Implement the plus_one function as directed in the comment above the function. If the directions are unclear, run the code, and look at the expected answer for a few inputs. This function should work regardless of whether x is negative or not, and you should not use the ‘+’ symbol anywhere in your solution, nor any constants. To find the solution to this task, you should use your understanding of integer operations to find a way to add 1. Hint: consider what happens when you do -x. Could you use it with other operations to get x+1?.
Implement the plus_one function as directed in the comment above the function. If the directions are unclear, run the code, and look at the expected answer for a few inputs. This function should work regardless of whether x is negative or not, and you should not use the ‘+’ symbol anywhere in your solution, nor any constants. To find the solution to this task, you should use your understanding of integer operations to find a way to add 1.
Hint: consider what happens when you do -x. Could you use it with other operations to get x+1?.
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
// BEGIN STRINGBUILDER IMPLEMENTATION
// This code is a very rudimentary stringbuilder-like implementation
// To create a new stringbuilder, use the following line of code
//
// stringbuilder sb = new_sb();
//
// If you want to append a character to the stringbuilder, use the
// following line of code. Replace whatever character you want to
// append where the 'a' is.
//
// sb_append_char(sb, 'a');
//
// Though there are some other functions that might be useful to you,
// the driver code provided uses the functions, so there is no need
// to use them manually.
typedef struct {
char** cars;
size_t* len;
size_t* alloc_size;
} stringbuilder;
stringbuilder new_sb() {
stringbuilder sb;
sb.cars = malloc(sizeof(char*));
*sb.cars = malloc(8*sizeof(char));
(*sb.cars)[0] = 0;
sb.len = malloc(sizeof(size_t));
*sb.len = 0;
sb.alloc_size = malloc(sizeof(size_t));
*sb.alloc_size = 8;
return sb;
}
void sb_append(stringbuilder sb, char a) {
int len = *sb.len;
if (len >= (*sb.alloc_size)-1) {
*sb.alloc_size = (*sb.alloc_size)*2;
char* newcars = malloc((*sb.alloc_size)*sizeof(char));
for (int i = 0; i < *sb.len; i++) {
newcars[i] = (*sb.cars)[i];
}
free(*sb.cars);
(*sb.cars) = newcars;
}
(*sb.cars)[len] = a;
len++;
(*sb.cars)[len] = 0;
*sb.len = len;
}
void delete_sb(stringbuilder sb) {
free(*sb.cars);
free(sb.cars);
free(sb.len);
free(sb.alloc_size);
}
bool sb_is_equal(stringbuilder sb1, stringbuilder sb2) {
if (*sb1.len != *sb2.len)
return false;
for (int i = 0; i < *sb1.len; i++) {
if ((*sb1.cars)[i] != (*sb2.cars)[i])
return false;
}
return true;
}
void print_sb(const stringbuilder sb) {
printf("%s", *sb.cars);
}
// END STRINGBUILDER IMPLEMENTATION
// ============================================================
// Write your solutions to the tasks below
const unsigned UNS_MAX = -1; // 1111...
const unsigned UNS_MIN = 0; // 0000...
const int INT_MAX = UNS_MAX >> 1; // 0111...
const int INT_MIN = ~INT_MAX; // 1000...
// Task 1
// For this function, you must return an integer holding the value
// x+1, however you may not use any constants or the symbol '+'
// anywhere in your solution. This means that
//
// return x - (-1);
//
// is not a valid soltion, because it uses the constant -1.
//
// Hint: Consider what internally happens when you do -x.
int plus_one(int x) {
return x;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

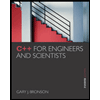
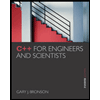