I don't know how to start the node server: const express = require('express'); const dotenv = require('dotenv'); dotenv.config(); const app = express(); const port = process.env.PORT || 3000; const host = process.env.HOST || 'localhost'; app.get('/dotted', (req, res) => { const { word1, word2 } = req.query; const dots = '.'.repeat(30 - (word1.length + word2.length)); const result = `${word1}${dots}${word2}`; res.type('html').send(result); }); app.get('/fizzBuzz', (req, res) => { const { start, end } = req.query; let result = ''; for (let i = start; i <= end; i++) { if (i % 3 === 0 && i % 5 === 0) { result += 'FizzBuzz\n'; } else if (i % 3 === 0) { result += 'Fizz\n'; } else if (i % 5 === 0) { result += 'Buzz\n'; } else { result += `${i}\n`; } } res.type('html').send(`${result.trim()}`); }); app.get('/gradeStats', (req, res) => { const grades = req.query.grades; const min = Math.min(...grades); const max = Math.max(...grades); const avg = (grades.reduce((acc, grade) => acc + grade) / grades.length).toFixed(2); res.json({ average: avg, minimum: min, maximum: max }); }); app.get('/rectangle', (req, res) => { const { length, width } = req.query; const area = length * width; const perimeter = 2 * (length + width); res.json({ area: area, perimeter: perimeter }); }); app.listen(port, host, () => { console.log(`Server listening at http://${host}:${port}`); });
I don't know how to start the node server:
const express = require('express');
const dotenv = require('dotenv');
dotenv.config();
const app = express();
const port = process.env.PORT || 3000;
const host = process.env.HOST || 'localhost';
app.get('/dotted', (req, res) => {
const { word1, word2 } = req.query;
const dots = '.'.repeat(30 - (word1.length + word2.length));
const result = `<pre>${word1}${dots}${word2}</pre>`;
res.type('html').send(result);
});
app.get('/fizzBuzz', (req, res) => {
const { start, end } = req.query;
let result = '';
for (let i = start; i <= end; i++) {
if (i % 3 === 0 && i % 5 === 0) {
result += 'FizzBuzz\n';
} else if (i % 3 === 0) {
result += 'Fizz\n';
} else if (i % 5 === 0) {
result += 'Buzz\n';
} else {
result += `${i}\n`;
}
}
res.type('html').send(`<pre>${result.trim()}</pre>`);
});
app.get('/gradeStats', (req, res) => {
const grades = req.query.grades;
const min = Math.min(...grades);
const max = Math.max(...grades);
const avg = (grades.reduce((acc, grade) => acc + grade) / grades.length).toFixed(2);
res.json({ average: avg, minimum: min, maximum: max });
});
app.get('/rectangle', (req, res) => {
const { length, width } = req.query;
const area = length * width;
const perimeter = 2 * (length + width);
res.json({ area: area, perimeter: perimeter });
});
app.listen(port, host, () => {
console.log(`Server listening at http://${host}:${port}`);
});

Step by step
Solved in 2 steps

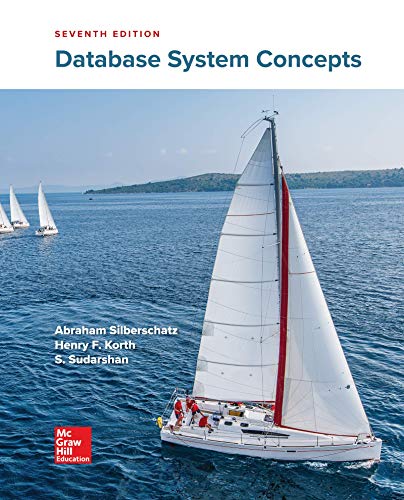
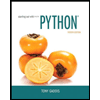
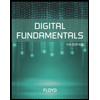
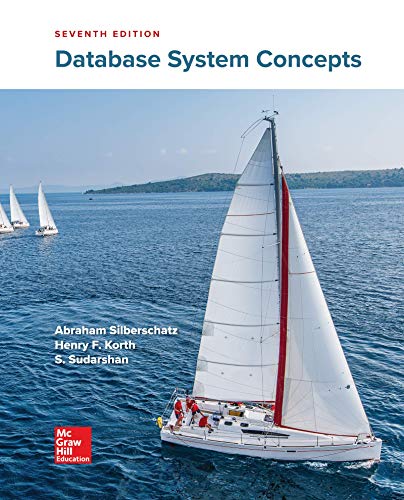
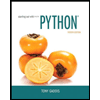
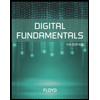
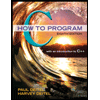
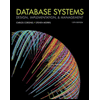
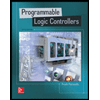