2 def transpose(self): nl = [[None for j in range(len(self.list1[i]))] for i in range(len(self.list1))] for i in range(len(self.list1)): # or remove -1 for j in range(len(self.list1[i])): # or remove -1 #nl[j].append(self.list1[i][j]) > nl[j][i] = self.list1[i][j] E IndexError: list assignment index out of range Below I have pasted the assignment and my code. here is the assignment: Implement a matrix class (in matrix.py). a) The initializer should take a list of lists as an argument, where each outer list is a row, and each value in an inner list is a value in the corresponding row. b) Implement the __str__ method to nicely format the string representation of the matrix: one line per row, two characters per number (%2d) and a space between numbers. For example: m = Matrix([[1,0,0],[0,1,0],[0,0,1]]) print(m) > 1 0 0 > 0 1 0 > 0 0 1 c) Implement a method scale(factor) that returns a new matrix where each value is multiplied by scale. For example: m = Matrix([[1,2,3],[4,5,6],[7,8,9]]) n = m.scale(2) print(n)> 2 4 6 > 8 10 12 >14 16 18 print(m) > 1 2 3 > 4 5 6 > 7 8 9 d) Implement a method transpose() that returns a new matrix that has been transposed. Transposing flips a matrix over its diagonal: it switches rows and columns. m = Matrix([[1,2,3],[4,5,6],[7,8,9]]) print(m) > 1 2 3 > 4 5 6 > 7 8 9 print(m.transpose()) > 1 4 7 > 2 5 8 > 3 6 9 My code: class Matrix: def __init__(self, rows): self.list1 = rows def __str__(self): res = "" for i in range(len(self.list1)): for j in range(len(self.list1[i])): res = res + "{:2d}".format(self.list1[i][j]) if j < len(self.list1[i]) - 1: res += " " res = res + "\n" return res def scale(self, w): nl = [] for i in range(len(self.list1)): nl.append([]) for j in range(len(self.list1[i])): nl[i].append(self.list1[i][j] * w) return Matrix(nl) def transpose(self): nl = [[None for j in range(len(self.list1[i]))] for i in range(len(self.list1))] for i in range(len(self.list1)): # or remove -1 for j in range(len(self.list1[i])): # or remove -1 #nl[j].append(self.list1[i][j]) nl[j][i] = self.list1[i][j] return Matrix(nl) #return nl def multiply(self, b): nl = [[0 for j in range(len(b.list1[i]))] for i in range(len(self.list1))] if len(self.list1[0]) == len(b.list1): for i in range(len(self.list1)): for j in range(len(b.list1[0])): for k in range(len(b.list1)): nl[i][j] += self.list1[i][k] * b.list1[k][j] return Matrix(nl) else: return None # repr is similar to __str__, but __str__ is used when # printing a value, __repr__ when showing a value in # the interpreter. # repr is intended to be an unambiguous, complete representation # of the object, while str is intended to be nicely readable # for this object they are the same def __repr__(self): return str(self) if __name__ == "__main__": m = Matrix([[1, 0, 0], [0, 1, 0], [0, 0, 1]]) m = Matrix([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) n = m.scale(2) print(n) print(m.transpose()) e = Matrix([[1, 2, 3], [2, 1, 3], [3, 2, 1]]) print(e.multiply(e)) print(e.__repr__())
Hi, I am implementing a matrox with several functions. I have pasted the error, the assignment and my code. Hopefully you can see what causes the error and how to fix it. Thank you so much.
My transpose function gives an error:
IndexError: list assignment index out of range
- self = <tests_matrix.TestsStudent testMethod=test_transpose>
- def test_transpose(self):
- self.assertEqual(str(self.a.transpose()), ' 1 4 7\n 2 5 8\n 3 6 9\n')
- self.assertEqual(str(self.b.transpose()), ' 2 8 14\n 4 10 16\n 6 12 18\n')
- self.assertEqual(str(self.c.transpose()), ' 1 0 0\n 0 1 0\n 0 0 1\n')
- self.assertEqual(str(self.a.transpose().transpose()), ' 1 2 3\n 4 5 6\n 7 8 9\n')
- self.assertEqual(str(self.e.transpose()), ' 0 0 0 1\n 0 0 1 0\n 0 1 0 0\n 1 0 0 0\n')
- > self.assertEqual(str(self.f.transpose()), ' 1 2 3 1\n 2 3 2 3\n 3 1 1 2\n')
- tests_matrix.py:34:
- _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _
- self = 1 2 3
- 2 3 1
- 3 2 1
- 1 3 2
- def transpose(self):
- nl = [[None for j in range(len(self.list1[i]))] for i in range(len(self.list1))]
- for i in range(len(self.list1)): # or remove -1
- for j in range(len(self.list1[i])): # or remove -1
- #nl[j].append(self.list1[i][j])
- > nl[j][i] = self.list1[i][j]
- E IndexError: list assignment index out of range
Below I have pasted the assignment and my code. here is the assignment:
Implement a matrix class (in matrix.py).
