Implement the algorithms in C++ program. Algorithm 1: (Traversing a Linked List) Let LIST be a linked list in memory. This algorithm traverses LIST, applying an operation PROCESS to each Node/element of the LIST. HEAD points to the first node of the LIST. Pointer variable CURR points to the node currently being processed. Step 1. Set CURR = HEAD. [Initializes pointer CURR.] Step 2. Repeat Steps 3 and 4 while CURR ≠ NULL. Step 3. Apply PROCESS to CURR -> INFO. Step 4. Set CURR = CURR -> NEXT [CURR now points to the next node.] [End of Step-2 loop.] Step 5. End Algorithm2: INSERT( ITEM) NOTE: Here it is needed that all the time, LIST should remains in the sorted order using Info part of the Node. To Insert / Enqueue a new node in the LIST, first LIST will be searched (using Info of Nodes) to find its LOCATION ( where to insert new Node). This exercise makes it possible that every new node has the probability to insert at the beginning, in the middle or at the end of LIST. CURR is a pointer points to the node currently being processed and PREV is a pointer pointing to previous node of the current node. [This algorithm adds NewNodes at any position (Top, in Middle OR at End) in the List ] Step 1. Create NewNode node for LIST in memory Step 2. Set NewNode -> Info =ITEM. [Copies new data into INFO of new node.] Step 3. NewNode -> Next = NULL. [Copies NULL in NEXT of new node.]. Step 4. If HEAD = NULL then: [ Condition to create the Linked List. ] HEAD=NewNode and return. [Add first node in list] [Add on top of existing list] Step 6. If NewNode-> Info < HEAD ->Info then: Set NewNode->NEXT = HEAD and HEAD = NewNode and return[Search the LOCATION in Middle or at end. ] [ Here CURR is the position where to insert and PREV points to previous node of the CURR node ] Step 7. Set Prev = NULL and Set Curr = NULL; Step 8. Repeat for CURR =HEAD until CURR ≠ NULL after iteration CURR = CURR ->Next if(NewNode->Info <= CURR ->Info) then: break the loop Set PREV = CURR; [ end of loop of step-7 ] [Insert after PREV node (in middle or at end) of the list] Step 9. Set NewNode->NEXT = PREV ->NEXT and Step 10. Set PREV ->NEXT= NewNode. Step 11. Exit.
Implement the algorithms in C++ program.
Step 1. Set CURR = HEAD. [Initializes pointer CURR.]
Step 2. Repeat Steps 3 and 4 while CURR ≠ NULL.
Step 3. Apply PROCESS to CURR -> INFO.
Step 4. Set CURR = CURR -> NEXT [CURR now points to the next node.] [End of Step-2 loop.]
Step 5. End
Algorithm2: INSERT( ITEM)
NOTE: Here it is needed that all the time, LIST should remains in the sorted order using Info part of the Node. To Insert / Enqueue a new node in the LIST, first LIST will be searched (using Info of Nodes) to find its LOCATION ( where to insert new Node). This exercise makes it possible that every new node has the probability to insert at the beginning, in the middle or at the end of LIST. CURR is a pointer points to the node currently being processed and PREV is a pointer pointing to previous node of the current node. [This algorithm adds NewNodes at any position (Top, in Middle OR at End) in the List ]
Step 1. Create NewNode node for LIST in memory
Step 2. Set NewNode -> Info =ITEM. [Copies new data into INFO of new node.]
Step 3. NewNode -> Next = NULL. [Copies NULL in NEXT of new node.].
Step 4. If HEAD = NULL then: [ Condition to create the Linked List. ]
HEAD=NewNode and return. [Add first node in list] [Add on top of existing list]
Step 6. If NewNode-> Info < HEAD ->Info then:
Set NewNode->NEXT = HEAD and HEAD = NewNode and return[Search the LOCATION in Middle or at end. ] [ Here CURR is the position where to insert and PREV points to previous node of the CURR node ]
Step 7. Set Prev = NULL and Set Curr = NULL;
Step 8. Repeat for CURR =HEAD until CURR ≠ NULL after iteration CURR = CURR ->Next if(NewNode->Info <= CURR ->Info) then: break the loop
Set PREV = CURR;
[ end of loop of step-7 ]
[Insert after PREV node (in middle or at end) of the list]
Step 9. Set NewNode->NEXT = PREV ->NEXT and
Step 10. Set PREV ->NEXT= NewNode.
Step 11. Exit.

Step by step
Solved in 3 steps with 1 images

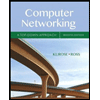
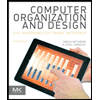
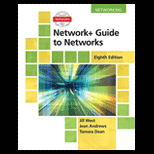
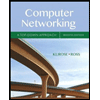
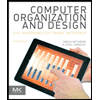
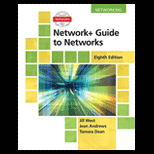
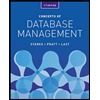
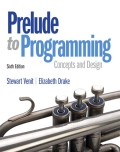
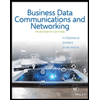