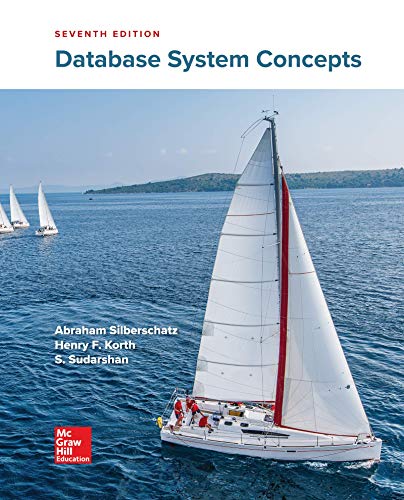
I need help with a java code problem that needs this output shown in image below:
import java.util.Scanner;
import java.util.NoSuchElementException;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int val1 = 0, val2 = 0, val3 = 0; // Initialize the variables
int max;
int inputCounter = 0; // Counter to keep track of the number of inputs read
try {
String inputLine = scnr.nextLine();
String[] parts = inputLine.split(" ");
if (parts.length > 0 && !parts[0].isEmpty()) {
val1 = Integer.parseInt(parts[0]);
inputCounter++;
}
if (parts.length > 1 && !parts[1].isEmpty()) {
val2 = Integer.parseInt(parts[1]);
inputCounter++;
}
if (parts.length > 2 && !parts[2].isEmpty()) {
val3 = Integer.parseInt(parts[2]);
inputCounter++;
}
// If no inputs were provided
if(inputCounter <= 1 ) {
throw new NoSuchElementException();
}
// Determine the max value
max = val1;
if (val2 > max) {
max = val2;
}
if (val3 > max) {
max = val3;
}
System.out.println(max);
} catch (NoSuchElementException ex) {
// If exception is caught, check how many inputs were read and print the results
if (inputCounter == 0) {
System.out.println("0 input(s) read:\nNo max");
} else if (inputCounter == 1) {
System.out.println("1 input(s) read:\nMax is " + val1);
}
} catch (NumberFormatException ex) {
System.out.println("Invalid input. Please provide integers.");
}
}
}
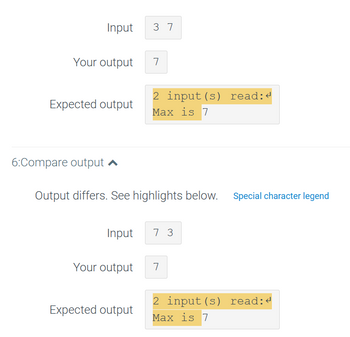

Step by stepSolved in 3 steps with 1 images

- Please write in Java import java.util.Scanner; public class ConcertPromoter { public static void main(String[] args) { Scanner key = new Scanner(System.in); Concert concert = new Concert(); System.out.println("Welcome to the Concert Promotion tool!"); String input = ""; while(input.equalsIgnoreCase("quit")!= true) { System.out.println("Currently the concert featuring the band: "+concert.getBandName()); System.out.println("Has sold "+concert.getNumTicketsSoldByPhone()+" tickets by phone"); System.out.println("Has sold "+concert.getNumTicketsSoldAtVenue()+" tickets at the venue"); System.out.println("And has grossed $"+concert.totalSales()); System.out.println("What would you like to do?\n" + "Enter 1: To change name\n" + "Enter 2: To change ticket by phone price\n" + "Enter 3: To change ticket at venue price\n" + "Enter 4: To add tickets by phone\n" + "Enter 5: To add tickets at the venue\n" + "Enter…arrow_forwardPlease answer question. This is pertaining to Java programming language 3-15arrow_forwardI'm getting this progm to run but vis producing no out put. import java.util.Scanner;import java.lang.Math; public class CarValue { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Car myCar = new Car(); int userYear = scnr.nextInt(); int userPrice = scnr.nextInt(); int userCurrentYear = scnr.nextInt(); myCar.setModelYear(userYear); myCar.setPurchasePrice(userPrice); myCar.calcCurrentValue(userCurrentYear); myCar.printInfo(); }} the calculations are performed in this prigram. private int currentValue; public void setModelYear(int userYear){ modelYear = userYear; } public int getModelYear() { return modelYear; } // Define setPurchasePrice() method public double setPurchasePrice(int userPrice){ return purchasePrice; } // Define getPurchasePrice() method public double getPurchasePrice(int userPrice){ return purchasePrice; }…arrow_forward
