I have this code below and I only have to correctly display the available burgers and customer waiting. In what way should be edited so that it will have the same output as the example? class Producer implements Runnable { private final int burgers; public Producer(int burgers) { this.burgers = burgers; } @Override public void run() { for (int i = 1; i <= burgers; i++) { // First increment avail burgers MidExam.addAvailBurgers(true); // If there are avail burgers and there are waiting, decrement waiting and decrement avail burgers if (MidExam.getAvailBurgers() > 0 && MidExam.getWaiting() > 0) { MidExam.addWaiting(false); MidExam.addAvailBurgers(false); } System.out.printf("Producer is cooking burger #%d\t\t\t# of Available burgers [%d] Customer waiting: [%d]%n", i, MidExam.getAvailBurgers(), MidExam.getWaiting()); try { Thread.sleep(500); } catch (InterruptedException ex) { Thread.currentThread().interrupt(); } } } } class Consumer implements Runnable { private final String name; private final int burgers; public Consumer(int b, String n) { burgers = b; name = n; } @Override public synchronized void run() { for (int i = 1; i <= burgers; i++) { try { // If there are avail burgers, decrement waiting and avail burgers if (MidExam.getAvailBurgers() > 0) { MidExam.addWaiting(false); MidExam.addAvailBurgers(false); } // else increment waiting else { MidExam.addWaiting(true); } System.out.printf("Consumer #%d of %s buys 1 burger\t# of Available burgers [%d] Customer waiting: [%d]%n", i, name, MidExam.getAvailBurgers(), MidExam .getWaiting()); Thread.sleep(500); } catch (InterruptedException ex) { Thread.currentThread().interrupt(); } } } } public class MidExam { //private static final AtomicInteger tempAvail = new AtomicInteger(0); //private static final AtomicInteger tempWait = new AtomicInteger(0); private static int avail = 0; private static int wait = 0; public static int getAvailBurgers() { return avail; } public static int getWaiting() { return wait; } public static synchronized void addAvailBurgers(boolean isAdd) { if (isAdd) { avail++; } else { avail = (avail > 0) ? avail - 1 : 0; } } public static synchronized void addWaiting(boolean isAdd) { if (isAdd) { wait++; } else { wait = (wait > 0) ? wait - 1 : 0; } } public static void main(String[] args) { int burgers = (int) Math.floor(Math.random() * (6 - 1 + 1) + 1); System.out.printf("# of burgers to cook per Customer = %d %n%n", burgers); Thread producer = new Thread(new Producer(burgers * 2)); producer.start(); Thread c1 = new Thread(new Consumer(burgers, "Thread #1")); c1.start(); Thread c2 = new Thread(new Consumer(burgers, "Thread #2")); c2.start(); } }
I have this code below and I only have to correctly display the available burgers and customer waiting. In what way should be edited so that it will have the same output as the example?
class Producer implements Runnable {
private final int burgers;
public Producer(int burgers) {
this.burgers = burgers;
}
@Override
public void run() {
for (int i = 1; i <= burgers; i++) {
// First increment avail burgers
MidExam.addAvailBurgers(true);
// If there are avail burgers and there are waiting, decrement waiting and decrement avail burgers
if (MidExam.getAvailBurgers() > 0 && MidExam.getWaiting() > 0) {
MidExam.addWaiting(false);
MidExam.addAvailBurgers(false);
}
System.out.printf("Producer is cooking burger #%d\t\t\t# of Available burgers [%d] Customer waiting: [%d]%n",
i, MidExam.getAvailBurgers(), MidExam.getWaiting());
try {
Thread.sleep(500);
} catch (InterruptedException ex) {
Thread.currentThread().interrupt();
}
}
}
}
class Consumer implements Runnable {
private final String name;
private final int burgers;
public Consumer(int b, String n) {
burgers = b;
name = n;
}
@Override
public synchronized void run() {
for (int i = 1; i <= burgers; i++) {
try {
// If there are avail burgers, decrement waiting and avail burgers
if (MidExam.getAvailBurgers() > 0) {
MidExam.addWaiting(false);
MidExam.addAvailBurgers(false);
}
// else increment waiting
