ICS 31, Summer Session 10-wk, 2022 Assignment 5 You will write a text adventure game. The idea of a text adventure game is that the player is in a virtual room in a "dungeon", and each room has a text description associated with it, such as, "This room in big and brightly lit. It has doors on the north and west walls". The player can move from room room using compass directional commands. Your program will be a function called adventure () which takes no arguments and returns no values. Your function adventure () will allow the user to enter commands related to moving through the dungeon and interacting with objects. Your function should print out a prompt to indicate that the user can enter a command. Your prompt should be a '$' character. At the prompt the user will type a command followed by a set of arguments for the command. Your function will perform whatever action is indicated by the command, and then your program will print a prompt on a new line in order to let the user know that he/she can type a new command. If the command is a compass command which moves the player into a new room, your program will print the description of the new room before printing the prompt on the next line. Dungeon File Each time a user plays the adventure game, he will be able to choose a dungeon to play in. The rooms in the dungeon, and their arrangement, is stored in a dungeon file. When the game starts, the player will have to issue a command to load a dungeon file describing the dungeon. The dungeon file will be a text file containing information about each room in a specific format. Each line of the file will contain information about a single room in the dungeon. Each room is associated with the following 6 fields of information. 1. Room number. This is a positive integer which uniquely identifies the room. 2. Description: This is a string which is printed when the player enters the room. This string is delimited by '+' symbols rather than the regular quotes. 3. North room: This is the room number of the room immediately to the north of this room. If there is no room to the north of this room then this value is -1. 4. South room: This is the room number of the room immediately to the south of this room. If there is no room to the south of this room then this value is -1. 5. East room: This is the room number of the room immediately to the east of this room. If there is no room to the east of this room then this value is -1. 6. West room: This is the room number of the room immediately to the west of this room. If there. is no room to the west of this room then this value is -1. All 6 fields of information about each room are contained in order on a single line in the dungeon file. Each field of information is separated by one or more spaces. The room described on the first line of the file is the initial room where the player will start after the dungeon file is loaded. Here is an example dungeon file. Be aware that this is only an example and that your code must work. with any valid dungeon file having any file name.. 1 This is an open field west of a white house, with a boarded front door. 2 -1 -1 -1 2 +North of House: You are facing the north side of a white house.+-1 1 3 -1 3 +Behind House: You are behind the white house.+ -1 4-1 2 4 South of House: You are facing the south side of a white house.+ 3 -1 -1 -1 Your program should accept the following command related to dungeon files: 1. loaddungeon: This command reads a dungeon file, allowing the player to explore the dungeon. The function takes one argument, the name of the dungeon file. The function returns no values. After executing this command the player should be in the room described on the first line of the dungeon file, and the description of the first room should be printed. The loaddungeon command can only be issued once. If the player tries to issue the command a second time, the error "Dungeon already loaded" should be printed. Moving Through the Dungeon The program must keep track of the current room which is the room which the player is currently in. The player will enter compass direction commands to move from one room to another. Your program should accept the following commands related to movement: 1. north: This command moves the player into the room to the north of the current room. If there is a room to the north then the description of the new room is printed. If there is no room to the north then the message, "You can't go there." is printed to the screen. 2. south: This command moves the player into the room to the south of the current room. If there is a room to the south then the description of the new room is printed. If there is no room to the south then the message, "You can't go there." is printed to the screen. LEWE east: This command moves the player into the room to the east of the current room. If there is a to the room to the east then the description of the new room is printed. If there is no room to the east then the message, "You can't go there." is printed to the screen. 4. west: This command moves the player into the room to the west of the current room. If there is a room to the west then the description of the new room is printed. If there is no room to the west then the message, "You can't go there." is printed to the screen. 3.
