I need help fixing my function for bool DelOddCopEven(Node*& headPtr). When I run my code it pass the first test but it crash after that. I am to replicate the test output but I don't know where the error in my function. Here is my results is my results. ================================ passed test on empty list test case 1 of 1000000 given list: 6 7 5 3 ================================ testing case 1 of 1000000 ================================ test case 1 of 1000000 initial: 6 7 5 3 ought2b: 6 6 outcome: 6 6 test case 2 of 1000000 given list: 6 2 9 1 2 7 ================================ testing case 2 of 1000000 ================================ test case 2 of 1000000 initial: 6 2 9 1 2 7 ought2b: 6 6 2 2 2 2 outcome: 6 6 2 2 2 2 test case 3 of 1000000 given list: 9 signal: segmentation fault (core dumped) And here is the test output ================================ passed test on empty list ================================ test case 1 of 1000000 initial: 6 7 5 3 ought2b: 6 6 outcome: 6 6 ================================ test case 2 of 1000000 initial: 6 2 9 1 2 7 ought2b: 6 6 2 2 2 2 outcome: 6 6 2 2 2 2 ================================ test case 3 of 1000000 initial: 9 ought2b: outcome: ================================ test case 4 of 1000000 initial: 6 0 6 2 ought2b: 6 6 0 0 6 6 2 2 outcome: 6 6 0 0 6 6 2 2 ================================ test case 5 of 1000000 initial: 1 8 7 9 2 0 2 ought2b: 8 8 2 2 0 0 2 2 outcome: 8 8 2 2 0 0 2 2 ================================ test case 40000 of 1000000 initial: 5 5 1 ought2b: outcome: ================================ test case 80000 of 1000000 initial: 2 ought2b: 2 2 outcome: 2 2 ================================ test case 120000 of 1000000 initial: 4 4 1 8 ought2b: 4 4 4 4 8 8 outcome: 4 4 4 4 8 8 ================================ test case 160000 of 1000000 initial: 0 4 6 ought2b: 0 0 4 4 6 6 outcome: 0 0 4 4 6 6 ================================ #ifndef LLCP_INT_H #define LLCP_INT_H #include struct Node { int data; Node *link; }; bool DelOddCopEven(Node* headPtr); int FindListLength(Node* headPtr); bool IsSortedUp(Node* headPtr); void InsertAsHead(Node*& headPtr, int value); void InsertAsTail(Node*& headPtr, int value); void InsertSortedUp(Node*& headPtr, int value); bool DelFirstTargetNode(Node*& headPtr, int target); bool DelNodeBefore1stMatch(Node*& headPtr, int target); void ShowAll(std::ostream& outs, Node* headPtr); void FindMinMax(Node* headPtr, int& minValue, int& maxValue); double FindAverage(Node* headPtr); void ListClear(Node*& headPtr, int noMsg = 0); // prototype of DelOddCopEven of Assignment 5 Part 1 #endif
I need help fixing my function for bool DelOddCopEven(Node*& headPtr). When I run my code it pass the first test but it crash after that. I am to replicate the test output but I don't know where the error in my function. Here is my results is my results.