a) The initializer should take a list of lists as an argument, where each outer list is a row, and each value in an inner list is a value in the corresponding row.
b) Implement the __str__ method to nicely format the string representation of the matrix: one line per row, two characters per number (%2d) and a space between numbers. For example:
m = Matrix([[1,0,0],[0,1,0],[0,0,1]])
print(m)
> 1 0 0
> 0 1 0
> 0 0 1
c) Implement a method scale(factor) that returns a new matrix where each value is multiplied by scale. For example:
m = Matrix([[1,2,3],[4,5,6],[7,8,9]])
n = m.scale(2)
print(n)> 2 4 6
> 8 10 12
>14 16 18
print(m)
> 1 2 3
> 4 5 6
> 7 8 9
d) Implement a method transpose() that returns a new matrix that has been transposed. Transposing flips a matrix over its diagonal: it switches rows and columns.
m = Matrix([[1,2,3],[4,5,6],[7,8,9]])
print(m)
> 1 2 3
> 4 5 6
> 7 8 9
print(m.transpose())
> 1 4 7
> 2 5 8
> 3 6 9
My code:
class Matrix:
def __init__(self, rows):
self.list1 = rows
def __str__(self):
res = ""
for i in range(len(self.list1)):
for j in range(len(self.list1[i])):
res = res + "{:2d}".format(self.list1[i][j])
if j < len(self.list1[i]) - 1:
res += " "
res = res + "\n"
return res
def scale(self, w):
nl = []
for i in range(len(self.list1)):
nl.append([])
for j in range(len(self.list1[i])):
nl[i].append(self.list1[i][j] * w)
return Matrix(nl)
def transpose(self):
nl = [[None for j in range(len(self.list1[i]))] for i in range(len(self.list1))]
for i in range(len(self.list1)): # or remove -1
for j in range(len(self.list1[i])): # or remove -1
#nl[j].append(self.list1[i][j])
nl[j][i] = self.list1[i][j]
return Matrix(nl)
#return nl
def multiply(self, b):
nl = [[0 for j in range(len(b.list1[i]))] for i in range(len(self.list1))]
if len(self.list1[0]) == len(b.list1):
for i in range(len(self.list1)):
for j in range(len(b.list1[0])):
for k in range(len(b.list1)):
nl[i][j] += self.list1[i][k] * b.list1[k][j]
return Matrix(nl)
else:
return None
# repr is similar to __str__, but __str__ is used when
# printing a value, __repr__ when showing a value in
# the interpreter.
# repr is intended to be an unambiguous, complete representation
# of the object, while str is intended to be nicely readable
# for this object they are the same
def __repr__(self):
return str(self)
if __name__ == "__main__":
m = Matrix([[1, 0, 0], [0, 1, 0], [0, 0, 1]])
m = Matrix([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
n = m.scale(2)
print(n)
print(m.transpose())
e = Matrix([[1, 2, 3], [2, 1, 3], [3, 2, 1]])
print(e.multiply(e))
print(e.__repr__())

Step by step
Solved in 2 steps with 1 images

Hi there,
You mention what should be changed in a comment in the function multiply right? but you dint cgange it in the code, right? (i see no difference). besides, my error was in the transpose function. The multiply function actually passed the tests. Do you see any errors in the transpose function? Thanks
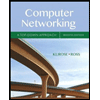
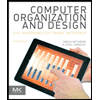
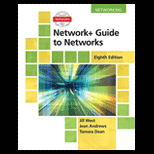
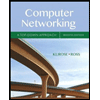
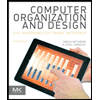
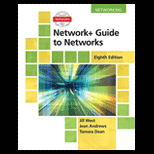
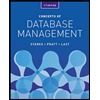
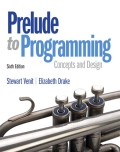
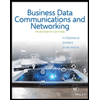