In C programming Language You have been tasked to calculate the amortization of a mortgage loan. This will make use of function calls and repetition. A loan officer meeting with a loan customer will use the program by entering in the required values: Duration of loan in years, principal loan amount, and the annual interest rate of the loan. Your program will compute the monthly payment and use that calculation to produce an amortization chart showing each month (period), the amount of principal applied, the amount of interest applied, the remaining balance and the total monthly payment. After the amortization information has displayed, the program will print out the total interest paid on the loan, the total principal paid and the grand total of all the money spent on the loan. Objectives: Use of functions with pointers as well as pass by value. Understand how to overcome the limitations of function return values Use of repetition constructs. Display formatted output using input/output statements. Use format control strings to format text output. Requirements: The get input functions must use pointers and not use return values except for error checking options. A function to prompt the user and get the needed input and return it to the caller. This function should check to make sure that the input is within the specified limits and provide prompting if it is not. The user should also be able to exit the application by choice. The main function should actually terminate the program not the called function. NOTE: you can break this into several functions called from main or several functions called from inside this function. Doing so can greatly decompose and simplify your task and source code. If you construct these correctly you can copy and paste much of the code for each function. Example: getinterest(), getloanamount(), getloanterm(). 3. A function to calculate the required monthly payment from the provided information. This function should return the monthly payment to the caller. This is a computed value needed of the amortization function. The formula and example is given below. A function to compute and print out the amortization as a table. Problem: How can this function keep track of the total interest paid and the total principal paid on this loan? 4. The amortization function should produce the table such as the example shown. You should make use of the minimum width specifier and the precision should be no more than 2 places to the right of the decimal. This will ensure your output is aligned. You need width to accommodate 2000000.00 this is 10 characters in width. 5. You must use defined constants to test the limits of the input values. Term must be at least 4 years and no more than 30. Amount must be at least 10000 and no more than 2000000. Interest rate must be at least 1% and no more than 20%. Name them correctly. Basic algorithm: Get the needed valid input. Compute the monthly payment. Create the amortization table/chart by repetitively computing and subtracting the monthly finance charge from the payment and applying the rest to the principal reduction. Keep track of the total interest payments over the life of the loan to report at the end. Report the statistics (totals).
In C programming Language
You have been tasked to calculate the amortization of a mortgage loan. This will make use of function calls and repetition. A loan officer meeting with a loan customer will use the program by entering in the required values: Duration of loan in years, principal loan amount, and the annual interest rate of the loan. Your program will compute the monthly payment and use that calculation to produce an amortization chart showing each month (period), the amount of principal applied, the amount of interest applied, the remaining balance and the total monthly payment. After the amortization information has displayed, the program will print out the total interest paid on the loan, the total principal paid and the grand total of all the money spent on the loan.
Objectives:
- Use of functions with pointers as well as pass by value.
- Understand how to overcome the limitations of function return values
- Use of repetition constructs.
- Display formatted output using input/output statements.
- Use format control strings to format text output.
Requirements:
- The get input functions must use pointers and not use return values except for error checking options.
- A function to prompt the user and get the needed input and return it to the caller. This function should check to make sure that the input is within the specified limits and provide prompting if it is not. The user should also be able to exit the application by choice. The main function should actually terminate the program not the called function.
NOTE: you can break this into several functions called from main or several functions called from inside this function. Doing so can greatly decompose and simplify your task and source code. If you construct these correctly you can copy and paste much of the code for each function. Example: getinterest(), getloanamount(), getloanterm().
3. A function to calculate the required monthly payment from the provided information. This function should return the monthly payment to the caller. This is a computed value needed of the amortization function. The formula and example is given below. A function to compute and print out the amortization as a table. Problem: How can this function keep track of the total interest paid and the total principal paid on this loan?
4. The amortization function should produce the table such as the example shown. You should make use of the minimum width specifier and the precision should be no more than 2 places to the right of the decimal. This will ensure your output is aligned. You need width to accommodate 2000000.00 this is 10 characters in width.
5. You must use defined constants to test the limits of the input values. Term must be at least 4 years and no more than 30. Amount must be at least 10000 and no more than 2000000. Interest rate must be at least 1% and no more than 20%. Name them correctly.
Basic
- Get the needed valid input.
- Compute the monthly payment.
- Create the amortization table/chart by repetitively computing and subtracting the monthly finance charge from the payment and applying the rest to the principal reduction.
- Keep track of the total interest payments over the life of the loan to report at the end.
- Report the statistics (totals).
![How Do You Calculate Loan Payments?
