i want ".h" header file Separate from this code "Your program must embrace modular programming technique should be menu-driven. Functions of similar operations can be grouped & stored as separate C files. Headerfiles are to be stored separately as .h files."
i want ".h" header file Separate from this code
"Your program must embrace modular
programming technique should be menu-driven. Functions of similar
operations can be grouped & stored as separate C files. Headerfiles are to be stored separately as .h files."
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct vacc{
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float population;
}v[10];
// Function Declarations
void create_inventory();
void update_vacc_qty();
int search_vaccine();
void display_vaccine();
// Main Function starts here
int main()
{
int ch;
do {
printf("\n 1:create inventory");
printf("\n 2: display vaccine info ");
printf("\n 3: search vaccine");
printf("\n enter your choice (0 to exit):");
scanf("%d",&ch);
switch (ch)
{
case 1: create_inventory();
break;
case 2: display_vaccine();
break;
case 3: search_vaccine();
break;
default : break;
}
}while(ch!=0);
return 0;
}
//Function to Create Vaccine.txt as per the given table
void create_inventory()
{
int option = 1;
// variables to collect data as per table given
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float populaion;
//File definition
FILE *infile;
infile = fopen("dist.txt","w"); // file opening for writing
if(infile == NULL) // Checking for the file creation
{
printf("Vaccine.txt file not found\n");
}
//Accepting data from user from keyboard till user enters 0 to close
while(option != 0)
{
printf("Enter Vaccine Name : ");
scanf("%s",vaccName);
printf("Enter Vaccine Code : ");
scanf("%s",vaccCode);
printf("Enter Counry : ");
scanf("%s",country);
printf("Enter Dosage Required : ");
scanf("%d",&qty);
printf("Enter Population Covered : ");
scanf("%f",&populaion);
//writing to the file using fprintf command
fprintf(infile,"%s %s %s %d %3.2f\n", vaccName,vaccCode,country,qty,populaion);
printf("\nEnter 1 to continue and 0 to exit : ");
scanf("%d",&option);
if(option == 0)
fclose(infile); // closing the file when user wants to exit
}
}
//Function to display the file contents in a formatted way
void display_vaccine()
{
// variables to collect data as per table given
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float populaion;
FILE *infile;
infile = fopen("Vaccine.txt","r"); // file opening for reading
if(infile == NULL) //checking for file exists or not
{
printf("Vaccine.txt file not found\n");
}
printf("%15s\t%2s\t%15s\t%6s\t%10s\n","Vaccine Name","Vaccine Code","Country","Dosage","Population");
// Reading the file
while(fscanf(infile,"%s %s %s %d %f\n",vaccName,vaccCode,country,&qty,&populaion) !=EOF)
{
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n",vaccName,vaccCode,country,qty,populaion);
}
fclose(infile); // closing the file
}
void update_vacc_qty()
{
int t, option;
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float population;
FILE *infile;
infile = fopen("dist.txt","w");
if(infile == NULL)
{
printf("dist.txt file not found\n");
}
//Accepting data from user from keyboard till user enters 0 to close
while(option != 0)
{
printf("Enter Vaccine Name : ");
scanf("%s",vaccName);
printf("Enter Vaccine Code : ");
scanf("%s",vaccCode);
printf("Enter Counry : ");
scanf("%s",country);
printf("Enter Dosage Required : ");
scanf("%d",&qty);
printf("Enter Population Covered : ");
scanf("%f",&population);
//writing to the file using fprintf command
fprintf(infile,"%s %s %s %d %3.2f\n", vaccName,vaccCode,country,qty,population);
printf("\nEnter 1 to continue and 0 to exit : ");
scanf("%d",&option);
if(option == 0)
fclose(infile);
}
{
printf("dist.txt file not found\n");
}
t=0;
while(fscanf(infile,"%s %s %s %d %f\n",vaccName,vaccCode,country,&qty,&population) !=EOF)
{
//printf("%s",vaccName);
strcpy(v[t].vaccName,vaccName);
strcpy(v[t].vaccCode,vaccCode);
strcpy(v[t].country,country);
v[t].qty=qty;
v[t].population=population;
t++;
}
struct vacc temp;
for (int i = 0; i < t - 1; i++)
{
for (int j = 0; j < (t - 1-i); j++)
{
if (v[j].qty < v[j + 1].qty)
{
temp = v[j];
v[j] = v[j + 1];
v[j + 1] = temp;
}
}
}
printf("%15s\t%2s\t%15s\t%6s\t%10s\n","Vaccine Name","Vaccine Code","Country","Dosage","Population");
for(int i=0;i<t; i++){
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n",v[i].vaccName,v[i].vaccCode,v[i].country,v[i].qty,v[i].population);
}
fclose(infile); // closing the file
}
int search_vaccine()
{
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float populaion;
FILE *infile;
char vcode[2];
char temp[2];
int value;
infile = fopen("Vaccine.txt","r");
//getting the vaccine code from user through keyboard to search
printf("Enter Vaccine Code to Search : ");
scanf("%s",vcode);
if(infile == NULL) // checking for file existence
{
printf("Vaccine.txt file not found\n");
}
strcpy(temp,vcode);
//Reading the file
while(fscanf(infile,"%s %s %s %d %f\n",vaccName,vaccCode,country,&qty,&populaion) !=EOF)
{
//checking user entered vaccine code and available in the file is same
if(vaccCode[0] == temp[0] && vaccCode[1] == temp[1])
{
// Printing the matched record
printf("%15s\t%2s\t%15s\t%6s\t%10s\n","Vaccine Name"," Vaccine Code","Country","Dosage","Population");
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n",vaccName,vaccCode,country,qty,populaion);
}
}
fclose(infile);
}

Step by step
Solved in 2 steps

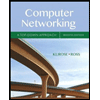
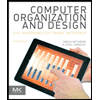
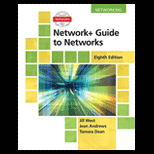
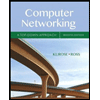
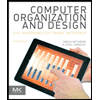
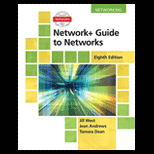
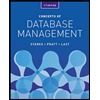
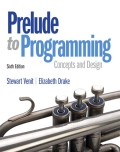
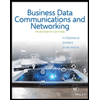