I am getting a error at line 73 and 75 , can you please review the code ? //get text fields Line 73 - doubleinvestmentAmount = Double.parseDouble(investmentAmount.getTest()); Line 75 - int years = Integer.parseInt(years.getText()); package chapter15; import javafx.application.Application; import javafx.geometry.HPos; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.layout.GridPane; import javafx.scene.control.Button; import javafx.geometry.Insets; //15.5 Create an investment calculator using Text Boxes //program calculates the furtureValue at a given interest rate for a specified number of years // futureValue = investmentAmount * (1 + monthlyIntrestRate)^years*12 //Display the future amount in the test field when the users clicks the Calculate button import javafx.stage.Stage; //Steps to create a user interface program //1. Create a user interface // A. gridPane, add Lables, text fields, and button to pane public class InvestmentCalculator extends Application{ private TextField futureValue = new TextField ("00.00"); private TextField investmentAmount = new TextField ("0"); private TextField interestRate = new TextField ("0.00"); private TextField years = new TextField ("$"); private Button calculateButton = new Button("Calculate"); // futureValue.setEditable(false); @Override publicvoid start (Stage primaryStage)throws Exception {//Creeate the UI //Create an GridPane GridPane pane = new GridPane(); pane.setAlignment(Pos.BASELINE_LEFT); //Set H and V gap can lay out its children in a single horizontal and veritcal column. pane.setHgap(5);// creates space ablove and below pane.setVgap(5);//creates space on the sides // Label ("String value) , column, row ; pane.add(new Label("Investment Amount:"),0,0); pane.add(investmentAmount,1,0); pane.add(new Label("Number of Years:"),0,1); pane.add(years,1,1); pane.add(new Label("Interest Rate:"),0,2); pane.add(interestRate,1,2); pane.add(new Label("Future Value:"),0,3); pane.add(futureValue,1,3); pane.add(calculateButton, 1, 4); // B. Set the alignment of the button to the right pane.setAlignment(Pos.CENTER); investmentAmount.setAlignment(Pos.BOTTOM_RIGHT); years.setAlignment(Pos.BOTTOM_RIGHT); futureValue.setAlignment(Pos.BOTTOM_RIGHT); interestRate.setAlignment(Pos.BOTTOM_RIGHT); GridPane.setHalignment(calculateButton, HPos.RIGHT); //2. Process the Event // Process events on the calculate Button calculateButton.setOnAction(e -> futureValue()); Scene scene = new Scene(pane,350,300); primaryStage.setTitle("Investment Calculator"); //set the title primaryStage.setScene(scene); primaryStage.show(); }//Calculate the futureamount //get the vaules from the test fields and calculate the futureValue privatevoid futureValue() { //get testfilds values doubleinvestmentAmount = Double.parseDouble(investmentAmount.getTest()); intyears = Integer.parseInt(years.getText()); doublemonthlyInterestRate = Double.parseDouble(interestRate.getText()) / 1200; futureValue.setText(String.format("$%.2f", (investmentAmount * Math.pow(1 + monthlyInterestRate, years * 12)))); } publicstaticvoid main(String[]args) { launch(args); } }
I am getting a error at line 73 and 75 , can you please review the code ?
//get text fields
Line 73 - doubleinvestmentAmount = Double.parseDouble(investmentAmount.getTest());
Line 75 - int years = Integer.parseInt(years.getText());
package chapter15;
import javafx.application.Application;
import javafx.geometry.HPos;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
import javafx.scene.control.Button;
import javafx.geometry.Insets;
//15.5 Create an investment calculator using Text Boxes
//program calculates the furtureValue at a given interest rate for a specified number of years
// futureValue = investmentAmount * (1 + monthlyIntrestRate)^years*12
//Display the future amount in the test field when the users clicks the Calculate button
import javafx.stage.Stage;
//Steps to create a user interface program
//1. Create a user interface
// A. gridPane, add Lables, text fields, and button to pane
public class InvestmentCalculator extends Application{
private TextField futureValue = new TextField ("00.00");
private TextField investmentAmount = new TextField ("0");
private TextField interestRate = new TextField ("0.00");
private TextField years = new TextField ("$");
private Button calculateButton = new Button("Calculate");
// futureValue.setEditable(false);
@Override
publicvoid start (Stage primaryStage)throws Exception
{//Creeate the UI
//Create an GridPane
GridPane pane = new GridPane();
pane.setAlignment(Pos.BASELINE_LEFT);
//Set H and V gap can lay out its children in a single horizontal and veritcal column.
