I need help with my Java compiler Generating the final machine code, such as x86 assembly language, that can be executed on a computer. import java.util.*; public class SimpleCalculator { private final String input; private int position; private boolean hasDivByZero = false; private Map symbolTable; private List instructions; public SimpleCalculator(String input) { this.input = input; this.position = 0; this.symbolTable = new HashMap<>(); this.instructions = new ArrayList<>(); } public static void main(String[] args) { SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)"); calculator.parseExpression(); if (calculator.hasDivByZero) { System.out.println("Error: division by zero"); } else { System.out.println("Result: " + calculator.instructions); } } public void parseExpression() { parseTerm('+'); } public String parseTerm(char op) { String t1 = parseFactor(); while (peek() == op || peek() == '/') { char operator = consume(); String t2 = parseFactor(); if (operator == '/' && t2.equals("0")) { hasDivByZero = true; break; } String result = newTemp(); emit(result + " = " + t1 + " " + operator + " " + t2); t1 = result; } return t1; } public String parseFactor() { if (consume('(')) { String result = parseExpression(); consume(')'); return result; } StringBuilder sb = new StringBuilder(); while (Character.isDigit(peek())) { sb.append(consume()); } String variable = sb.toString(); Optional.ofNullable(symbolTable.get(variable)) .filter(type -> type.equals("int")) .orElseThrow(() -> new IllegalArgumentException("Error: " + variable + " is not defined or not an integer")); return variable; } private String newTemp() { String temp = "t" + (instructions.size() + 1); symbolTable.put(temp, "int"); return temp; } private void emit(String instruction) { instructions.add(instruction); } private char consume() { return input.charAt(position++); } private boolean consume(char c) { if (peek() == c) { position++; return true; } return false; } private char peek() { return position < input.length() ? input.charAt(position) : '\0'; } }
I need help with my Java compiler Generating the final machine code, such as x86 assembly language, that can be executed
on a computer.
import java.util.*;
public class SimpleCalculator {
private final String input;
private int position;
private boolean hasDivByZero = false;
private Map<String, String> symbolTable;
private List<String> instructions;
public SimpleCalculator(String input) {
this.input = input;
this.position = 0;
this.symbolTable = new HashMap<>();
this.instructions = new ArrayList<>();
}
public static void main(String[] args) {
SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)");
calculator.parseExpression();
if (calculator.hasDivByZero) {
System.out.println("Error: division by zero");
} else {
System.out.println("Result: " + calculator.instructions);
}
}
public void parseExpression() {
parseTerm('+');
}
public String parseTerm(char op) {
String t1 = parseFactor();
while (peek() == op || peek() == '/') {
char operator = consume();
String t2 = parseFactor();
if (operator == '/' && t2.equals("0")) {
hasDivByZero = true;
break;
}
String result = newTemp();
emit(result + " = " + t1 + " " + operator + " " + t2);
t1 = result;
}
return t1;
}
public String parseFactor() {
if (consume('(')) {
String result = parseExpression();
consume(')');
return result;
}
StringBuilder sb = new StringBuilder();
while (Character.isDigit(peek())) {
sb.append(consume());
}
String variable = sb.toString();
Optional.ofNullable(symbolTable.get(variable))
.filter(type -> type.equals("int"))
.orElseThrow(() -> new IllegalArgumentException("Error: " + variable + " is not defined or not an integer"));
return variable;
}
private String newTemp() {
String temp = "t" + (instructions.size() + 1);
symbolTable.put(temp, "int");
return temp;
}
private void emit(String instruction) {
instructions.add(instruction);
}
private char consume() {
return input.charAt(position++);
}
private boolean consume(char c) {
if (peek() == c) {
position++;
return true;
}
return false;
}
private char peek() {
return position < input.length() ? input.charAt(position) : '\0';
}
}

Step by step
Solved in 3 steps

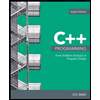
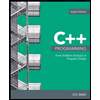