I am having trouble with this: Implement the convertString method based on its specification. Here are some things that can help: the Character class has some utility methods to determine if a character is uppercase, lowercase, or a digit (numeral) the String class has a method to get the character at a specific position (called the index). Find that method and use it. make use of the convert methods you already have! convertString Specification: /** * Converts the letters and numerals in the given string * to their circled counterparts. All other characters * are unchanged. * * @precondition text != null * @postcondition none * * @param text the text to convert * @return a modified version of text, with all letters and numerals * circled. */ My code: public class CircleStrings { /** * Converts the letters and numerals in the given string * to their circled counterparts. All other characters * are unchanged. * * @precondition text != null * @postcondition none * * @param text the text to convert * @return a modified version of text, with all letters and numerals * circled. */ public static String convertString(String text) { // replace the return statement with your solution return ""; } /** * Converts an uppercase letter to its circled counterpart. * * @precondition 'A' <= letter <= 'Z' * @postcondition none * * @param letter the uppercase letter to convert * @return the circled version of the uppercase letter */ public static char convertUppercaseLetter(char letter) { if (letter < 'A' || letter > 'Z') { throw new IllegalArgumentException("letter must be uppercase"); } return (char)(letter + 9333); } /** * Converts a lowercase letter to its circled counterpart. * * @precondition 'a' <= letter <= 'a' * @postcondition none * * @param letter the lowercase letter to convert * @return the circled version of the lowercase letter */ public static char convertLowercaseLetter(char letter) { if (letter < 'a' || letter > 'z') { throw new IllegalArgumentException("letter must be lowercase"); } return (char)(letter + 9327); } /** * Converts a numeral character to its circled counterpart. * * @precondition '0' <= letter <= '9' * @postcondition none * * @param numeral the numeral to convert * @return the circled version of the numeral */ public static char convertNumeral(char numeral) { if (numeral < '0' || numeral > '9') { throw new IllegalArgumentException("numeral must be between '0' and '9' inclusive"); } if (numeral == '0') { return (char)(numeral + 9402); } return (char)(numeral + 9263); } }
I am having trouble with this:
- Implement the convertString method based on its specification. Here are some things that can help:
- the Character class has some utility methods to determine if a character is uppercase, lowercase, or a digit (numeral)
- the String class has a method to get the character at a specific position (called the index). Find that method and use it.
- make use of the convert methods you already have!
convertString Specification:
/**
* Converts the letters and numerals in the given string
* to their circled counterparts. All other characters
* are unchanged.
*
* @precondition text != null
* @postcondition none
*
* @param text the text to convert
* @return a modified version of text, with all letters and numerals
* circled.
*/
My code:
public class CircleStrings {
/**
* Converts the letters and numerals in the given string
* to their circled counterparts. All other characters
* are unchanged.
*
* @precondition text != null
* @postcondition none
*
* @param text the text to convert
* @return a modified version of text, with all letters and numerals
* circled.
*/
public static String convertString(String text) {
// replace the return statement with your solution
return "";
}
/**
* Converts an uppercase letter to its circled counterpart.
*
* @precondition 'A' <= letter <= 'Z'
* @postcondition none
*
* @param letter the uppercase letter to convert
* @return the circled version of the uppercase letter
*/
public static char convertUppercaseLetter(char letter) {
if (letter < 'A' || letter > 'Z') {
throw new IllegalArgumentException("letter must be uppercase");
}
return (char)(letter + 9333);
}
/**
* Converts a lowercase letter to its circled counterpart.
*
* @precondition 'a' <= letter <= 'a'
* @postcondition none
*
* @param letter the lowercase letter to convert
* @return the circled version of the lowercase letter
*/
public static char convertLowercaseLetter(char letter) {
if (letter < 'a' || letter > 'z') {
throw new IllegalArgumentException("letter must be lowercase");
}
return (char)(letter + 9327);
}
/**
* Converts a numeral character to its circled counterpart.
*
* @precondition '0' <= letter <= '9'
* @postcondition none
*
* @param numeral the numeral to convert
* @return the circled version of the numeral
*/
public static char convertNumeral(char numeral) {
if (numeral < '0' || numeral > '9') {
throw new IllegalArgumentException("numeral must be between '0' and '9' inclusive");
}
if (numeral == '0') {
return (char)(numeral + 9402);
}
return (char)(numeral + 9263);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

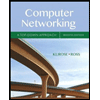
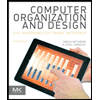
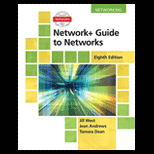
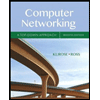
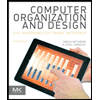
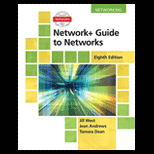
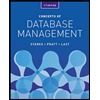
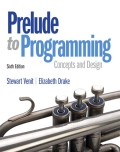
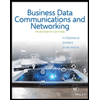