ICS 31, Summer Session 10-wk, 2022 Assignment 5 You will write a text adventure game. The idea of a text adventure game is that the player is in a virtual room in a "dungeon", and each room has a text description associated with it, such as, "This room in big and brightly lit. It has doors on the north and west walls". The player can move from room room using compass directional commands. Your program will be a function called adventure () which takes no arguments and returns no values. Your function adventure () will allow the user to enter commands related to moving through the dungeon and interacting with objects. Your function should print out a prompt to indicate that the user can enter a command. Your prompt should be a '$' character. At the prompt the user will type a command followed by a set of arguments for the command. Your function will perform whatever action is indicated by the command, and then your program will print a prompt on a new line in order to let the user know that he/she can type a new command. If the command is a compass command which moves the player into a new room, your program will print the description of the new room before printing the prompt on the next line. Dungeon File Each time a user plays the adventure game, he will be able to choose a dungeon to play in. The rooms in the dungeon, and their arrangement, is stored in a dungeon file. When the game starts, the player will have to issue a command to load a dungeon file describing the dungeon. The dungeon file will be a text file containing information about each room in a specific format. Each line of the file will contain information about a single room in the dungeon. Each room is associated with the following 6 fields of information. 1. Room number. This is a positive integer which uniquely identifies the room. 2. Description: This is a string which is printed when the player enters the room. This string is delimited by '+' symbols rather than the regular quotes. 3. North room: This is the room number of the room immediately to the north of this room. If there is no room to the north of this room then this value is -1. 4. South room: This is the room number of the room immediately to the south of this room. If there is no room to the south of this room then this value is -1. 5. East room: This is the room number of the room immediately to the east of this room. If there is no room to the east of this room then this value is -1. 6. West room: This is the room number of the room immediately to the west of this room. If there. is no room to the west of this room then this value is -1. All 6 fields of information about each room are contained in order on a single line in the dungeon file. Each field of information is separated by one or more spaces. The room described on the first line of the file is the initial room where the player will start after the dungeon file is loaded. Here is an example dungeon file. Be aware that this is only an example and that your code must work. with any valid dungeon file having any file name.. 1 This is an open field west of a white house, with a boarded front door. 2 -1 -1 -1 2 +North of House: You are facing the north side of a white house.+-1 1 3 -1 3 +Behind House: You are behind the white house.+ -1 4-1 2 4 South of House: You are facing the south side of a white house.+ 3 -1 -1 -1 Your program should accept the following command related to dungeon files: 1. loaddungeon: This command reads a dungeon file, allowing the player to explore the dungeon. The function takes one argument, the name of the dungeon file. The function returns no values. After executing this command the player should be in the room described on the first line of the dungeon file, and the description of the first room should be printed. The loaddungeon command can only be issued once. If the player tries to issue the command a second time, the error "Dungeon already loaded" should be printed. Moving Through the Dungeon The program must keep track of the current room which is the room which the player is currently in. The player will enter compass direction commands to move from one room to another. Your program should accept the following commands related to movement: 1. north: This command moves the player into the room to the north of the current room. If there is a room to the north then the description of the new room is printed. If there is no room to the north then the message, "You can't go there." is printed to the screen. 