IF POSSIBLE EXPLAIN EACH LINE, It doesn't have to be long, as long as you explain what the important parts of your code do. #include #include "linkedlist.h" /** * This activity is focused on using Arrays and Linked Lists as two different * implementations of List. It follows that you are only to use the methods of * List and not of the specific array or linkedlist. */ int main(void) { // WARNING! Do not modify this line up until line 22! // Doing so will nullify your score for this activity. // Also, do not create more instances of ArrayList or LinkedList // aside from those in lines 19 and 21. char li; cin >> li; List* list; if (li == 'A') { list = new ArrayList(); } else { list = new LinkedList(); } char sym; int arg1; int arg2; int count; int sum; // You may want to insert a loop here do{ cin >> sym; switch (sym) { case '?': cin >> arg1; cout << list->get(arg1) << endl; break; case '+': cin >> arg1; list->add(arg1); break; case '-': cin >> arg1; cout << list->removeAt(arg1) << endl; break; case '\'': cin >> arg1; cout << list->remove(arg1) << endl; break; case '\"': cin >> arg1; count = 0; while (list->remove(arg1) != 0){ count++; } cout <> arg1 >> arg2; list->addAt(arg1,arg2); break; case '$': cout << list->size() << endl; break; case '<': cin >> arg1; if (arg1 < list->size()){ for (int i=1; i<=arg1; i++){ list->removeAt(1); } } break; case '>': cin >> arg1; if (arg1 <= list->size()){ for (int i=1; i<=arg1; i++){ list->removeAt(list->size()); } } break; case '*': cin >> arg1 >> arg2; if (arg1 <= list->size() && arg2 <= list->size()){ cout << list->get(arg1) * list->get(arg2) << endl; } break; case '^': cin >> arg1 >> arg2; if (arg1 <= list->size() && arg2 <= list->size()){ if(list->get(arg1) > list->get(arg2)){ cout << list->get(arg1) << endl; }else{ cout << list->get(arg2) << endl; } } break; case '!': cin >> arg1 >> arg2; if (arg1 <= list->size() && arg2 <= list->size()){ cout << list->get(arg2) - list->get(arg1) << endl; } break; case '=': sum = 0; for (int i=1; i<= list->size(); i++){ sum += list->get(i); } cout << sum << endl; break; case '#': list->print(); break; } } while (sym != '/'); return 0; }
IF POSSIBLE EXPLAIN EACH LINE, It doesn't have to be long, as long as you explain what the important parts of your code do.
#include <iostream>
#include "linkedlist.h"
/**
* This activity is focused on using Arrays and Linked Lists as two different
* implementations of List. It follows that you are only to use the methods of
* List and not of the specific array or linkedlist.
*/
int main(void) {
// WARNING! Do not modify this line up until line 22!
// Doing so will nullify your score for this activity.
// Also, do not create more instances of ArrayList or LinkedList
// aside from those in lines 19 and 21.
char li;
cin >> li;
List* list;
if (li == 'A') {
list = new ArrayList();
} else {
list = new LinkedList();
}
char sym;
int arg1;
int arg2;
int count;
int sum;
// You may want to insert a loop here
do{
cin >> sym;
switch (sym) {
case '?':
cin >> arg1;
cout << list->get(arg1) << endl;
break;
case '+':
cin >> arg1;
list->add(arg1);
break;
case '-':
cin >> arg1;
cout << list->removeAt(arg1) << endl;
break;
case '\'':
cin >> arg1;
cout << list->remove(arg1) << endl;
break;
case '\"':
cin >> arg1;
count = 0;
while (list->remove(arg1) != 0){
count++;
}
cout <<count << endl;
break;
case '@':
cin >> arg1 >> arg2;
list->addAt(arg1,arg2);
break;
case '$':
cout << list->size() << endl;
break;
case '<':
cin >> arg1;
if (arg1 < list->size()){
for (int i=1; i<=arg1; i++){
list->removeAt(1);
}
}
break;
case '>':
cin >> arg1;
if (arg1 <= list->size()){
for (int i=1; i<=arg1; i++){
list->removeAt(list->size());
}
}
break;
case '*':
cin >> arg1 >> arg2;
if (arg1 <= list->size() && arg2 <= list->size()){
cout << list->get(arg1) * list->get(arg2) << endl;
}
break;
case '^':
cin >> arg1 >> arg2;
if (arg1 <= list->size() && arg2 <= list->size()){
if(list->get(arg1) > list->get(arg2)){
cout << list->get(arg1) << endl;
}else{
cout << list->get(arg2) << endl;
}
}
break;
case '!':
cin >> arg1 >> arg2;
if (arg1 <= list->size() && arg2 <= list->size()){
cout << list->get(arg2) - list->get(arg1) << endl;
}
break;
case '=':
sum = 0;
for (int i=1; i<= list->size(); i++){
sum += list->get(i);
}
cout << sum << endl;
break;
case '#':
list->print();
break;
}
}
while (sym != '/');
return 0;
}

Step by step
Solved in 2 steps

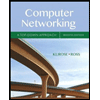
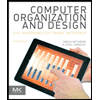
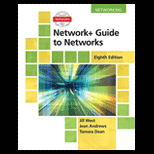
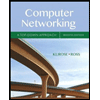
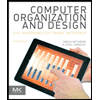
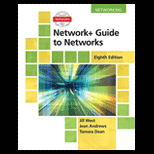
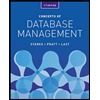
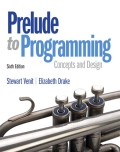
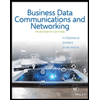