import bridges.base.ColorGrid; import bridges.base.Color; public class Scene {
import bridges.base.ColorGrid;
import bridges.base.Color;
public class Scene {
/* Creates a Scene with a maximum capacity of Marks and
with a background color.
maxMarks: the maximum capacity of Marks
backgroundColor: the background color of this Scene
*/
public Scene(int maxMarks, Color backgroundColor) {
}
// returns true if the Scene has no room for additional Marks
private boolean isFull() {
return false;
}
/* Adds a Mark to this Scene. When drawn, the Mark
will appear on top of the background and previously added Marks
m: the Mark to add
*/
public void addMark(Mark m) {
if (isFull()) throw new IllegalStateException("No room to add more Marks");
}
/*
Helper method: deletes the Mark at an index.
If no Marks have been previously deleted, the method
deletes the ith Mark that was added (0 based).
i: the index
*/
protected void deleteMark(int i) {
}
/*
Deletes all Marks from this Scene that
have a given Color
c: the Color
*/
public void deleteMarksByColor(Color c) {
}
/* draws the Marks in this Scene over a background color
onto a ColorGrid. Marks will appear on top of the
background, and Marks will overlap other Marks if
they were added by a more recent call to addMark.
cg: the ColorGrid to draw on
*/
public void draw(ColorGrid cg) {
}
};
import bridges.base.Color;
import bridges.base.ColorGrid;
import org.junit.Test;
public class SceneTest {
private static final Color Y = new Color("yellow");
private static final Color B = new Color("black");
private static final Color G = new Color("green");
private static final Color C = new Color("cyan");
@Test
public void backgroundTest() {
Scene s = new Scene(2, Y);
ColorGrid cg = new ColorGrid(3, 3);
s.draw(cg);
TestUtilities.checkCG(new Color[][]{
{Y, Y, Y},
{Y, Y, Y},
{Y, Y, Y}}, cg);
}
@Test
public void multipleScenesTest() {
Scene s = new Scene(2, Y);
Scene s2 = new Scene(3, C);
ColorGrid cg = new ColorGrid(3, 3);
ColorGrid cg2 = new ColorGrid(2, 3);
s.draw(cg);
s2.draw(cg2);
TestUtilities.checkCG(new Color[][]{
{Y, Y, Y},
{Y, Y, Y},
{Y, Y, Y}}, cg);
TestUtilities.checkCG(new Color[][]{
{C, C, C},
{C, C, C}}, cg2);
}
@Test
public void pointTest() {
Scene s = new Scene(2, B);
s.addMark(new Point(1, 2, Y));
ColorGrid cg = new ColorGrid(3, 3);
s.draw(cg);
TestUtilities.checkCG(new Color[][]{
{B, B, B},
{B, B, B},
{B, Y, B}}, cg);
}
@Test
public void pointsTest() {
Scene s = new Scene(2, B);
s.addMark(new Point(1, 2, Y));
s.addMark(new Point(0, 2, G));
ColorGrid cg = new ColorGrid(3, 3);
s.draw(cg);
TestUtilities.checkCG(new Color[][]{
{B, B, B},
{B, B, B},
{G, Y, B}}, cg);
}
@Test
public void multipleDrawsTest() {
Scene s = new Scene(4, G);
s.addMark(new Point(0, 0, Y));
ColorGrid cg = new ColorGrid(1, 3);
s.draw(cg);
TestUtilities.checkCG(new Color[][]{{Y, G, G}}, cg);
s.addMark(new Point(1, 0, C));
s.addMark(new Point(0, 0, B));
s.draw(cg);
TestUtilities.checkCG(new Color[][]{{B, C, G}}, cg);
}
@Test
public void multipleScenesPointsTest() {
Scene s = new Scene(2, B);
Scene s2 = new Scene(1, C);
s.addMark(new Point(1, 2, Y));
s2.addMark(new Point(0, 2, G));
ColorGrid cg = new ColorGrid(3, 3);
s.draw(cg);
ColorGrid cg2 = new ColorGrid(3, 1);
s2.draw(cg2);
TestUtilities.checkCG(new Color[][]{
{B, B, B},
{B, B, B},
{B, Y, B}}, cg);
TestUtilities.checkCG(new Color[][]{
{C},
{C},
{G}}, cg2);
}
@Test
public void pointAndLineTest() {
Scene s = new Scene(2, B);
s.addMark(new Point(1, 2, Y));
s.addMark(new HorizontalLine(0, 1, 0, G));
ColorGrid cg = new ColorGrid(3, 3);
s.draw(cg);
TestUtilities.checkCG(new Color[][]{
{G, G, B},
{B, B, B},
{B, Y, B}}, cg);
}
// This test passes if the code throws the expected
// exception. It fails if the code finishes with no exception.