else {
MidExam.addWaiting(true);
}
System.out.printf("Consumer #%d of %s buys 1 burger\t# of Available burgers [%d] Customer waiting: [%d]%n",
i, name, MidExam.getAvailBurgers(), MidExam .getWaiting());
Thread.sleep(500);
} catch (InterruptedException ex) { Thread.currentThread().interrupt(); }
}
}
}
public class MidExam {
//private static final AtomicInteger tempAvail = new AtomicInteger(0);
//private static final AtomicInteger tempWait = new AtomicInteger(0);
private static int avail = 0;
private static int wait = 0;
public static int getAvailBurgers() {
return avail;
}
public static int getWaiting() {
return wait;
}
public static synchronized void addAvailBurgers(boolean isAdd) {
if (isAdd) {
avail++;
}
else {
avail = (avail > 0) ? avail - 1 : 0;
}
}
public static synchronized void addWaiting(boolean isAdd) {
if (isAdd) {
wait++;
}
else {
wait = (wait > 0) ? wait - 1 : 0;
}
}
public static void main(String[] args) {
int burgers = (int) Math.floor(Math.random() * (6 - 1 + 1) + 1);
System.out.printf("# of burgers to cook per Customer = %d %n%n", burgers);
Thread producer = new Thread(new Producer(burgers * 2));
producer.start();
Thread c1 = new Thread(new Consumer(burgers, "Thread #1"));
c1.start();
Thread c2 = new Thread(new Consumer(burgers, "Thread #2"));
c2.start();
}
}
![Sample output: Assume the system generates 4
Command Prompt
# of burgers to cook per Consumer = 4
Producer is Cooking Burger #1
Producer is Cooking Burger #2
Consumer #1 of Thread# 1 buys 1 burger
Consumer #2 of Thread# 1 buys 1 burger
Producer is Cooking Burger #3
Consumer #1 of Thread# 2 buys 1 burger
Producer is Cooking Burger #4
Producer is Cooking Burger #5
Producer is Cooking Burger #6
Consumer #3 of Thread# 1 buys 1 burger
Producer is Cooking Burger #7
Consumer #2 of Thread# 2 buys 1 burger
Consumer #3 of Thread# 2 buys 1 burger
Producer is Cooking Burger #8
Consumer #4 of Thread# 1 buys 1 burger
Consumer #4 of Thread# 2 buys 1 burger
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [3] Customer(s) Waiting: [0]
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [3] Customer(s) Waiting: [0]
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting:
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [0]
Sample output: Assume the
system generates 2
%3D
Command Prompt
# of burgers to cook per Consumer = 2
Producer is Cooking Burger #1
Consumer #1 of Thread# 1 buys 1 burger
Consumer #1 of Thread# 2 buys 1 burger
Consumer #2 of Thread# 1 buys 1 burger
Producer is Cooking Burger #2
Consumer #2 of Thread# 2 buys 1 burger
Producer is Cooking Burger #3
Producer is Cooking Burger #4
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [1]
# of Available burgers [0] Customer(s) Waiting: [2]
# of Available burgers [0] Customer(s) Waiting: [1]
# of Available burgers [0] Customer(s) Waiting: [2]
# of Available burgers [0] Customer(s) Waiting: [1]
# of Available burgers [0] Customer(s) Waiting: [0]
Note:
|Customer(s) Waiting: [1]
Since There are no more Available burgers created by the Producer Thread, the Customer “waits" !
until the Producer makes a new Burger.
Producer is Cooking Burger #2 # of Available burgers [0] Customer(s) Waiting: [1]
Although the Producer creates a new Burger, It will not be counted on the “Available" because
there were 2 customer(s) waiting. That's why on this line, Waiting was instead decremented
from 2 (see previous line) to 1.
Waiting: [1]
The same concept happened here.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0e34f58a-8202-406f-a590-818ff4ef050c%2Fa2dd9b1f-0f55-4678-9a18-9ba0e99897a0%2F5s76s0w_processed.jpeg&w=3840&q=75)

Step by step
Solved in 6 steps with 6 images

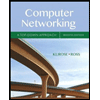
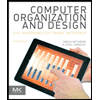
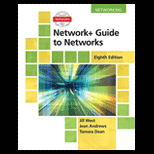
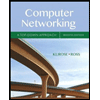
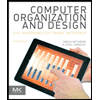
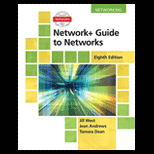
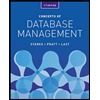
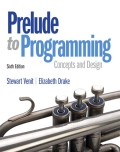
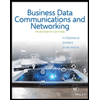