2. south: This command moves the player into the room to the south of the current room. If there is a room to the south then the description of the new room is printed. If there is no room to the south then the message, "You can't go there." is printed to the screen. LEWE east: This command moves the player into the room to the east of the current room. If there is a to the room to the east then the description of the new room is printed. If there is no room to the east then the message, "You can't go there." is printed to the screen. 4. west: This command moves the player into the room to the west of the current room. If there is a room to the west then the description of the new room is printed. If there is no room to the west then the message, "You can't go there." is printed to the screen. 3.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Python3
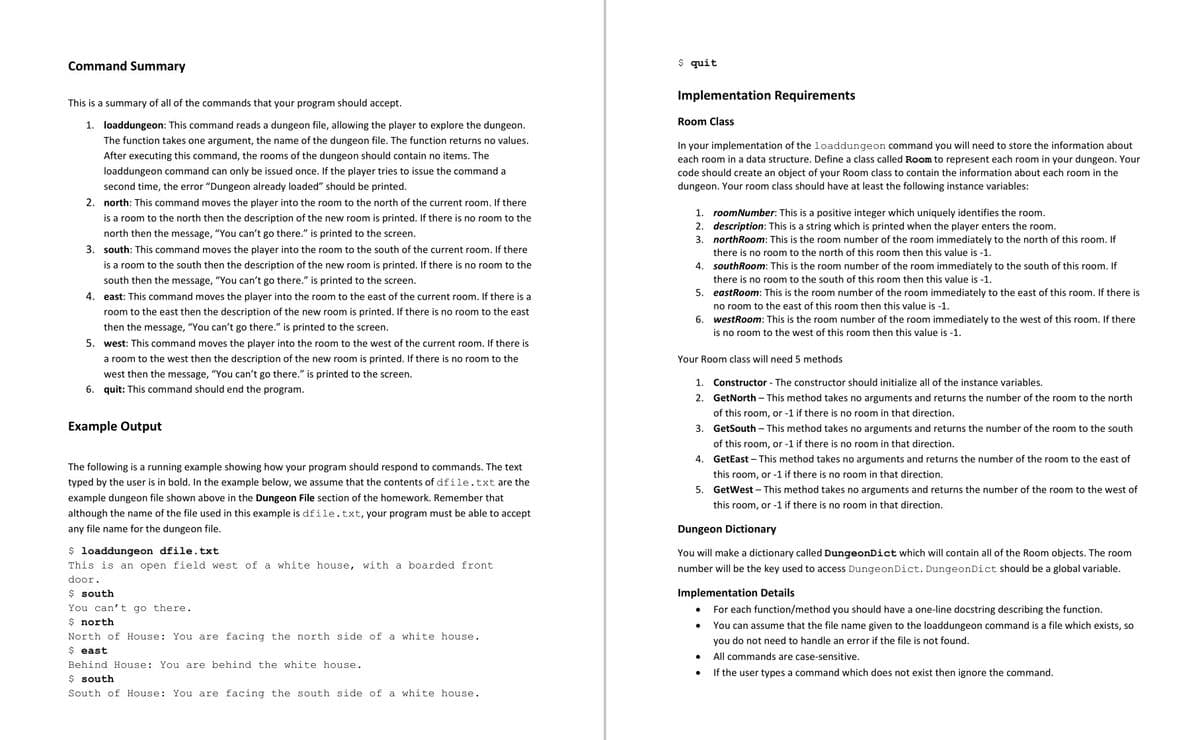
Transcribed Image Text:Command Summary
This is a summary of all of the commands that your program should accept.
1. loaddungeon: This command reads a dungeon file, allowing the player to explore the dungeon.
The function takes one argument, the name of the dungeon file. The function returns no values.
After executing this command, the rooms of the dungeon should contain no items. The
loaddungeon command can only be issued once. If the player tries to issue the command a
second time, the error "Dungeon already loaded" should be printed.
2. north: This command moves the player into the room to the north of the current room. If there
is a room to the north then the description of the new room is printed. If there is no room to the
north then the message, "You can't go there." is printed to the screen.
3. south: This command moves the player into the room to the south of the current room. If there
is a room to the south then the description of the new room is printed. If there is no room to the
south then the message, "You can't go there." is printed to the screen.
4. east: This command moves the player into the room to the east of the current room. If there is a
room to the east then the description of the new room is printed. If there is no room to the east
then the message, "You can't go there." is printed to the screen.
5. west: This command moves the player into the room to the west of the current room. If there is
a room to the west then the description of the new room is printed. If there is no room to the
west then the message, "You can't go there." is printed to the screen.
6. quit: This command should end the program.
Example Output
The following is a running example showing how your program should respond to commands. The text
typed by the user is in bold. In the example below, we assume that the contents of dfile.txt are the
example dungeon file shown above in the Dungeon File section of the homework. Remember that
although the name of the file used in this example is dfile.txt, your program must be able to accept
any file name for the dungeon file.
$ loaddungeon dfile.txt
This is an open field west of a white house, with a boarded front
door.
$ south
You can't go there.
$ north
North of House: You are facing the north side of a white house.
$ east.
Behind House: You are behind the white house.
$ south
South of House: You are facing the south side of a white house.
$ quit
Implementation Requirements
Room Class
In your implementation of the loaddungeon command you will need to store the information about
each room in a data structure. Define a class called Room to represent each room in your dungeon. Your
code should create an object of your Room class to contain the information about each room in the
dungeon. Your room class should have at least the following instance variables:
1. roomNumber: This is a positive integer which uniquely identifies the room.
2. description: This is a string which is printed when the player enters the room.
3. northRoom: This is the room number of the room immediately to the north of this room. If
there is no room to the north this room then this value is -1.
4.
5.
6.
westRoom: This is the room number of the room immediately to the west of this room. If there
is no room to the west of this room then this value is -1.
southRoom: This is the room number of the room immediately to the south of this room. If
there is no room to the south of this room then this value is -1.