Amortized Loan Payment Formula
Calculate your monthly payment (p) using your principal balance or total loan amount
(a). periodic interest rate (r). which is your annual rate divided by the number of
payment periods. and your total number of payment periods (n):131
a/{[(1+r)^n]-1}/[r(1+r)^n]=p
Assume you borrow $100.000 at 6% for 30 years to be repaid monthly. To calculate
the monthly payment, convert percentages to decimal format, then follow the
formula:
a: 100,000, the amount of the loan
r.0.005 (6% annual rate- expressed as 0.06-divided by 12 monthly payments
per year)
* n: 360 (12 monthly payments per year times 30 years)
Calculation: 100,000/((1+0.005) 360)-1)/[0.005(110.005) 560)-599,55. or
100.000/166.7916-599.55
The monthly payment is S599.55, Check your math with an online payment
calculator,
*You can use this to example data ensure your function is working. Hint: Don't feed pow()
an expression, compute the base and then provide it to pow().](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbc29e3d0-ab06-4b11-9f3c-9c089e985983%2F21f26dc3-b857-4a0c-a60e-6b65e6a1ec94%2Ftlklql_processed.jpeg&w=3840&q=75)
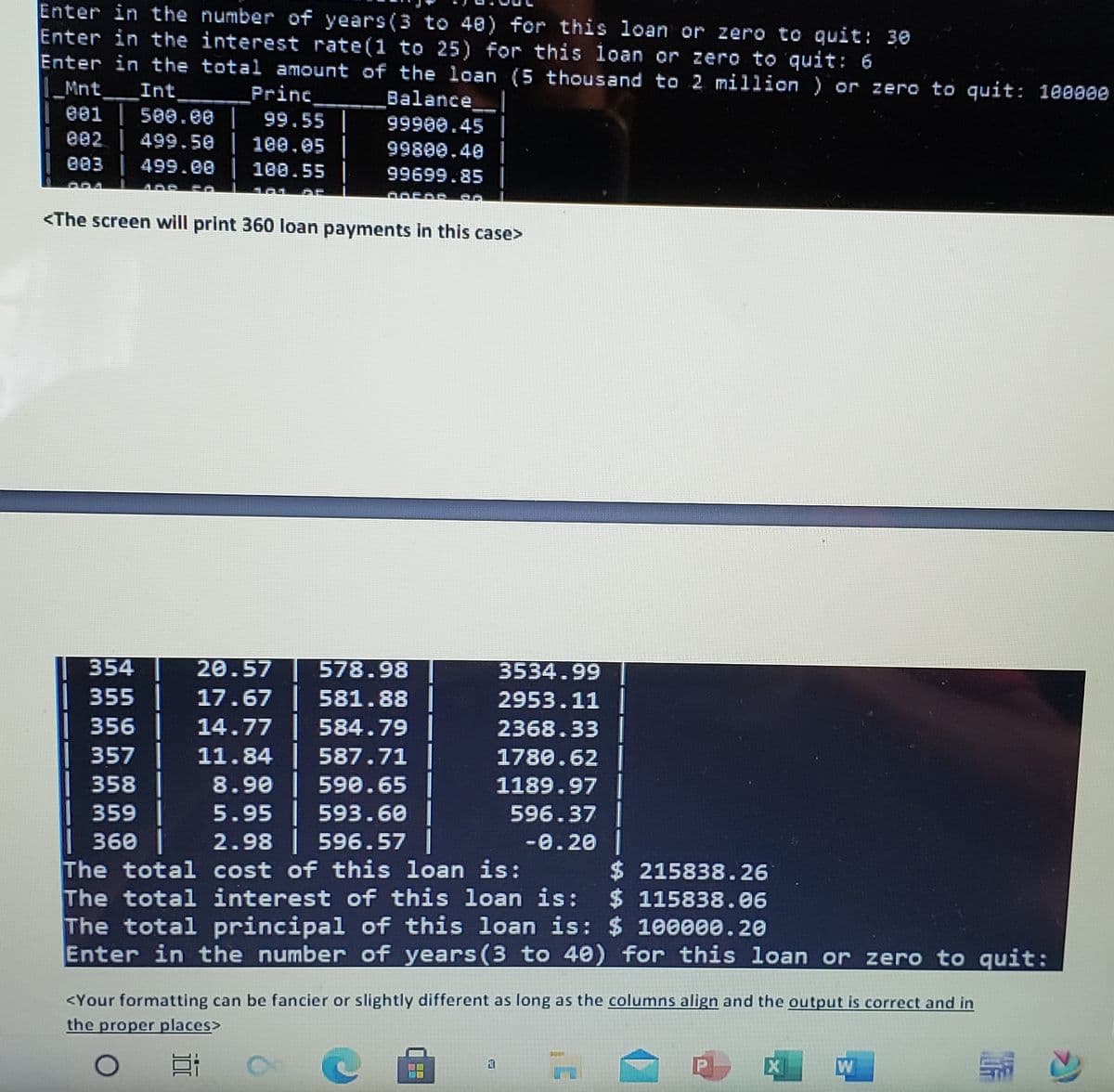

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

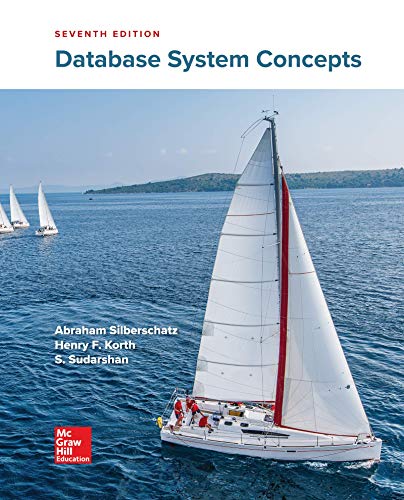
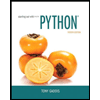
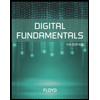
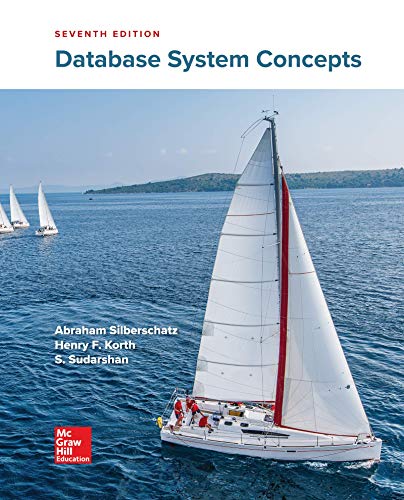
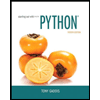
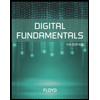
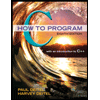
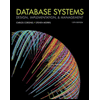
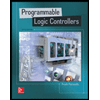