pane.setHgap(5);// creates space ablove and below
pane.setVgap(5);//creates space on the sides
// Label ("String value) , column, row ;
pane.add(new Label("Investment Amount:"),0,0);
pane.add(investmentAmount,1,0);
pane.add(new Label("Number of Years:"),0,1);
pane.add(years,1,1);
pane.add(new Label("Interest Rate:"),0,2);
pane.add(interestRate,1,2);
pane.add(new Label("Future Value:"),0,3);
pane.add(futureValue,1,3);
pane.add(calculateButton, 1, 4);
// B. Set the alignment of the button to the right
pane.setAlignment(Pos.CENTER);
investmentAmount.setAlignment(Pos.BOTTOM_RIGHT);
years.setAlignment(Pos.BOTTOM_RIGHT);
futureValue.setAlignment(Pos.BOTTOM_RIGHT);
interestRate.setAlignment(Pos.BOTTOM_RIGHT);
GridPane.setHalignment(calculateButton, HPos.RIGHT);
//2. Process the Event
// Process events on the calculate Button
calculateButton.setOnAction(e -> futureValue());
Scene scene = new Scene(pane,350,300);
primaryStage.setTitle("Investment Calculator"); //set the title
primaryStage.setScene(scene);
primaryStage.show();
}//Calculate the futureamount
//get the vaules from the test fields and calculate the futureValue
privatevoid futureValue() {
//get testfilds values
doubleinvestmentAmount = Double.parseDouble(investmentAmount.getTest());
intyears = Integer.parseInt(years.getText());
doublemonthlyInterestRate = Double.parseDouble(interestRate.getText()) / 1200;
futureValue.setText(String.format("$%.2f",
(investmentAmount * Math.pow(1 + monthlyInterestRate, years * 12))));
}
publicstaticvoid main(String[]args) {
launch(args);
}
}
![3
B
JavaFxShapes.java
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
710
72
73
74
075
76
77
78
79
80
810
82
83
84 }
85
86
87
88
89
moveTheBall.java
*InvestmentCalculator.java XEmoji.java
futureValue.setAlignment
interestRate.setAlignment
(Pos. BOTTOM RIGHT);
(Pos. BOTTOM_RIGHT) ;
GridPane.setHalignment (calculateButton, HPos.RIGHT) ;
//2. Process the Event
// Process events on the calculate Button
calculateButton.setOnAction (e -> futureValue () );
}
Scene scene = new Scene (pane, 350, 300);
primaryStage.setTitle ("Investment Calculator"); //set the title
primaryStage.setScene (scene);
primaryStage.show();
}//Calculate the futureamount
//get the vaules from the test fields and calculate the futureValue
private void futureValue () {
//get testfilds values
double investment Amount = Double.parse Double (investment Amount.getTest ();
}
int years = Integer.parseInt(years.getText());
double monthlyInterestRate = Double.parse Double (interestRate.getText()) / 1200;
futureValue.setText (String.format("$%.2f",
(investment Amount * Math.pow (1 + monthlyInterestRate, years * 12))));
public static void main(String[] args) {
launch (args);
■
I
Ir](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb285d47c-f9b3-4470-95ff-68106571d4da%2F8a492d9a-8340-480b-af7f-bdf1d24e85f5%2F8zofl3kw_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps

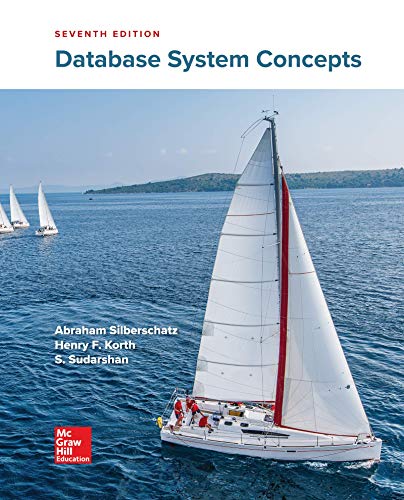
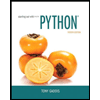
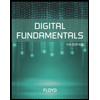
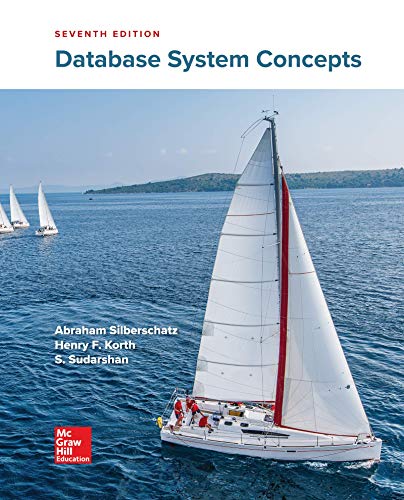
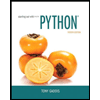
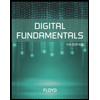
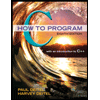
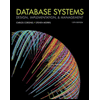
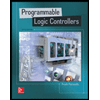