In c++ please help me answer this question I will give you a good rating :) implement the three versions of the addupto20() function: iterative, recursive, and divide-and-conquer approach test these functions with a few inputs from your main() Iterative solution * check if there exsits two numbers from vector data that add up to 20 e.g., if data=[2,5,3,15], the function returns true, as data [0] +data [3]==20 e.g., if data=[3,4,0,8], the function return false precondition: vector data has been initialized postcondition: if there are two numbers from list add up to 20, return true; otherwise, return false */ bool AddupTo20 (const vector‹int> & data){ } Come up with a recursive solution to the problem, following the hints given below: * check if there exsits two numbers from vector data[first...right] add up to 20 e.g., if data=[2,5,3,15], first=0, last=3, the function returns true, as data [0] +data [3]==20 e.g., if data=[2,5,3,15], first=2, last=3, the function returns fales (there are no pairs from data[2..3] that add up to 20 precondition: vector data has been initialized first, last are indicating the starting and ending indices postcondition: if there are two numbers from data[first. ..last] add up to 20, return true; otherwise, return false */ bool AddupTo20 (const vector‹int› & data, int first, int last) { // BASE CASE: if data[first... last]'s length is 1 // return false //General case // check if adding a[first] with a number from data[first+1...last] // yields a sum of 20 // check if any pairs from data[first+1 ... last] add up to 20 }
In c++ please help me answer this question I will give you a good rating :)
-
implement the three versions of the addupto20() function: iterative, recursive, and divide-and-conquer approach
-
test these functions with a few inputs from your main()
Iterative solution
* check if there exsits two numbers from
e.g., if data=[2,5,3,15], the function returns true, as data [0] +data [3]==20
e.g., if data=[3,4,0,8], the function return false
precondition: vector data has been initialized
postcondition: if there are two numbers from list add up to 20, return true;
otherwise, return false */
bool AddupTo20 (const vector‹int> & data){
}
Come up with a recursive solution to the problem, following the hints given below:
* check if there exsits two numbers from vector data[first...right] add up to 20
e.g., if data=[2,5,3,15], first=0, last=3, the function returns true,
as data [0] +data [3]==20
e.g., if data=[2,5,3,15], first=2, last=3, the function returns fales
(there are no pairs from data[2..3] that add up to 20
precondition: vector data has been initialized
first, last are indicating the starting and ending indices
postcondition: if there are two numbers from data[first.
..last] add up to 20,
return true; otherwise, return false */
bool AddupTo20 (const vector‹int› & data, int first, int last)
{
// BASE CASE: if data[first... last]'s length is 1
// return false
//General case
// check if adding a[first] with a number from data[first+1...last]
// yields a sum of 20
// check if any pairs from data[first+1 ... last] add up to 20
}

Step by step
Solved in 6 steps with 2 images

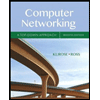
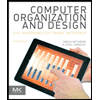
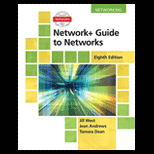
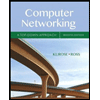
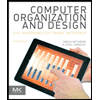
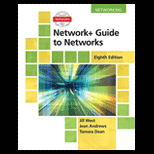
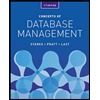
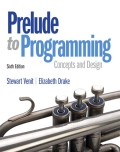
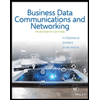