@Test(expected = IllegalStateException.class)
public void exceededCapacityTest() {
Scene s = new Scene(3, Y);
s.addMark(new Point(0, 0, G));
s.addMark(new Point(0, 0, G));
s.addMark(new Point(0, 0, G));
s.addMark(new Point(0, 0, G));
}
@Test
public void deleteFrontTest() {
Scene s = new Scene(3, Y);
s.addMark(new Point(0, 0, B));
s.addMark(new Point(1, 1, G));
s.addMark(new Point(2, 2, C));
s.deleteMark(0);
ColorGrid cg = new ColorGrid(3,3);
s.draw(cg);
TestUtilities.checkCG(new Color[][]{
{Y, Y, Y},
{Y, G, Y},
{Y, Y, C}}, cg);
}
@Test
public void deleteMidTest() {
Scene s = new Scene(5, Y);
s.addMark(new Point(0, 0, B));
s.addMark(new Point(1, 1, G));
s.addMark(new Point(2, 2, C));
s.addMark(new Point(0, 1, B));
s.deleteMark(1);
ColorGrid cg = new ColorGrid(3,3);
s.draw(cg);
TestUtilities.checkCG(new Color[][]{
{B, Y, Y},
{B, Y, Y},
{Y, Y, C}}, cg);
}
@Test
public void addAfterDeleteTest() {
Scene s = new Scene(4, Y);
s.addMark(new Point(0, 0, B));
s.addMark(new Point(1, 1, G));
s.addMark(new Point(2, 2, C));
s.addMark(new Point(0, 1, B));
s.deleteMark(1);
s.addMark(new Point(2, 1, B));
ColorGrid cg = new ColorGrid(3,3);
s.draw(cg);
TestUtilities.checkCG(new Color[][]{
{B, Y, Y},
{B, Y, B},
{Y, Y, C}}, cg);
}
![179
@Test
public void deleteEndTest (0 {
Scene s = new Scene( maxMarks: 5, Y);
s.addMark(new Point( x 0,
s.addMark(new Point( x 1,
s.addMark(new Point( x 2,
s.addMark(new Point( x 0,
s.deleteMark( 3);
ColorGrid cg = new ColorGrid( rows: 3, cols: 3);
s.draw(cg);
TestUtilities.checkCG(new Color[][]{
180
181
182
y: 0, B));
y: 1, G));
y: 2, C));
у 1, В));
183
184
185
186
187
188
189
190
{в, Y, V},
{Y, G, Y},
{Y, Y, C}}, cg);
191
192
193
194
195
@Test
public void occlusionTest(0 {
Scene s = new Scene( maxMarks: 5, G);
s.addMark(new Horizontalline( start: 0,
s.addMark(new Verticalline( start: 0,
196
197
198
end: 2, y 0, Y));
end: 2
end: 1,
199
x 1, B));
x 2, B));
200
s.addMark(new Verticalline( start 0,
ColorGrid cg = new ColorGrid( rows: 3, cols: 3);
s.draw (cg);
Testutilities.checkCG(new Color[][]{
201
202
203
204
{Y, B, B},
{G, B, B},
{G, B, G}}, cg);
205
206
207
208
209
@Test
public void deleteByColorTest() {
Scene s = new Scene( maxMarks: 5, G);
s.addMark(new Verticalline( start 0,
s.addMark(new HorizontalLine( start 0,
210
211
212
x 1, B));
end: 2,
end: 2, y 0, Y));
end: 1, x 2, B));
213
214
215
s.addMark(new Verticalline( start 0,
216
ColorGrid cg = new ColorGrid( rows: 3, cols: 3);
217
s.deleteMarksByColor(B);
218
s.draw(cg);
TestUtilities.checkCG(new Color[][]{
{Y, Y, Y},
{G, G, G},
{G, G, G}}, cg);
219
220
221
222
223
224](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F43d990fb-9cc0-47b0-a7c8-1fe0440662e3%2Fa714d7ee-d8d0-4569-abac-2fd23c5dae44%2F46knqs9_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

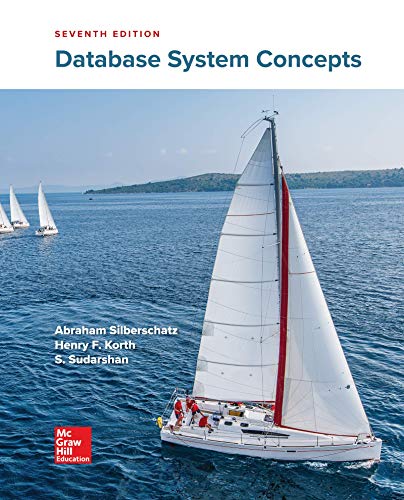
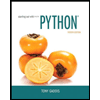
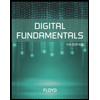
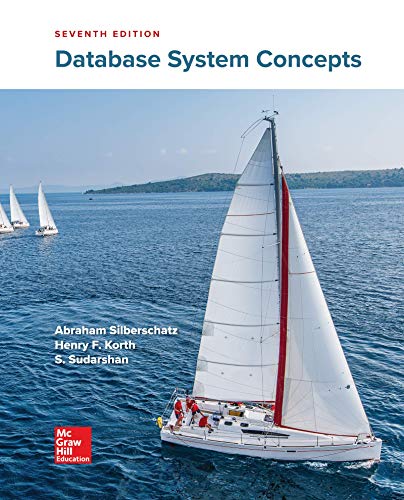
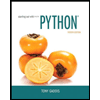
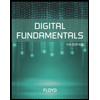
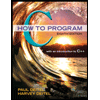
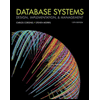
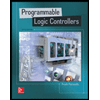