- I have my code here and I am getting errors (I have also attached my code): " import java.util.Compiler; public class Test Score { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Ënter the first exam Score: "); Double Score1 = scan.nextDouble(); System.out.println("Enter the second exam Score: "); Double Score2 = scan.nextDouble(); System.out.println("Enter the third exam Score: "); Double Score3 = scan.nextDouble(); System.out.println("Enter the fourth exam Score: "); Double Score4 = scan.nextDouble(); System.out.println("Enter the fifth exam Score: "); Double Score5 = scan.nextDouble(); Double total = Score1 + Score2 + Score3 + Score4 + Score5 Double average = total/5; if (average >= 90) { System.out.printf("your average was a: %f and recieved an A in this Class", average ); } else if (average >= 80) {…arrow_forwardJAVA CODE: check outputarrow_forwardWrite code that prints: countNum ... 21 Print a newline after each number. Ex: If the input is: 3 the output is: 3 2 1 1 import java.util.Scanner; 3 public class ForLoops { UAWN HOUх и блашин 2 ST 4 5 6 7 8 9 10 11 12 13 14 15 } public static void main (String [] args) { int countNum; int i; Scanner input = new Scanner(System.in); input.nextInt(); countNum = for * Your code goes here *) { System.out.println(i); } }arrow_forward
- The language is Java. The code provided is what I have however it is not outputting the correct number, only 0.arrow_forwardI need help with fixing this java program as described in the image below: import java.util.Scanner; public class LabProgram { /* TODO: Write recursive drawTriangle() method here. */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int baseLength; baseLength = scnr.nextInt(); drawTriangle(baseLength); } }arrow_forwardI need help fixing this code so that it can print outputs described in the image below: import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int currentPrice; int lastMonthsPrice; double mortgage; currentPrice = scnr.nextInt(); lastMonthsPrice = scnr.nextInt(); mortgage = (currentPrice * 0.051) / 12; System.out.println("This house is $" + currentPrice + ". The change is -100000 since last month."); System.out.println("The estimated monthly mortgage is $" + mortgage + "."); }}arrow_forward
- Hi I am having some difficulties with this problem, I am not sure why my code is giving an incorrect output. Here is the provided code: import java.util.Scanner; public class DrawHalfArrow { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int arrowBaseHeight; int arrowBaseWidth; int arrowHeadWidth; System.out.println("Enter arrow base height:"); arrowBaseHeight = scnr.nextInt(); System.out.println("Enter arrow base width:"); arrowBaseWidth = scnr.nextInt(); System.out.println("Enter arrow head width:"); arrowHeadWidth = scnr.nextInt(); System.out.println(""); // Draw arrow base (height = 3, width = 2) System.out.println("**"); System.out.println("**"); System.out.println("**"); // Draw arrow head (width = 4) System.out.println("****"); System.out.println("***"); System.out.println("**"); System.out.println("*"); }}…arrow_forwardI need help fixing this Java program: import java.util.Scanner; public class TimeConverter { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int totalYears; int numMillennia; int numCenturies; int numYears; totalYears = scnr.nextInt(); /* Your code goes here */ System.out.println("Millennia: " + numMillennia); System.out.println("Centuries: " + numCenturies); System.out.println("Years: " + numYears); } }arrow_forwardHelp me find the output to this problem and how to solve itarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
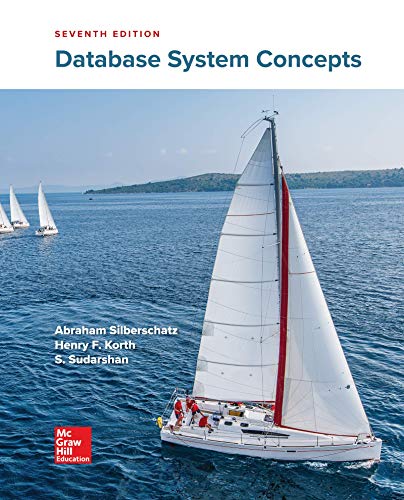
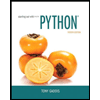
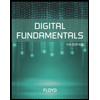
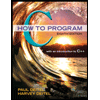
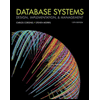
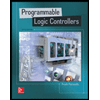