I'm getting an error on the output. How do I get it to print: The gas cost for 10 miles is $1.58 The gas cost for 50 miles is $7.90 The gas cost for 400 miles is $63.2
I'm getting an error on the output. How do I get it to print: The gas cost for 10 miles is $1.58 The gas cost for 50 miles is $7.90 The gas cost for 400 miles is $63.2
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section: Chapter Questions
Problem 8PP: (Statistical) In many statistical analysis programs, data values considerably outside the range of...
Related questions
Question
I'm getting an error on the output. How do I get it to print:
The gas cost for 10 miles is $1.58
The gas cost for 50 miles is $7.90
The gas cost for 400 miles is $63.20

Transcribed Image Text:Create a function to match the specifications
• Use floating-point value division
• Write a program to get the user input and call the custom function and produce the desired output using a
format function to output the specified precision
Instructions
Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and
output the gas cost for 10 miles, 50 miles, and 400 miles.
Output each floating-point value with two digits after the decimal point, which can be achieved as follows:
print('{:.2f}'.format(your_value))
Ex: If the input to your program is:
20.0
3.1599
the output is:
The gas cost for 10 miles is $1.58
The gas cost for 50 miles is $7.90
The gas cost for 400 miles is $63.20
Your program must define and call the driving_cost() function. Given input parameters driven_miles,
miles_per_gallon, and dollars_per_gallon, the function returns the dollar cost to drive those miles.
Ex: If the function is called with:

Transcribed Image Text:50
20.0
3.1599
the function returns:
7.89975
def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon)
Your program should call the function three times to determine the gas cost for 10 miles, 50 miles, and 400
miles.
Hint: To calculate how many gallons I will spend to drive 1 mile I will divide 1 by a car's miles/gallon parameter.
Note: Pay attention to the distinction of what the function expects and is supposed to do versus what the
program needs to collect and do. In this lab, the function has 3 parameters, whereas the program collects only
2 input values because the miles are already pre-determined (i.e., hard-coded) inside of its code.
354684.2275504.qx3zgy7
LAB
5.16.1: LAB: Driving costs - functions
0/ 10
АCTIVITY
main.py
Load default template...
1 # Define your function here.
2 def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon):
3
return (driven_miles/miles_per_gallon)*dollars_per_gallon
4
5 if
name
== ' main ':
6
# Type your code here.
miles_per_gallon = float(input())
dollars_per_gallon = float(input())
8
9
10
11 print('{:.2f}'.format(driving_cost(10, miles_per_gallon, dollars_per_gallon)))
12 print('{:.2f}'.format(driving_cost(50, miles_per_gallon, dollars_per_gallon)))
13 print('{:.2f}'.format(driving_cost(400, miles_per_gallon, dollars_per_gallon)))
14
15
16
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
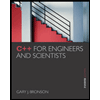
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
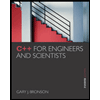
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr