import cv2 import sys import logging as log import datetime as dt from time import sleep cascPath = "haarcascade_frontalface_default.xml" faceCascade = cv2.CascadeClassifier(cascPath) log.basicConfig(filename='webcam.log',level=log.INFO) video_capture = cv2.VideoCapture(0) anterior = 0 while True: if not video_capture.isOpened(): print('Unable to load camera.') sleep(5) pass # Capture frame-by-frame ret, frame = video_capture.read() gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) faces = faceCascade.detectMultiScale( gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30) ) # Draw a rectangle around the faces for (x, y, w, h) in faces: cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2) if anterior != len(faces): anterior = len(faces) log.info("faces: "+str(len(faces))+" at "+str(dt.datetime.now())) # Display the resulting frame cv2.imshow('Video', frame) if cv2.waitKey(1) & 0xFF == ord('s'): check, frame = video_capture.read() cv2.imshow("Capturing", frame) cv2.imwrite(filename='saved_img.jpg', img=frame) video_capture.release() img_new = cv2.imread('saved_img.jpg', cv2.IMREAD_GRAYSCALE) img_new = cv2.imshow("Captured Image", img_new) cv2.waitKey(1650) print("Image Saved") print("Program End") cv2.destroyAllWindows() break elif cv2.waitKey(1) & 0xFF == ord('q'): print("Turning off camera.") video_capture.release() print("Camera off.") print("Program ended.") cv2.destroyAllWindows() break # Display the resulting frame cv2.imshow('Video', frame) # When everything is done, release the capture video_capture.release() cv2.destroyAllWindows() 1. Please explains in details per line about above coding face recognition with opencv+python? 2. How to make a correct coding with additional recognition lips or eyebrow beside face recognition with opencv+python?
import cv2 import sys import logging as log import datetime as dt from time import sleep cascPath = "haarcascade_frontalface_default.xml" faceCascade = cv2.CascadeClassifier(cascPath) log.basicConfig(filename='webcam.log',level=log.INFO) video_capture = cv2.VideoCapture(0) anterior = 0 while True: if not video_capture.isOpened(): print('Unable to load camera.') sleep(5) pass # Capture frame-by-frame ret, frame = video_capture.read() gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) faces = faceCascade.detectMultiScale( gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30) ) # Draw a rectangle around the faces for (x, y, w, h) in faces: cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2) if anterior != len(faces): anterior = len(faces) log.info("faces: "+str(len(faces))+" at "+str(dt.datetime.now())) # Display the resulting frame cv2.imshow('Video', frame) if cv2.waitKey(1) & 0xFF == ord('s'): check, frame = video_capture.read() cv2.imshow("Capturing", frame) cv2.imwrite(filename='saved_img.jpg', img=frame) video_capture.release() img_new = cv2.imread('saved_img.jpg', cv2.IMREAD_GRAYSCALE) img_new = cv2.imshow("Captured Image", img_new) cv2.waitKey(1650) print("Image Saved") print("Program End") cv2.destroyAllWindows() break elif cv2.waitKey(1) & 0xFF == ord('q'): print("Turning off camera.") video_capture.release() print("Camera off.") print("Program ended.") cv2.destroyAllWindows() break # Display the resulting frame cv2.imshow('Video', frame) # When everything is done, release the capture video_capture.release() cv2.destroyAllWindows() 1. Please explains in details per line about above coding face recognition with opencv+python? 2. How to make a correct coding with additional recognition lips or eyebrow beside face recognition with opencv+python?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
import cv2
import sys
import logging as log
import datetime as dt
from time import sleep
cascPath = "haarcascade_frontalface_default.xml"
faceCascade = cv2.CascadeClassifier(cascPath)
log.basicConfig(filename='webcam.log',level=log.INFO)
video_capture = cv2.VideoCapture(0)
anterior = 0
while True:
if not video_capture.isOpened():
print('Unable to load camera.')