================================
passed test on empty list
test case 1 of 1000000
given list: 6 7 5 3
================================
testing case 1 of 1000000
================================
test case 1 of 1000000
initial: 6 7 5 3
ought2b: 6 6
outcome: 6 6
test case 2 of 1000000
given list: 6 2 9 1 2 7
================================
testing case 2 of 1000000
================================
test case 2 of 1000000
initial: 6 2 9 1 2 7
ought2b: 6 6 2 2 2 2
outcome: 6 6 2 2 2 2
test case 3 of 1000000
given list: 9
signal: segmentation fault (core dumped)
And here is the test output
================================
passed test on empty list
================================
test case 1 of 1000000
initial: 6 7 5 3
ought2b: 6 6
outcome: 6 6
================================
test case 2 of 1000000
initial: 6 2 9 1 2 7
ought2b: 6 6 2 2 2 2
outcome: 6 6 2 2 2 2
================================
test case 3 of 1000000
initial: 9
ought2b:
outcome:
================================
test case 4 of 1000000
initial: 6 0 6 2
ought2b: 6 6 0 0 6 6 2 2
outcome: 6 6 0 0 6 6 2 2
================================
test case 5 of 1000000
initial: 1 8 7 9 2 0 2
ought2b: 8 8 2 2 0 0 2 2
outcome: 8 8 2 2 0 0 2 2
================================
test case 40000 of 1000000
initial: 5 5 1
ought2b:
outcome:
================================
test case 80000 of 1000000
initial: 2
ought2b: 2 2
outcome: 2 2
================================
test case 120000 of 1000000
initial: 4 4 1 8
ought2b: 4 4 4 4 8 8
outcome: 4 4 4 4 8 8
================================
test case 160000 of 1000000
initial: 0 4 6
ought2b: 0 0 4 4 6 6
outcome: 0 0 4 4 6 6
================================
#ifndef LLCP_INT_H
#define LLCP_INT_H
#include <iostream>
struct Node
{
int data;
Node *link;
};
bool DelOddCopEven(Node* headPtr);
int FindListLength(Node* headPtr);
bool IsSortedUp(Node* headPtr);
void InsertAsHead(Node*& headPtr, int value);
void InsertAsTail(Node*& headPtr, int value);
void InsertSortedUp(Node*& headPtr, int value);
bool DelFirstTargetNode(Node*& headPtr, int target);
bool DelNodeBefore1stMatch(Node*& headPtr, int target);
void ShowAll(std::ostream& outs, Node* headPtr);
void FindMinMax(Node* headPtr, int& minValue, int& maxValue);
double FindAverage(Node* headPtr);
void ListClear(Node*& headPtr, int noMsg = 0);
// prototype of DelOddCopEven of Assignment 5 Part 1
#endif
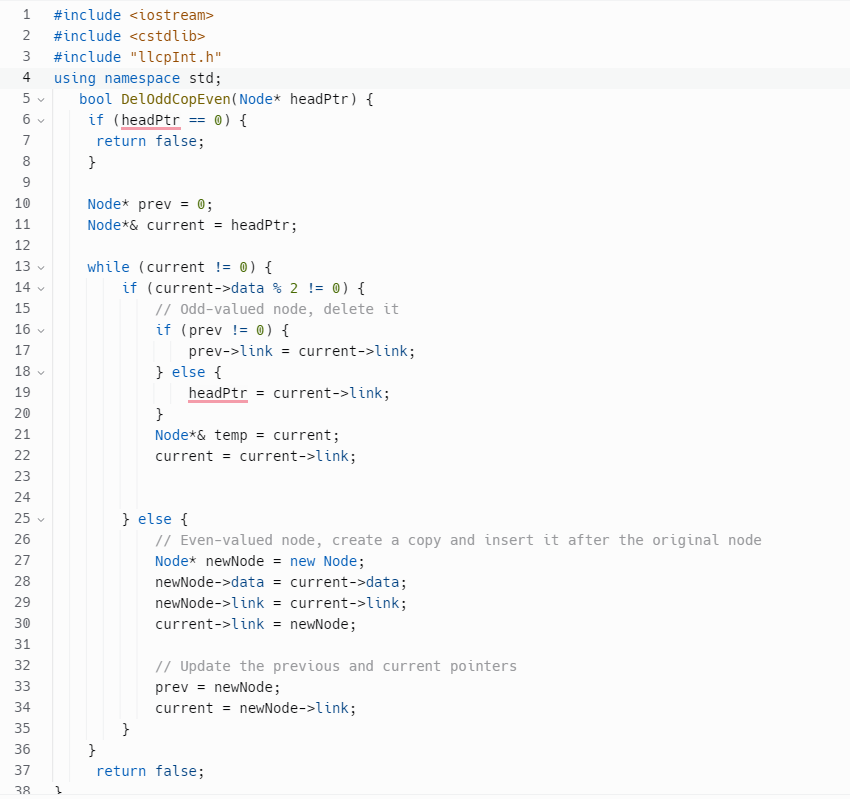

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

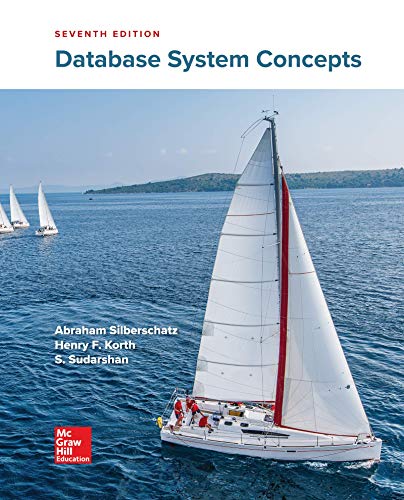
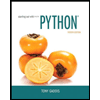
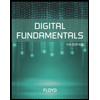
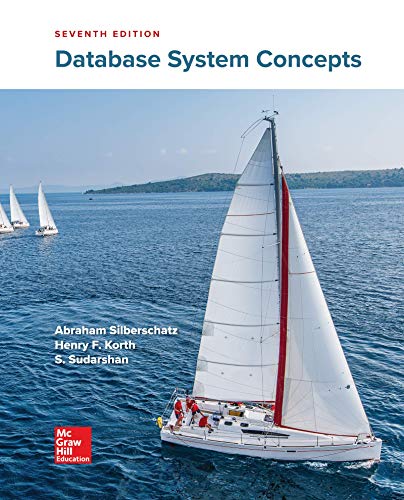
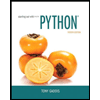
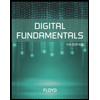
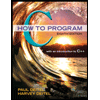
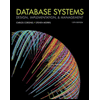
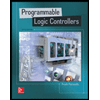