In Java Every computer on the Internet has a unique identifying number, called an Internet protocol (IP) address. To contact a computer on the Internet, you send a message to the computer’s IP address. Here are some typical IP addresses: 216.27.6.136 224.0.118.62 There are different formats for displaying IP addresses, but the most common format is the dotted decimal format. The above two IP addresses use the dotteddecimal format. It’s called “dotted” because dots are used to split up the big IP address number into four smaller numbers. It’s called “decimal” because decimal numbers are used (as opposed to binary) for the four smaller numbers. Each of the four smaller numbers is called an octet because each number represents eight bits (oct means eight). For example, the 216 octet represents 11011000 and the 27 octet represents 00011011. Implement an IpAddress class that stores an IP address as a dotted-decimal string and as four octet ints. You must implement all of the following: Instance variables: 1-dottedDecimal – a dotted-decimal string. Example value: "216.27.6.136" 2-firstOctet, secondOctet, thirdOctet, fourthOctet – four int variables that store the octets for an IP address Constructor: This constructor receives one parameter, a dotted-decimal string. You may assume that the parameter’s value is valid (i.e., no error checking required). The constructor initializes the instance variables with appropriate values. There are many ways to solve the problem of extracting octets from the given dotted-decimal string. We recommend that you use String methods to extract the individual octets as strings, and then use parseInt method calls to convert the octet strings to ints. getDottedDecimal method: This is a standard accessor method that simply returns the dottedDecimal instance variable’s value. getOctet method: This method receives the position of one of the octets (1, 2, 3, or 4) and returns the octet that’s at that position. Provide a driver class that tests your IpAddress class. Your driver class should contain this main method: public static void main(String[] args) { IpAddress ip = new IpAddress("216.27.6.136"); System.out.println(ip.getDottedDecimal()); System.out.println(ip.getOctet(4)); System.out.println(ip.getOctet(1)); System.out.println(ip.getOctet(3)); System.out.println(ip.getOctet(2)); } // end main Using the above main method, your program should generate the following output. Sample output: 216.27.6.136 136 216 6 27 IPAddress Class: public class IpAddress { // IP address using dotted-decimal format // IP address's four octets //*********************************************************** // This constructor initializes the instance variables with the given dottedDecimal string. public IpAddress(String dottedDecimal) { } // end constructor //*********************************************************** public String getDottedDecimal() { } // end getOctet //*********************************************************** // This method returns the octet at the given position. public int getOctet(int octetPosition) { } // end getOctet } // end class IpAddress
In Java
Every computer on the Internet has a unique identifying number, called an Internet
protocol (IP) address. To contact a computer on the Internet, you send a message to
the computer’s IP address. Here are some typical IP addresses:
216.27.6.136
224.0.118.62
There are different formats for displaying IP addresses, but the most common
format is the dotted decimal format. The above two IP addresses use the dotteddecimal format. It’s called “dotted” because dots are used to split up the big IP
address number into four smaller numbers. It’s called “decimal” because decimal
numbers are used (as opposed to binary) for the four smaller numbers.
Each of the four smaller numbers is called an octet because each number represents
eight bits (oct means eight). For example, the 216 octet represents 11011000 and
the 27 octet represents 00011011.
Implement an IpAddress class that stores an IP address as a dotted-decimal string
and as four octet ints. You must implement all of the following:
Instance variables:
1-dottedDecimal – a dotted-decimal string. Example value: "216.27.6.136"
2-firstOctet, secondOctet, thirdOctet, fourthOctet – four int variables that
store the octets for an IP address
Constructor:
This constructor receives one parameter, a dotted-decimal string. You may assume
that the parameter’s value is valid (i.e., no error checking required). The constructor
initializes the instance variables with appropriate values. There are many ways to
solve the problem of extracting octets from the given dotted-decimal string. We
recommend that you use String methods to extract the individual octets as strings,
and then use parseInt method calls to convert the octet strings to ints.
getDottedDecimal method:
This is a standard accessor method that simply returns the dottedDecimal instance
variable’s value.
getOctet method:
This method receives the position of one of the octets (1, 2, 3, or 4) and returns
the octet that’s at that position.
Provide a driver class that tests your IpAddress class. Your driver class should
contain this main method:
public static void main(String[] args)
{
IpAddress ip = new IpAddress("216.27.6.136");
System.out.println(ip.getDottedDecimal());
System.out.println(ip.getOctet(4));
System.out.println(ip.getOctet(1));
System.out.println(ip.getOctet(3));
System.out.println(ip.getOctet(2));
} // end main
Using the above main method, your program should generate the following output.
Sample output:
216.27.6.136
136
216
6
27
IPAddress Class:
public class IpAddress
{
// IP address using dotted-decimal format
// IP address's four octets
//***********************************************************
// This constructor initializes the instance variables with the given dottedDecimal
string.
public IpAddress(String dottedDecimal)
{
} // end constructor
//***********************************************************
public String getDottedDecimal()
{
} // end getOctet
//***********************************************************
// This method returns the octet at the given position.
public int getOctet(int octetPosition)
{
} // end getOctet
} // end class IpAddress

Step by step
Solved in 3 steps

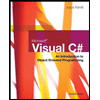
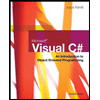