In c++ Create a new project named lab9_2. You will continue to use the Courses class, but this time you will create a vector of Courses. The file you will read from is below: 6 CSS 2A 1111 35 CSS 2A 2222 20 CSS 1 3333 40 CSS 1 4444 33 CSS 3 5555 15 CSS 44 6666 12 Read this information into a vector of Courses. Then, print out a summary of your vector. Here's a sample driver: #include #include #include #include #include #include "Course.h" using namespace std; int main() { vector myclass; string dep, c_num; int classes, sec, num_stus; ifstream fin("sample.txt"); if (fin.fail()) { cout << "File path is bad\n"; exit(1); } fin >> classes; for (int i = 0; i < classes; i++) { fin >> dep >> c_num >> sec >> num_stus; // Now how do you create a Course object // that contains the information you just read in // and add it to your myclass vector? } cout << "Here are the college courses: " << endl; for (Course& c : myclass) { c.print(); } return 0; } Sample run: Here are the college courses: ----------------------------- Course: CSS 2A, Section: 1111 Enrolled: 35, Status: Open ----------------------------- Course: CSS 2A, Section: 2222 Enrolled: 20, Status: Open ----------------------------- Course: CSS 1, Section: 3333 Enrolled: 40, Status: Closed ----------------------------- Course: CSS 1, Section: 4444 Enrolled: 33, Status: Open ----------------------------- Course: CSS 3, Section: 5555 Enrolled: 15, Status: Open ----------------------------- Course: CSS 44, Section: 6666 Enrolled: 12, Status: Open
In c++
Create a new project named lab9_2. You will continue to use the Courses class, but this time you will create a
6
CSS 2A 1111 35
CSS 2A 2222 20
CSS 1 3333 40
CSS 1 4444 33
CSS 3 5555 15
CSS 44 6666 12
Read this information into a vector of Courses. Then, print out a summary of your vector.
Here's a sample driver:
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <cstdlib>
#include "Course.h"
using namespace std;
int main()
{
vector<Course> myclass;
string dep, c_num;
int classes, sec, num_stus;
ifstream fin("sample.txt");
if (fin.fail())
{
cout << "File path is bad\n";
exit(1);
}
fin >> classes;
for (int i = 0; i < classes; i++)
{
fin >> dep >> c_num >> sec >> num_stus;
// Now how do you create a Course object
// that contains the information you just read in
// and add it to your myclass vector?
}
cout << "Here are the college courses: " << endl;
for (Course& c : myclass)
{
c.print();
}
return 0;
}
Sample run:
Here are the college courses:
-----------------------------
Course: CSS 2A, Section: 1111
Enrolled: 35, Status: Open
-----------------------------
Course: CSS 2A, Section: 2222
Enrolled: 20, Status: Open
-----------------------------
Course: CSS 1, Section: 3333
Enrolled: 40, Status: Closed
-----------------------------
Course: CSS 1, Section: 4444
Enrolled: 33, Status: Open
-----------------------------
Course: CSS 3, Section: 5555
Enrolled: 15, Status: Open
-----------------------------
Course: CSS 44, Section: 6666
Enrolled: 12, Status: Open
-----------------------------

Step by step
Solved in 7 steps with 7 images

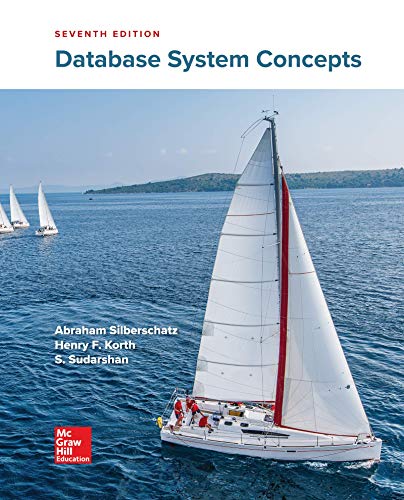
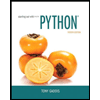
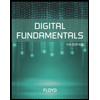
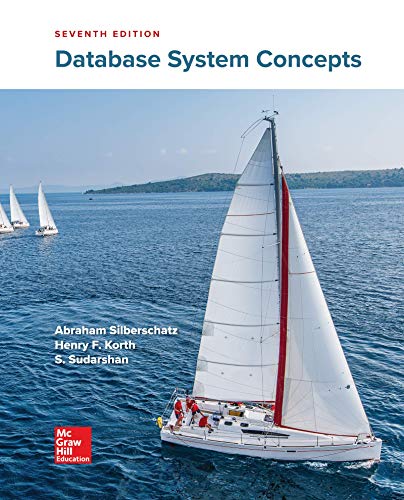
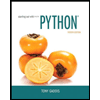
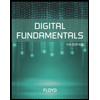
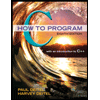
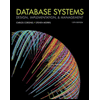
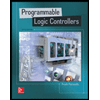