Your Room class will need 5 methods
eastRoom: This is the room number of the room immediately to the east of this room. If there is
no room to the east of this room. hen this value is -1.
1. Constructor - The constructor should initialize all of the instance variables.
2. GetNorth This method takes no arguments and returns the number of the room to the north
of this room, or -1 if there is no room in that direction.
3. GetSouth - This method takes no arguments and returns the number of the room to the south
of this room, or -1 if there is no room in that direction.
4. GetEast - This method takes no arguments and returns the number of the room to the east of
this room, or -1 if there is no room in that direction.
5. GetWest - This method takes no arguments and returns the number of the room to the west of
this room, or -1 if there is no room in that direction.
Dungeon Dictionary
You will make a dictionary called DungeonDict which will contain all of the Room objects. The room
number will be the key used to access Dungeon Dict. DungeonDict should be a global variable.
●
Implementation Details
For each function/method you should have a one-line docstring describing the function.
You can assume that the file name given to the loaddungeon command is a file which exists, so
you do not need to handle an error if the file is not found.
● All commands are case-sensitive.
If the user types a command which does not exist then ignore the command.
●
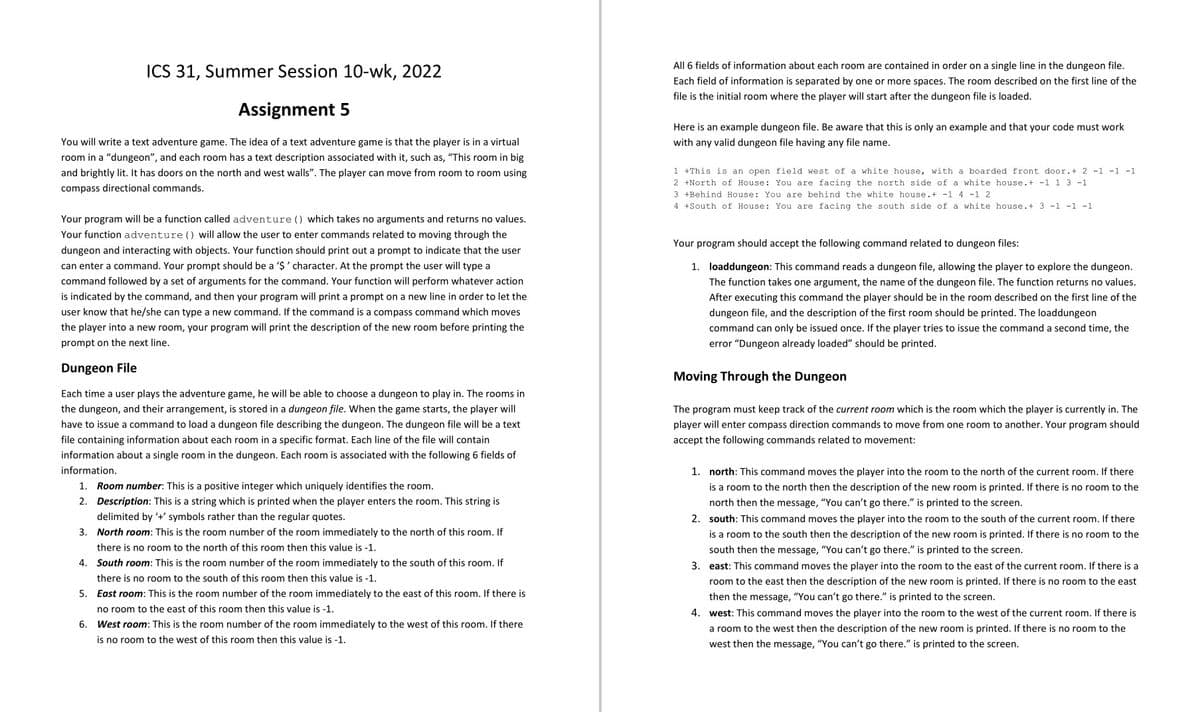
Transcribed Image Text:ICS 31, Summer Session 10-wk, 2022
Assignment 5
You will write a text adventure game. The idea of a text adventure game is that the player is in a virtual
room in a "dungeon", and each room has a text description associated with it, such as, "This room in big
and brightly lit. It has doors on the north and west walls". The player can move from room to room using
compass directional commands.
