I am working on a code for loops where I need to list all the prime numbers between value A(number person enters lets say 101) and value b(the other number a person enters 200) and I am totally lost. here is what I have so far. import java.util.Scanner; public class PrimeNumber { public static void main(String[] args) { Scanner input = new Scanner(System.in); final int NUMBER_OF_PRIMES = 21; final int NUMBER_OF_PRIMES_PER_LINE = 5; int count = 0 ; int number = 2; System.out.println("enter a number value for a: "); int nbr1 = input.nextInt(); System.out.println("Enter a number for b: "); int nbr2= input.nextInt(); // Repeatedly find prime numbers while (count < NUMBER_OF_PRIMES) { boolean isPrime = true; for (int divisor = 2; divisor <= number / 2; divisor++) if (number % divisor == 0) { isPrime = false; break; } if (isPrime) { count++; if (count % NUMBER_OF_PRIMES_PER_LINE == 0) { System.out.println(number); } else { System.out.print(number + " "); } } number++; } // End while } } // End main } I want to get the prime numbers between 101 and 200 but I keep getting the prime between 2 and 73 Output: 2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73
I am working on a code for loops where I need to list all the prime numbers between value A(number person enters lets say 101) and value b(the other number a person enters 200) and I am totally lost. here is what I have so far.
import java.util.Scanner;
public class PrimeNumber
{
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
final int NUMBER_OF_PRIMES = 21;
final int NUMBER_OF_PRIMES_PER_LINE = 5;
int count = 0 ;
int number = 2;
System.out.println("enter a number value for a: ");
int nbr1 = input.nextInt();
System.out.println("Enter a number for b: ");
int nbr2= input.nextInt();
// Repeatedly find prime numbers
while (count < NUMBER_OF_PRIMES) {
boolean isPrime = true;
for (int divisor = 2; divisor <= number / 2; divisor++)
if (number % divisor == 0) {
isPrime = false;
break; }
if (isPrime) { count++;
if (count % NUMBER_OF_PRIMES_PER_LINE == 0) {
System.out.println(number); }
else {
System.out.print(number + " "); }
}
number++; } // End while
}
}
// End main }
I want to get the prime numbers between 101 and 200 but I keep getting the prime between 2 and 73
Output:
2 3 5 7 11
13 17 19 23 29
31 37 41 43 47
53 59 61 67 71
73

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

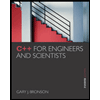
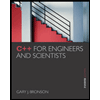