How would I add a TextWatcher to the following Java calculator app code: package com.example.tipcalculatorv2; import androidx.appcompat.app.AppCompatActivity; import android.content.Context; import android.os.Bundle; import android.view.View; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private EditText billEditText; private TextView totalTextView; private EditText tipPercentEditText; private TextView tipTextView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); billEditText = (EditText) findViewById(R.id.amount_bill); totalTextView = (TextView) findViewById(R.id.amount_total); tipPercentEditText = (EditText) findViewById(R.id.amount_tip_percent); tipTextView = (TextView) findViewById(R.id.amount_tip); } public void calculate(View view) { String billStr=billEditText.getText().toString(); totalTextView.setText(billStr); String tipPercentStr=tipPercentEditText.getText().toString(); tipTextView.setText(billStr); try { float billAmount = Float.parseFloat(billStr); float tipPercent = Float.parseFloat(tipPercentStr); float tip = billAmount * tipPercent / 100; tipTextView.setText(Float.toString(tip)); float total = billAmount + tip; totalTextView.setText(Float.toString(total)); } catch (NumberFormatException e) { Context context = getApplicationContext(); CharSequence text = "Please enter numbers for bill and tip"; int duration = Toast.LENGTH_SHORT; Toast toast = Toast.makeText(context, text, duration); toast.show(); e.printStackTrace(); } } }
How would I add a TextWatcher to the following Java calculator app code: package com.example.tipcalculatorv2; import androidx.appcompat.app.AppCompatActivity; import android.content.Context; import android.os.Bundle; import android.view.View; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private EditText billEditText; private TextView totalTextView; private EditText tipPercentEditText; private TextView tipTextView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); billEditText = (EditText) findViewById(R.id.amount_bill); totalTextView = (TextView) findViewById(R.id.amount_total); tipPercentEditText = (EditText) findViewById(R.id.amount_tip_percent); tipTextView = (TextView) findViewById(R.id.amount_tip); } public void calculate(View view) { String billStr=billEditText.getText().toString(); totalTextView.setText(billStr); String tipPercentStr=tipPercentEditText.getText().toString(); tipTextView.setText(billStr); try { float billAmount = Float.parseFloat(billStr); float tipPercent = Float.parseFloat(tipPercentStr); float tip = billAmount * tipPercent / 100; tipTextView.setText(Float.toString(tip)); float total = billAmount + tip; totalTextView.setText(Float.toString(total)); } catch (NumberFormatException e) { Context context = getApplicationContext(); CharSequence text = "Please enter numbers for bill and tip"; int duration = Toast.LENGTH_SHORT; Toast toast = Toast.makeText(context, text, duration); toast.show(); e.printStackTrace(); } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How would I add a TextWatcher to the following Java calculator app code:
package com.example.tipcalculatorv2;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private EditText billEditText;
private TextView totalTextView;
private EditText tipPercentEditText;
private TextView tipTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
billEditText = (EditText) findViewById(R.id.amount_bill);
totalTextView = (TextView) findViewById(R.id.amount_total);
tipPercentEditText = (EditText) findViewById(R.id.amount_tip_percent);
tipTextView = (TextView) findViewById(R.id.amount_tip);
}
public void calculate(View view) {
String billStr=billEditText.getText().toString();
totalTextView.setText(billStr);
String tipPercentStr=tipPercentEditText.getText().toString();
tipTextView.setText(billStr);
try {
float billAmount = Float.parseFloat(billStr);
float tipPercent = Float.parseFloat(tipPercentStr);
float tip = billAmount * tipPercent / 100;
tipTextView.setText(Float.toString(tip));
float total = billAmount + tip;
totalTextView.setText(Float.toString(total));
} catch (NumberFormatException e) {
Context context = getApplicationContext();
CharSequence text = "Please enter numbers for bill and tip";
int duration = Toast.LENGTH_SHORT;
Toast toast = Toast.makeText(context, text, duration);
toast.show();
e.printStackTrace();
}
}
}
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private EditText billEditText;
private TextView totalTextView;
private EditText tipPercentEditText;
private TextView tipTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
billEditText = (EditText) findViewById(R.id.amount_bill);
totalTextView = (TextView) findViewById(R.id.amount_total);
tipPercentEditText = (EditText) findViewById(R.id.amount_tip_percent);
tipTextView = (TextView) findViewById(R.id.amount_tip);
}
public void calculate(View view) {
String billStr=billEditText.getText().toString();
totalTextView.setText(billStr);
String tipPercentStr=tipPercentEditText.getText().toString();
tipTextView.setText(billStr);
try {
float billAmount = Float.parseFloat(billStr);
float tipPercent = Float.parseFloat(tipPercentStr);
float tip = billAmount * tipPercent / 100;
tipTextView.setText(Float.toString(tip));
float total = billAmount + tip;
totalTextView.setText(Float.toString(total));
} catch (NumberFormatException e) {
Context context = getApplicationContext();
CharSequence text = "Please enter numbers for bill and tip";
int duration = Toast.LENGTH_SHORT;
Toast toast = Toast.makeText(context, text, duration);
toast.show();
e.printStackTrace();
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
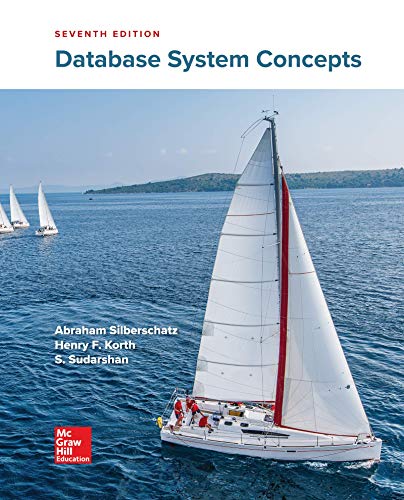
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
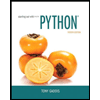
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
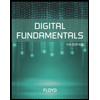
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
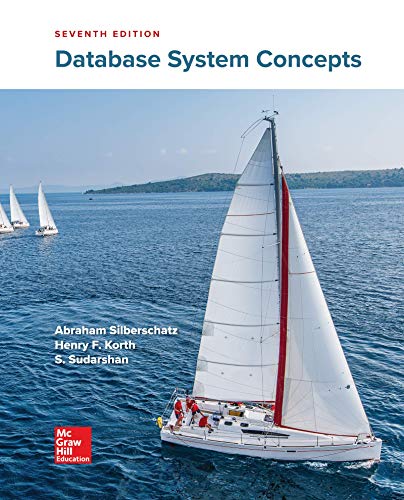
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
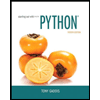
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
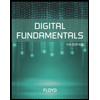
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
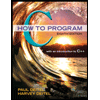
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
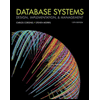
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
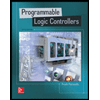
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education