I need help in improving my Elevator class and it's subclasses code. I already provided my code at the bottom. This is an elevator simulator that uses polymorphism and object-oriented programming to simulate the movement of elevators in a building with multiple types of passengers and elevators. There are 4 types of elevators in the system: StandardElevator: This is the most common type of elevator and has a request percentage of 70%.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
I need help in improving my Elevator class and it's subclasses code. I already provided my code at the bottom. This is an elevator simulator that uses polymorphism and object-oriented
There are 4 types of elevators in the system:
StandardElevator: This is the most common type of elevator and has a request percentage of 70%.
Standard elevators have a maximum capacity of 10 passengers.
ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a
maximum capacity of 8 passengers and are faster than standard elevators.
FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a
maximum capacity of 5 passengers and are designed to transport heavy items.
GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a
maximum capacity of 6 passengers and are designed to transport fragile item
Classes requirements:
Elevator {
Holds variable and ArrayList needed for each elevators
Holds setters and getters
}
Standard, Express, Glass, Freight subclasses {
Each keep track of the amount of Elevators
Contains method of generating passengers
}
My code:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Random;
import java.util.Scanner;
public class Simulation {
SimulatorSettings settings;
public void initSimulation(SimulatorSettings settings) throws FileNotFoundException {
Passenger.passengerCounter = 0;
this.settings = settings;
File file = new File("settings.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
if (line.startsWith("floor=")) {
line = line.replace("floor=", "");
settings.setNofloors(Integer.parseInt(line));
}
}
ArrayList<Passenger> passengers = new ArrayList<>();
for (int i = 0; i < 100; i++) {
// Use the percentage from the file
passengers.add(new StandardPassenger());
}
}
}
public class SimulatorSettings {
SimulatorSettings(String fileName){
}
private int nofloors;
public int getNofloors() {
return nofloors;
}
public void setNofloors(int nofloors) {
this.nofloors = nofloors;
}
}
abstract class Passenger {
private static int passengerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
protected static Random rand = new Random();
public Passenger() {
this.passengerID = String.valueOf(passengerCounter);
passengerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
public abstract class Elevator{
private int currentFloor;
private int maxCapacity;
private int passengersCount;
public Elevator(int maxCapacity) {
this.currentFloor = 0; // assuming initial floor is 0
this.maxCapacity = maxCapacity;
this.passengersCount = 0;
}
public abstract void moveUp();
public abstract void moveDown();
public abstract void addPassenger(Passenger passenger);
public abstract void removePassenger(Passenger passenger);
}
class StandardElevator extends Elevator{
public StandardElevator() {
super(10); // max capacity is 10 for StandardElevator
}
@Override
public void moveUp() {
// implementation for moving up for StandardElevator
}
@Override
public void moveDown() {
// implementation for moving down for StandardElevator
}
@Override
public void addPassenger(Passenger passenger) {
// implementation for adding passenger to StandardElevator
}
@Override
public void removePassenger(Passenger passenger) {
// implementation for removing passenger from StandardElevator
}
}
public class FreightElevator extends Elevator{
public FreightElevator() {
super( 4);
}
}
public class GlassElevator extends Elevator{
public GlassElevator() {
super( 8);
}
}
class ExpressElevator extends Elevator{
public ExpressElevator() {
super(8); // max capacity is 8 for ExpressElevator
}
}

Step by step
Solved in 3 steps

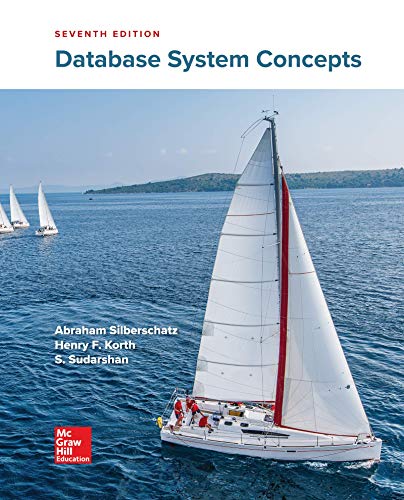
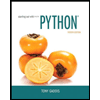
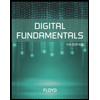
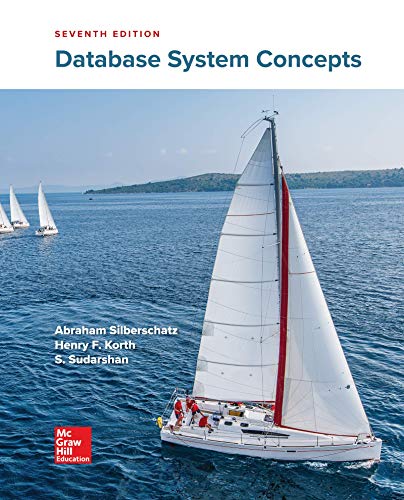
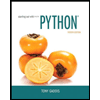
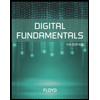
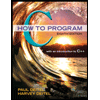
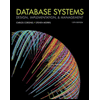
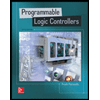