How can I convert this sequential C++ program to a parallel C++ program using OpenMP. What is the speedup? I will rate! #include #include using namespace std; #ifndef N #define N 5 #endif #ifndef FS #define FS 38 #endif struct node { int data; int fibdata; struct node* next; }; int fib(int n) { int x, y; if (n < 2) { return (n); } else { x = fib(n - 1); y = fib(n - 2); return (x + y); } } void processwork(struct node* p) { int n; n = p->data; p->fibdata = fib(n); } struct node* init_list(struct node* p) { int i; struct node* head = NULL; struct node* temp = NULL; head = new node;
How can I convert this sequential C++ program to a parallel C++ program using OpenMP. What is the speedup? I will rate! #include #include using namespace std; #ifndef N #define N 5 #endif #ifndef FS #define FS 38 #endif struct node { int data; int fibdata; struct node* next; }; int fib(int n) { int x, y; if (n < 2) { return (n); } else { x = fib(n - 1); y = fib(n - 2); return (x + y); } } void processwork(struct node* p) { int n; n = p->data; p->fibdata = fib(n); } struct node* init_list(struct node* p) { int i; struct node* head = NULL; struct node* temp = NULL; head = new node;
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
How can I convert this sequential C++ program to a parallel C++ program using OpenMP.
What is the speedup? I will rate!
#include <iostream>
#include <omp.h>
using namespace std;
#ifndef N
#define N 5
#endif
#ifndef FS
#define FS 38
#endif
struct node {
int data;
int fibdata;
struct node* next;
};
int fib(int n) {
int x, y;
if (n < 2) {
return (n);
} else {
x = fib(n - 1);
y = fib(n - 2);
return (x + y);
}
}
void processwork(struct node* p)
{
int n;
n = p->data;
p->fibdata = fib(n);
}
struct node* init_list(struct node* p) {
int i;
struct node* head = NULL;
struct node* temp = NULL;
head = new node;
p = head;
p->data = FS;
p->fibdata = 0;
for (i=0; i< N; i++) {
temp = new node;
p->next = temp;
p = temp;
p->data = FS + i + 1;
p->fibdata = i+1;
}
p->next = NULL;
return head;
}
int main(int argc, char *argv[]) {
double start, end;
struct node *p=NULL;
struct node *temp=NULL;
struct node *head=NULL;
printf("Process linked list\n");
printf(" Each linked list node will be processed by function 'processwork()'\n");
printf(" Each ll node will compute %d fibonacci numbers beginning with %d\n",N,FS);
p = init_list(p);
head = p;
start = omp_get_wtime();
{
while (p != NULL) {
processwork(p);
p = p->next;
}
}
end = omp_get_wtime();
p = head;
while (p != NULL) {
printf("%d : %d\n",p->data, p->fibdata);
temp = p->next;
delete p;
p = temp;
}
delete p;
printf("Compute Time: %f seconds\n", end - start);
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
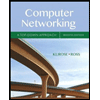
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
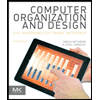
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
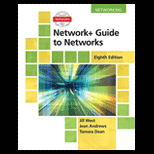
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
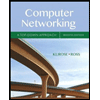
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
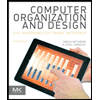
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
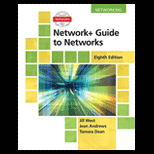
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
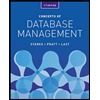
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
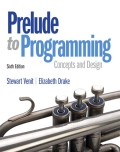
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
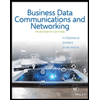
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY