In an ancient land, the beautiful princess Eve had many suitors. She decided on the following procedure to determine which suitor she would marry. First, all of the suitors would be lined up one after the other and assigned numbers. The first suitor would be number 1, the second number 2, and so on up to the last suitor, number n. Starting at the first suitor, she would then count three suitors down the line (because of the three letters in her name) and the third suitor would be eliminated from winning her hand and removed from the line. Eve would then continue, counting three more suitors, and eliminating every third suitor. When she reached the end of the line, she would continue counting from the beginning. For example, if there were six suitors, then the elimination process would proceed as follows: 123456 Initial list of suitors, start counting from 1 12456 Suitor 3 eliminated, continue counting from 4 1245 Suitor 6 eliminated, continue counting from 1 125 Suitor 4 eliminated, continue counting from 5 15 Suitor 2 eliminated, continue counting from 5 1 Suitor 5 eliminated, 1 is the lucky winner Write a program that creates a circular linked list of nodes to determine which position you should stand in to marry the princess if there are n suitors. Your program should simulate the elimination process by deleting the node that corresponds to the suitor that is eliminated for each step in the process. Be careful that you do not leave any memory leaks. *Previous we solved this question using vector, now use the linkedList instead of vector* Following is the program using vector for your reference. #include #include #include using namespace std; int main(){ int n; cout<<"Enter the number of suitor: "; cin>>n; vector v; for(int i=1;i<=n;i++) v.push_back(i); int index = 0; while(v.size()>1){ index = index + 2; if(index >= v.size()) index = index % v.size(); v.erase(v.begin()+index); for(int i=0;i4 6->1 10->4 */
C++ Question please help
In an ancient land, the beautiful princess Eve had many suitors. She decided on the
following procedure to determine which suitor she would marry. First, all of the suitors
would be lined up one after the other and assigned numbers. The first suitor would be
number 1, the second number 2, and so on up to the last suitor, number n. Starting at
the first suitor, she would then count three suitors down the line (because of the three
letters in her name) and the third suitor would be eliminated from winning her hand and
removed from the line. Eve would then continue, counting three more suitors, and
eliminating every third suitor. When she reached the end of the line, she would continue
counting from the beginning. For example, if there were six suitors, then the elimination
process would proceed as follows:
123456 Initial list of suitors, start counting from 1
12456 Suitor 3 eliminated, continue counting from 4
1245 Suitor 6 eliminated, continue counting from 1
125 Suitor 4 eliminated, continue counting from 5
15 Suitor 2 eliminated, continue counting from 5
1 Suitor 5 eliminated, 1 is the lucky winner
Write a program that creates a circular linked list of nodes to determine which position
you should stand in to marry the princess if there are n suitors. Your program should
simulate the elimination process by deleting the node that corresponds to the suitor that
is eliminated for each step in the process. Be careful that you do not leave any memory
leaks.
*Previous we solved this question using
vector*
Following is the program using vector for your reference.
#include <iostream>
#include <string>
#include <vector>
using namespace std;
int main(){
int n;
cout<<"Enter the number of suitor: ";
cin>>n;
vector<int> v;
for(int i=1;i<=n;i++)
v.push_back(i);
int index = 0;
while(v.size()>1){
index = index + 2;
if(index >= v.size()) index = index % v.size();
v.erase(v.begin()+index);
for(int i=0;i<v.size();i++)
cout<<v[i]<<" ";
cout<<endl;
}
cout<<"the final one is "<<v[0]<<endl;
}
/*
5->4
6->1
10->4
*/

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

There's a lot of errors
![J
main.cpp:9:20: error: expected unqualified-id
list<int>1;
main.cpp:23:14: error: assigning to 'int' from incompatible type 'int (const char *) noexcept
(true)'
i= remove;
Annenenene
main.cpp:25:36: error: invalid suffix 'begin' on floating constant
list<int>::iterator it-1.begin();
main.cpp:27:19: error: invalid suffix 'size' on floating constant
while (1.size() >1) {
main.cpp:31:45: error: expected ';' at end of declaration
list<int>::iterator it2=it++
main.cpp:35:19: error: assigning to 'int' from incompatible type 'int (const char *) noexcept
(true)'
i=remove;
main.cpp:41:21: error: exponent has no digits
if (it==1.end()) it-1.begin();
main.cpp:41:33: error: invalid suffix 'begin' on floating constant
if (it==1.end()) it-1.begin();
main.cpp:45:23: error: invalid suffix 'front' on floating constant
cout<<"winner:"<<1.front() +1 end1;
9 errors generated.
make: *** [Makefile:17: main.o] Error 1
exit status 2](https://content.bartleby.com/qna-images/question/913a0921-d398-48e9-845b-58c3dce12c9b/00c29de5-3162-46de-a762-20a30d1e8bc1/jg47ju_thumbnail.png)
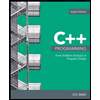
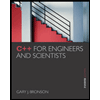
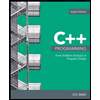
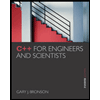