I have written a code for diving fractions but it will not compute. How do I fix my code to divide the fractions? class Fraction: #Constructor def __init__(self, numerator, denominator): self.numer = numerator self.denom = denominator def __str__(self): return str(self.numer) + "/" + str(self.denom) def gcd(n, d): while n%d != 0: oldnumer = n olddenom = d n = olddenom d = oldnumer%olddenom return d #Division method def __div__(self, otherFraction): newnumer = self.numer*otherFraction.denom newdenom = otherFraction.numer*self.denom common = Fraction.gcd(newnumer, newdenom) return Fraction(newnumer//common, newdenom//common) fractionOne = Fraction(2, 8) fractionTwo = Fraction(4, 8) fractionThree = fractionOne//fractionTwo print(fractionThree)
I have written a code for diving fractions but it will not compute. How do I fix my code to divide the fractions?
class Fraction:
#Constructor
def __init__(self, numerator, denominator):
self.numer = numerator
self.denom = denominator
def __str__(self):
return str(self.numer) + "/" + str(self.denom)
def gcd(n, d):
while n%d != 0:
oldnumer = n
olddenom = d
n = olddenom
d = oldnumer%olddenom
return d
#Division method
def __div__(self, otherFraction):
newnumer = self.numer*otherFraction.denom
newdenom = otherFraction.numer*self.denom
common = Fraction.gcd(newnumer, newdenom)
return Fraction(newnumer//common, newdenom//common)
fractionOne = Fraction(2, 8)
fractionTwo = Fraction(4, 8)
fractionThree = fractionOne//fractionTwo
print(fractionThree)

Step by step
Solved in 3 steps

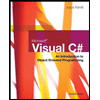
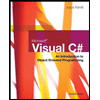