sleep(5)
pass
# Capture frame-by-frame
ret, frame = video_capture.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = faceCascade.detectMultiScale(
gray,
scaleFactor=1.1,
minNeighbors=5,
minSize=(30, 30)
)
# Draw a rectangle around the faces
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
if anterior != len(faces):
anterior = len(faces)
log.info("faces: "+str(len(faces))+" at "+str(dt.datetime.now()))
# Display the resulting frame
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('s'):
check, frame = video_capture.read()
cv2.imshow("Capturing", frame)
cv2.imwrite(filename='saved_img.jpg', img=frame)
video_capture.release()
img_new = cv2.imread('saved_img.jpg', cv2.IMREAD_GRAYSCALE)
img_new = cv2.imshow("Captured Image", img_new)
cv2.waitKey(1650)
print("Image Saved")
print("Program End")
cv2.destroyAllWindows()
break
elif cv2.waitKey(1) & 0xFF == ord('q'):
print("Turning off camera.")
video_capture.release()
print("Camera off.")
print("Program ended.")
cv2.destroyAllWindows()
break
# Display the resulting frame
cv2.imshow('Video', frame)
# When everything is done, release the capture
video_capture.release()
cv2.destroyAllWindows()
import sys
import logging as log
import datetime as dt
from time import sleep
cascPath = "haarcascade_frontalface_default.xml"
faceCascade = cv2.CascadeClassifier(cascPath)
log.basicConfig(filename='webcam.log',level=log.INFO)
video_capture = cv2.VideoCapture(0)
anterior = 0
while True:
if not video_capture.isOpened():
print('Unable to load camera.')
sleep(5)
pass
# Capture frame-by-frame
ret, frame = video_capture.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = faceCascade.detectMultiScale(
gray,
scaleFactor=1.1,
minNeighbors=5,
minSize=(30, 30)
)
# Draw a rectangle around the faces
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
if anterior != len(faces):
anterior = len(faces)
log.info("faces: "+str(len(faces))+" at "+str(dt.datetime.now()))
# Display the resulting frame
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('s'):
check, frame = video_capture.read()
cv2.imshow("Capturing", frame)
cv2.imwrite(filename='saved_img.jpg', img=frame)
video_capture.release()
img_new = cv2.imread('saved_img.jpg', cv2.IMREAD_GRAYSCALE)
img_new = cv2.imshow("Captured Image", img_new)
cv2.waitKey(1650)
print("Image Saved")
print("Program End")
cv2.destroyAllWindows()
break
elif cv2.waitKey(1) & 0xFF == ord('q'):
print("Turning off camera.")
video_capture.release()
print("Camera off.")
print("Program ended.")
cv2.destroyAllWindows()
break
# Display the resulting frame
cv2.imshow('Video', frame)
# When everything is done, release the capture
video_capture.release()
cv2.destroyAllWindows()
1. Please explains in details per line about above coding face recognition with opencv+python?
2. How to make a correct coding with additional recognition lips or eyebrow beside face recognition with opencv+python?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
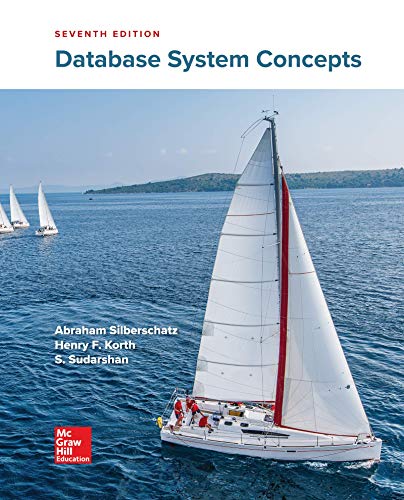
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
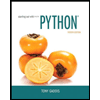
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
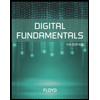
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
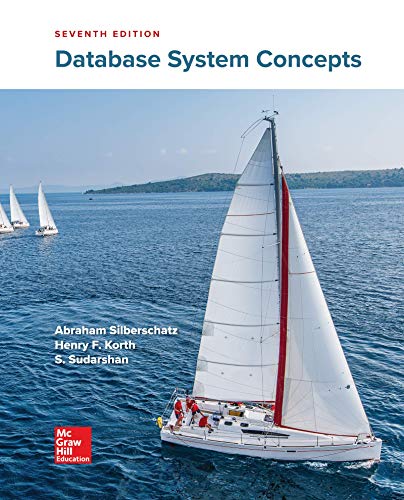
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
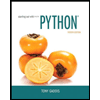
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
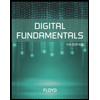
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
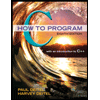
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
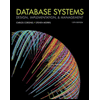
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
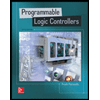
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education