Your program will be a function called adventure () which takes no arguments and returns no values.
Your function adventure () will allow the user to enter commands related to moving through the
dungeon and interacting with objects. Your function should print out a prompt to indicate that the user
can enter a command. Your prompt should be a '$' character. At the prompt the user will type a
command followed by a set of arguments for the command. Your function will perform whatever action
is indicated by the command, and then your program will print a prompt on a new line in order to let the
user know that he/she can type a new command. If the command is a compass command which moves
the player into a new room, your program will print the description of the new room before printing the
prompt on the next line.
Dungeon File
Each time a user plays the adventure game, he will be able to choose a dungeon to play in. The rooms in
the dungeon, and their arrangement, is stored in a dungeon file. When the game starts, the player will
have to issue a command to load a dungeon file describing the dungeon. The dungeon file will be a text
file containing information about each room in a specific format. Each line of the file will contain
information about a single room in the dungeon. Each room is associated with the following 6 fields of
information.
1. Room number: This is a positive integer which uniquely identifies the room.
2. Description: This is a string which is printed when the player enters the room. This string is
delimited by '+' symbols rather than the regular quotes.
North room: This is the room number of the room immediately to the north of this room. If
there is no room to the north of this room then this value is -1.
3.
4. South room: This is the room number of the room immediately to the south of this room. If
there is no room to the south of this room then this value is -1.
5. East room: This is the room number of the room immediately to the east of this room. If there is
no room to the east of this room then this value is -1.
6. West room: This is the room number of the room immediately to the west of this room. If there
is no room to the west of this room then this value is -1.
All 6 fields of information about each room are contained in order on a single line in the dungeon file.
Each field of information is separated by one or more spaces. The room described on the first line of the
file is the initial room where the player will start after the dungeon file is loaded.
Here is an example dungeon file. Be aware that this is only an example and that your code must work
with any valid dungeon file having any file name.
1 +This is an open field west of a white house, with a boarded front door.+ 2 -1 -1 -1
2 +North of House: You are facing the north side of a white house. + -1 1 3 -1
3 +Behind House: You are behind the white house.+ -1 4-1 2
4 +South of House: You are facing the south side of a white house.+ 3 -1 -1 -1
Your program should accept the following command related to dungeon files:
1. loaddungeon: This command reads a dungeon file, allowing the player to explore the dungeon.
The function takes one argument, the name of the dungeon file. The function returns no values.
After executing this command the player should be in the room described on the first line of the
dungeon file, and the description of the first room should be printed. The loaddungeon
command can only be issued once. If the player tries to issue the command a second time, the
error "Dungeon already loaded" should be printed.
Moving Through the Dungeon
The program must keep track of the current room which is the room which the player is currently in. The
player will enter compass direction commands to move from one room to another. Your program should
accept the following commands related to movement:
1. north: This command moves the player into the room to the north of the current room. If there
is a room to the north then the description of the new room is printed. If there is no room to the
north then the message, "You can't go there." is printed to the screen.
2. south: This command moves the player into the room to the south of the current room. If there
is a room to the south then the description of the new room is printed. If there is no room to the
south then the message, "You can't go there." is printed to the screen.
3. east: This command moves the player into the room to the east of the current room. If there is a
room to the east then the description of the new room is printed. If there is no room to east
then the message, "You can't go there." is printed to the screen.
4. west: This command moves the player into the room to the west of the current room. If there is
a room to the west then the description of the new room is printed. If there is no room to the
west then the message, "You can't go there." is printed to the screen.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Recommended textbooks for you
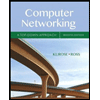
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
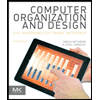
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
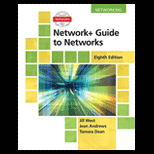
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
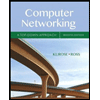
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
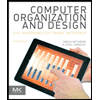
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
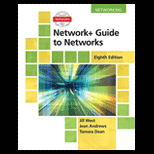
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
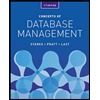
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
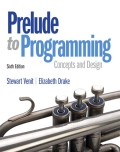
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
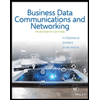
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY