import org.firmata4j.IODevice; import org.firmata4j.Pin; import org.firmata4j.firmata.FirmataDevice; import org.firmata4j.ssd1306.SSD1306; import java.util.Timer; import java.io.IOException; import java.util.HashMap; import java.util.TimerTask; public class task extends TimerTask { private final Pin moisturesensor; private final SSD1306 OLEDscreen; private final Pin Waterpump; private boolean lastMoistureState = false; // Track previous moisture state task(Pin sensor, SSD1306 screen, Pin pump) { this.moisturesensor = sensor; this.OLEDscreen = screen; this.Waterpump = pump; } @Override public void run(){ String valueString = String.valueOf(moisturesensor.getValue()); boolean currentMoistureState = moisturesensor.getValue() > 600; if (currentMoistureState != lastMoistureState) { OLEDscreen.getCanvas().clear(); if (currentMoistureState) { try { Waterpump.setValue(1); } catch (IOException e) { throw new RuntimeException(e); } OLEDscreen.getCanvas().drawString(0, 15, "DRY WATER MORE"); OLEDscreen.getCanvas().drawString(0, 26, "PUMPING WATER"); System.out.println("Soil is dry and needs more water"); } else { try { Waterpump.setValue(0); } catch (IOException e) { throw new RuntimeException(e); } OLEDscreen.getCanvas().drawString(0, 15, "WET, NO MORE NEEDED"); OLEDscreen.getCanvas().drawString(0, 26, "STOP THE PUMPING"); System.out.println("Soil is WET AND NEEDS NO MORE WATER"); } OLEDscreen.display(); lastMoistureState = currentMoistureState; } } }--- import StdDraw.StopButton; import org.firmata4j.IODevice; import org.firmata4j.Pin; import org.firmata4j.firmata.FirmataDevice; import org.firmata4j.ssd1306.SSD1306; import java.util.Timer; import java.io.IOException; import java.util.HashMap; import java.util.TimerTask; public class minorproj { private static Object timerObject; public static void main(String[] args) throws IOException, InterruptedException { String portID = "/dev/cu.usbserial-0001"; IODevice arduinoObject = new FirmataDevice(portID); arduinoObject.start(); arduinoObject.ensureInitializationIsDone(); var i2cObject = arduinoObject.getI2CDevice((byte) 0x3C); SSD1306 OLED = new SSD1306(i2cObject, SSD1306.Size.SSD1306_128_64); OLED.init(); var moistureSensor = arduinoObject.getPin(16); moistureSensor.setMode(Pin.Mode.ANALOG); var buttonPin = arduinoObject.getPin(6); // Button pin on D6 buttonPin.setMode(Pin.Mode.INPUT); var stopButton = new StopButton(buttonPin, timerObject); // Create a StopButton instance var waterPump = arduinoObject.getPin(7); waterPump.setMode(Pin.Mode.OUTPUT); var Task = new task(moistureSensor, OLED, waterPump); Timer timerObject = new Timer(); timerObject.schedule(Task, 0, 1000); } for this entire code, just ADD a part where pressing the button will END THE ENTRE CODE. the button pin is D6.
import org.firmata4j.IODevice; import org.firmata4j.Pin; import org.firmata4j.firmata.FirmataDevice; import org.firmata4j.ssd1306.SSD1306; import java.util.Timer; import java.io.IOException; import java.util.HashMap; import java.util.TimerTask; public class task extends TimerTask { private final Pin moisturesensor; private final SSD1306 OLEDscreen; private final Pin Waterpump; private boolean lastMoistureState = false; // Track previous moisture state task(Pin sensor, SSD1306 screen, Pin pump) { this.moisturesensor = sensor; this.OLEDscreen = screen; this.Waterpump = pump; } @Override public void run(){ String valueString = String.valueOf(moisturesensor.getValue()); boolean currentMoistureState = moisturesensor.getValue() > 600; if (currentMoistureState != lastMoistureState) { OLEDscreen.getCanvas().clear(); if (currentMoistureState) { try { Waterpump.setValue(1); } catch (IOException e) { throw new RuntimeException(e); } OLEDscreen.getCanvas().drawString(0, 15, "DRY WATER MORE"); OLEDscreen.getCanvas().drawString(0, 26, "PUMPING WATER"); System.out.println("Soil is dry and needs more water"); } else { try { Waterpump.setValue(0); } catch (IOException e) { throw new RuntimeException(e); } OLEDscreen.getCanvas().drawString(0, 15, "WET, NO MORE NEEDED"); OLEDscreen.getCanvas().drawString(0, 26, "STOP THE PUMPING"); System.out.println("Soil is WET AND NEEDS NO MORE WATER"); } OLEDscreen.display(); lastMoistureState = currentMoistureState; } } }--- import StdDraw.StopButton; import org.firmata4j.IODevice; import org.firmata4j.Pin; import org.firmata4j.firmata.FirmataDevice; import org.firmata4j.ssd1306.SSD1306; import java.util.Timer; import java.io.IOException; import java.util.HashMap; import java.util.TimerTask; public class minorproj { private static Object timerObject; public static void main(String[] args) throws IOException, InterruptedException { String portID = "/dev/cu.usbserial-0001"; IODevice arduinoObject = new FirmataDevice(portID); arduinoObject.start(); arduinoObject.ensureInitializationIsDone(); var i2cObject = arduinoObject.getI2CDevice((byte) 0x3C); SSD1306 OLED = new SSD1306(i2cObject, SSD1306.Size.SSD1306_128_64); OLED.init(); var moistureSensor = arduinoObject.getPin(16); moistureSensor.setMode(Pin.Mode.ANALOG); var buttonPin = arduinoObject.getPin(6); // Button pin on D6 buttonPin.setMode(Pin.Mode.INPUT); var stopButton = new StopButton(buttonPin, timerObject); // Create a StopButton instance var waterPump = arduinoObject.getPin(7); waterPump.setMode(Pin.Mode.OUTPUT); var Task = new task(moistureSensor, OLED, waterPump); Timer timerObject = new Timer(); timerObject.schedule(Task, 0, 1000); } for this entire code, just ADD a part where pressing the button will END THE ENTRE CODE. the button pin is D6.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
import org.firmata4j.IODevice;
import org.firmata4j.Pin;
import org.firmata4j.firmata.FirmataDevice;
import org.firmata4j.ssd1306.SSD1306;
import java.util.Timer;
import java.io.IOException;
import java.util.HashMap;
import java.util.TimerTask;
public class task extends TimerTask {
private final Pin moisturesensor;
private final SSD1306 OLEDscreen;
private final Pin Waterpump;
private boolean lastMoistureState = false; // Track previous moisture state
task(Pin sensor, SSD1306 screen, Pin pump) {
this.moisturesensor = sensor;
this.OLEDscreen = screen;
this.Waterpump = pump;
}
@Override
public void run(){
String valueString = String.valueOf(moisturesensor.getValue());
boolean currentMoistureState = moisturesensor.getValue() > 600;
if (currentMoistureState != lastMoistureState) {
OLEDscreen.getCanvas().clear();
if (currentMoistureState) {
try {
Waterpump.setValue(1);
} catch (IOException e) {
throw new RuntimeException(e);
}
OLEDscreen.getCanvas().drawString(0, 15, "DRY WATER MORE");
OLEDscreen.getCanvas().drawString(0, 26, "PUMPING WATER");
System.out.println("Soil is dry and needs more water");
} else {
try {
Waterpump.setValue(0);
} catch (IOException e) {
throw new RuntimeException(e);
}
OLEDscreen.getCanvas().drawString(0, 15, "WET, NO MORE NEEDED");
OLEDscreen.getCanvas().drawString(0, 26, "STOP THE PUMPING");
System.out.println("Soil is WET AND NEEDS NO MORE WATER");
}
OLEDscreen.display();
lastMoistureState = currentMoistureState;
}
}
}---
import StdDraw.StopButton;
import org.firmata4j.IODevice;
import org.firmata4j.Pin;
import org.firmata4j.firmata.FirmataDevice;
import org.firmata4j.ssd1306.SSD1306;
import java.util.Timer;
import java.io.IOException;
import java.util.HashMap;
import java.util.TimerTask;
public class minorproj {
private static Object timerObject;
public static void main(String[] args) throws IOException, InterruptedException {
String portID = "/dev/cu.usbserial-0001";
IODevice arduinoObject = new FirmataDevice(portID);
arduinoObject.start();
arduinoObject.ensureInitializationIsDone();
var i2cObject = arduinoObject.getI2CDevice((byte) 0x3C);
SSD1306 OLED = new SSD1306(i2cObject, SSD1306.Size.SSD1306_128_64);
OLED.init();
var moistureSensor = arduinoObject.getPin(16);
moistureSensor.setMode(Pin.Mode.ANALOG);
var buttonPin = arduinoObject.getPin(6); // Button pin on D6
buttonPin.setMode(Pin.Mode.INPUT);
var stopButton = new StopButton(buttonPin, timerObject); // Create a StopButton instance
var waterPump = arduinoObject.getPin(7);
waterPump.setMode(Pin.Mode.OUTPUT);
var Task = new task(moistureSensor, OLED, waterPump);
Timer timerObject = new Timer();
timerObject.schedule(Task, 0, 1000);
}
for this entire code, just ADD a part where pressing the button will END THE ENTRE CODE. the button pin is D6.
import org.firmata4j.IODevice;
import org.firmata4j.Pin;
import org.firmata4j.firmata.FirmataDevice;
import org.firmata4j.ssd1306.SSD1306;
import java.util.Timer;
import java.io.IOException;
import java.util.HashMap;
import java.util.TimerTask;
public class minorproj {
private static Object timerObject;
public static void main(String[] args) throws IOException, InterruptedException {
String portID = "/dev/cu.usbserial-0001";
IODevice arduinoObject = new FirmataDevice(portID);
arduinoObject.start();
arduinoObject.ensureInitializationIsDone();
var i2cObject = arduinoObject.getI2CDevice((byte) 0x3C);
SSD1306 OLED = new SSD1306(i2cObject, SSD1306.Size.SSD1306_128_64);
OLED.init();
var moistureSensor = arduinoObject.getPin(16);
moistureSensor.setMode(Pin.Mode.ANALOG);
var buttonPin = arduinoObject.getPin(6); // Button pin on D6
buttonPin.setMode(Pin.Mode.INPUT);
var stopButton = new StopButton(buttonPin, timerObject); // Create a StopButton instance
var waterPump = arduinoObject.getPin(7);
waterPump.setMode(Pin.Mode.OUTPUT);
var Task = new task(moistureSensor, OLED, waterPump);
Timer timerObject = new Timer();
timerObject.schedule(Task, 0, 1000);
}
for this entire code, just ADD a part where pressing the button will END THE ENTRE CODE. the button pin is D6.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
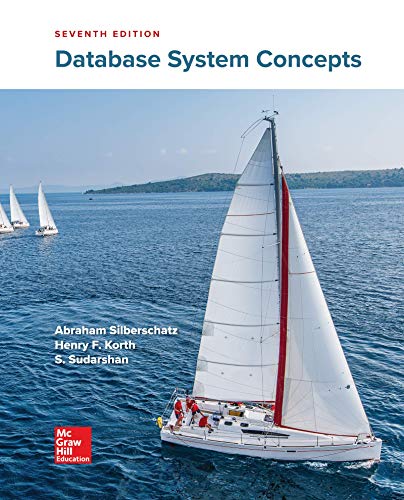
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
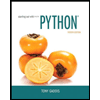
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
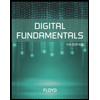
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
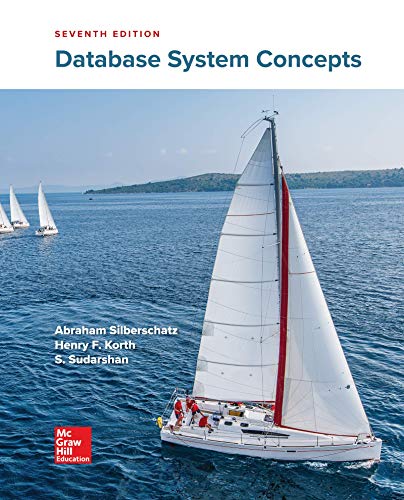
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
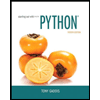
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
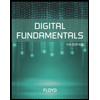
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
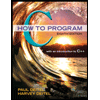
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
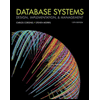
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
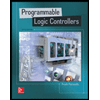
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education